Use C++ In part 1 you will be creating a quiz grading program. You will compare the student's answers with the correct answers, and determine passed or not. The program will make use of two parallel arrays. Each array must be able to support up to 30 characters (so you have two arrays of char or unsigned char values, each one with 30 elements). Your program will need to read in the student answer input file name from cin. It will also need to read in the correct answer file name from cin. You should use the >> operator to read from cin and not get or getline. Using get or getline will be more difficult and will require that you filter out white-space characters in your program. The contents of the student input file should be read into one char array. The file will contain up to 30 characters, each character on a separate line of the file. The first line in the input file will contain the students answer to the first question, and so on for up to 30 questions. The answers are A, B, C or D . The answer file will be read in as well, one answer per line for up to 30 answers. The first line of text is the answer for question 1, then second line is the answer for question 2, and so on. You need to keep track of the number of student answers and the correct answers. If the number of student answers and correct answers is not the same you need to output an error message. close the input files when you are done with them. program needs to determine the number of questions that the student missed and then display the following: A list of questions missed by the student, showing the question number (1 through up to 30), the correct answer, and the incorrect answer. The total number of questions missed by the student The percentage of the questions answered correctly. If the percentage of questions answered correctly is 70% or more indicate that the student passed, otherwise state that the student failed the quiz. You need to have at least the following three functions, including main. Read function One function needs to be passed a char or unsigned char array of size 30, it also needs to be passed an input file name. The return from the function should be an int value. If the number of input values is > 30 return 30. Otherwise return the number of values read in. If the file does not exist return -1. Work/Display function The work/display function will be passed the two arrays, the number of student answers and correct answers read in, and should calculate and display the results from the program. The function needs to do the following: A list of questions missed by the student, showing the question number (1 through up to 30), the correct answer, and the incorrect answer. The total number missed questions answered in percentage. If questions answered correctly is >= 70% indicate passed, otherwise state failed. The main function The main will prompt for the student answers file name and call the read function. If the read function fails the program should output an error message and quit. If the first read works the main should prompt for an read in the correct answers file name and call the read function a second time. If the read fails an error message should be displayed and main should quit. If the number of student answers is not the same as the number of correct answers an error message should be displayed and main should quit. If the number of student answers and correct answers are the same but are both 0 display an error message and quit. If your program gets this far call the work/display function. As always you cannot use any global variables in your program. See the sample runs for examples of the prompts, error messages, and results output. See the sample runs for the output requirements. Sample run 1 (valid data) Contents of cin: student.txt answers.txt Contents of student.txt: A B C D A B C D A B C D A B C D A B C D Contents of answers.txt: A A A A B B B B C C C C D D D D A B C D Here is the output to cout: Enter student answers file name Enter correct answer file name Question 2 has incorrect answer 'B', the correct answer is 'A' Question 3 has incorrect answer 'C', the correct answer is 'A' Question 4 has incorrect answer 'D', the correct answer is 'A' Question 5 has incorrect answer 'A', the correct answer is 'B' Question 7 has incorrect answer 'C', the correct answer is 'B' Question 8 has incorrect answer 'D', the correct answer is 'B' Question 9 has incorrect answer 'A', the correct answer is 'C' Question 10 has incorrect answer 'B', the correct answer is 'C' Question 12 has incorrect answer 'D', the correct answer is 'C' Question 13 has incorrect answer 'A', the correct answer is 'D' Question 14 has incorrect answer 'B', the correct answer is 'D' Question 15 has incorrect answer 'C', the correct answer is 'D' 12 questions were missed out of 20 The student grade is 40.0%. The student failed Sample run 2 (invalid student file) Contents of cin: invalidfile.txt answers.txt Here is the output to cout: Enter student answers file name File "invalidfile.txt" could not be opened
Use C++
In part 1 you will be creating a quiz grading
The program will make use of two parallel arrays.
Each array must be able to support up to 30 characters (so you have two arrays of char or unsigned char values, each one with 30 elements).
