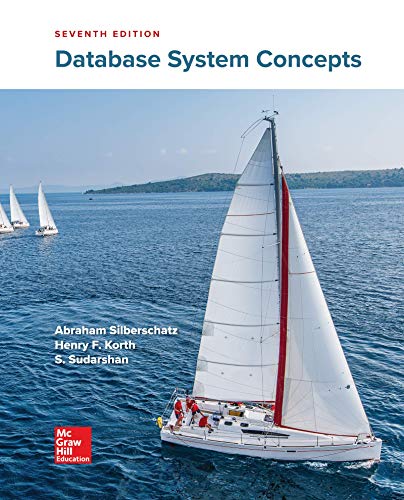
Concept explainers
Implement in C Programming
9.8.1: LAB: Parsing food data
Given a text file containing the availability of food items, write a program that reads the information from the text file and outputs the available food items. The program first reads the name of the text file from the user. The program then reads the text file, stores the information into the four string arrays predefined in the program, and outputs the available food items in the following format: name (category) -- description
Assume the text file contains the category, name, description, and availability of at least one food item, separated by a tab character ('\t').
Hints: Use the fgets() function to read each line of the input text file. When extracting texts between the tab characters, copy the texts character-by-character until a tab character is reached. A string always ends with a null character ('\0').
Ex: If the input of the program is:
food.txt
and the contents of food.txt are:
Sandwiches Ham sandwich Classic ham sandwich Available Sandwiches Chicken salad sandwich Chicken salad sandwich Not available Sandwiches Cheeseburger Classic cheeseburger Not available Salads Caesar salad Chunks of romaine heart lettuce dressed with lemon juice Available Salads Asian salad Mixed greens with ginger dressing, sprinkled with sesame Not available Beverages Water 16oz bottled water Available Beverages Coca-Cola 16oz Coca-Cola Not available Mexican food Chicken tacos Grilled chicken breast in freshly made tortillas Not available Mexican food Beef tacos Ground beef in freshly made tortillas Available Vegetarian Avocado sandwich Sliced avocado with fruity spread Not available
the output of the program is:
Ham sandwich (Sandwiches) -- Classic ham sandwich Caesar salad (Salads) -- Chunks of romaine heart lettuce dressed with lemon juice Water (Beverages) -- 16oz bottled water Beef tacos (Mexican food) -- Ground beef in freshly made tortillas
#include <stdio.h>
#include <string.h>
int main(void) {
const int MAX_LINES = 25; // Maximum number of lines in the input text file
const int MAX_STRING_LENGTH = 100; // Maximum number of characters in each column of the input text file
const int MAX_LINE_LENGTH = 200; // Maximum number of characters in each line of the input text file
// Declare 4 string arrays to store the 4 columns from the input text file
char column1[MAX_LINES][MAX_STRING_LENGTH];
char column2[MAX_LINES][MAX_STRING_LENGTH];
char column3[MAX_LINES][MAX_STRING_LENGTH];
char column4[MAX_LINES][MAX_STRING_LENGTH];
/* Type your code here. */
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- Computer Science csv file "/dsa/data/all_datasets/texas.csv" Task 6: Write a function "county_locator" that allows a user to enter in a name of a spatial data frame and a county name and have a map generated that shows the location of that county with respect to other counties (by using different colors and/or symbols). Hint: function(); $county ==; plot(); add=TRUE.arrow_forwardC++ Code: Write a program that finds word differences between two sentences. The input begins with an integer indicating the number of words in each sentence. The next input line is the first sentence and the following input line is the second sentence. Assume that the two sentences have the same number of words and no more than 20 words each. The program displays word pairs that differ between the two sentences. One pair is displayed per line. Ex: If the input is: 6 Smaller cars get better gas mileage Tiny cars get great fuel economy then the output is: Smaller Tiny better great gas fuel mileage economy Add a function named ReadSentences to read the input sentences into two string vectors. void ReadSentences(vector<string>& sentence1Words, vector<string>& sentence2Words, int wordCount) main() already contains code to read the word count and call ReadSentences(). Complete main() to display differing word pairs.arrow_forwardJAVA PROGRAM Homework #2. Chapter 7. PC #13. Name Search (page 492) Read these instructions for additional requirements carefully. Write a program that reads the contents of the two files into two separate arrays. The user should be able to enter a name the application will display messages indicating whether the names were among the most popular. 1. GirlNames.txt and BoyNames.txt contain a list of the 200 most popular names given to girls/boys born in the United States for the years 2000 through 2009. 2. Your application should use an array to hold the names. 3. The program should continue interacting with the user indefinitely unless the user chooses to quit by entering "QUIT" (should be case insensitive). 4. The user should enter a single name and the program will search the name in both lists. The user SHOULD NOT specify whether the search is for girls name or boys name. The program is responsible for finding either or both and telling the user where it found it. 5.…arrow_forward
- JAVA 8.13 LAB: Movie show time display Write a program that reads movie data from a csv (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the csv file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator (|) in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the csv file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Ex: If the input of the program…arrow_forward# dates and times with lubridateinstall.packages("nycflights13") library(tidyverse)library(lubridate)library(nycflights13) Qustion: Create a function called date_quarter that accepts any vector of dates as its input and then returns the corresponding quarter for each date Examples: “2019-01-01” should return “Q1” “2011-05-23” should return “Q2” “1978-09-30” should return “Q3” Etc. Use the flight's data set from the nycflights13 package to test your function by creating a new column called quarter using mutate()arrow_forwardc++ 6. Write a program which will prompt the user to enter an array of 12 characters. The program should then print the array, and then print the array again, but this time as a 4 colums by 3 rows array.arrow_forward
- write a c++ program that declares an array that can hold 20 chars. then the program outputs two upper case character which are A & Z(initials). next using a loop the program populates the array with the pair of the initials until the array is full. then the program outputs the array on one line. then the program swaps the contents of the first and last locations in the array and output the modified array on the next line.arrow_forwardThis assignment will give you practice on basic C programming. You will implement a few Cprogramsarrow_forwardC Programming Write function checkHorizontal to count how many discs of the opposing player would be flipped, it should do the following a. Return type integer b. Parameter list i. int rowii. int col iii. char board[ROW][COL] iv. char playerCharc. If the square to the left or right is a space, stop checking d. If the square to the left or right is the same character as the player’s character, save that it was a flank, stop checking e. If the square to the left or right is not the same character as the player’s character, count the disc f. If the counted discs is greater than zero AND the player found their own character, return the counted discs, otherwise return ZERO Write function checkVertical to count how many discs of the opposing player would be flipped, it should do the following a. Return type integer b. Parameter list i. int rowii. int col iii. char board[ROW][COL] iv. char playerCharc. If the square above or below is a space, stop checking d. If the square above or below is…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