Your program will need to read in the student answer input file name from cin. It will also need to read in the correct answer file name from cin. You should use the >> operator to read from cin and not get or getline. Using get or getline will be more difficult and will require that you filter out white-space characters in your program.
The contents of the student input file should be read into one char array. The file will contain up to 30 characters, each character on a separate line of the file. The first line in the input file will contain the students answer to the first question, and so on for up to 30 questions. The answers are A, B, C or D .
The answer file will be read in as well, one answer per line for up to 30 answers. The first line of text is the answer for question 1, then second line is the answer for question 2, and so on.
You need to keep track of the number of student answers and the correct answers. If the number of student answers and correct answers is not the same you need to output an error message.
close the input files when you are done with them.
program needs to determine the number of questions that the student missed and then display the following:
- A list of questions missed by the student, showing the question number (1 through up to 30), the correct answer, and the incorrect answer.
- The total number of questions missed by the student
- The percentage of the questions answered correctly.
- If the percentage of questions answered correctly is 70% or more indicate that the student passed, otherwise state that the student failed the quiz.
You need to have at least the following three functions, including main.
Read function
One function needs to be passed a char or unsigned char array of size 30, it also needs to be passed an input file name. The return from the function should be an int value.
If the number of input values is > 30 return 30. Otherwise return the number of values read in. If the file does not exist return -1.
Work/Display function
The work/display function will be passed the two arrays, the number of student answers and correct answers read in, and should calculate and display the results from the program.
The function needs to do the following:
- A list of questions missed by the student, showing the question number (1 through up to 30), the correct answer, and the incorrect answer.
- The total number missed
- questions answered in percentage.
- If questions answered correctly is >= 70% indicate passed, otherwise state failed.
The main function
The main will prompt for the student answers file name and call the read function.
If the read function fails the program should output an error message and quit.
If the first read works the main should prompt for an read in the correct answers file name and call the read function a second time.
If the read fails an error message should be displayed and main should quit.
If the number of student answers is not the same as the number of correct answers an error message should be displayed and main should quit.
If the number of student answers and correct answers are the same but are both 0 display an error message and quit.
If your program gets this far call the work/display function.
As always you cannot use any global variables in your program.
See the sample runs for examples of the prompts, error messages, and results output.
See the sample runs for the output requirements.
Sample run 1 (valid data)
Contents of cin:
student.txt answers.txt
Contents of student.txt:
A
B
C
D
A
B
C
D
A
B
C
D
A
B
C
D
A
B
C
D
Contents of answers.txt:
A
A
A
A
B
B
B
B
C
C
C
C
D
D
D
D
A
B
C
D
Here is the output to cout:
Enter student answers file name
Enter correct answer file name
Question 2 has incorrect answer 'B', the correct answer is 'A'
Question 3 has incorrect answer 'C', the correct answer is 'A'
Question 4 has incorrect answer 'D', the correct answer is 'A'
Question 5 has incorrect answer 'A', the correct answer is 'B'
Question 7 has incorrect answer 'C', the correct answer is 'B'
Question 8 has incorrect answer 'D', the correct answer is 'B'
Question 9 has incorrect answer 'A', the correct answer is 'C'
Question 10 has incorrect answer 'B', the correct answer is 'C'
Question 12 has incorrect answer 'D', the correct answer is 'C'
Question 13 has incorrect answer 'A', the correct answer is 'D'
Question 14 has incorrect answer 'B', the correct answer is 'D'
Question 15 has incorrect answer 'C', the correct answer is 'D'
12 questions were missed out of 20
The student grade is 40.0%.
The student failed
Sample run 2 (invalid student file)
Contents of cin:
invalidfile.txt answers.txt
Here is the output to cout:
Enter student answers file name File "invalidfile.txt" could not be opened

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

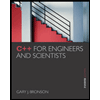
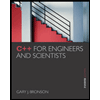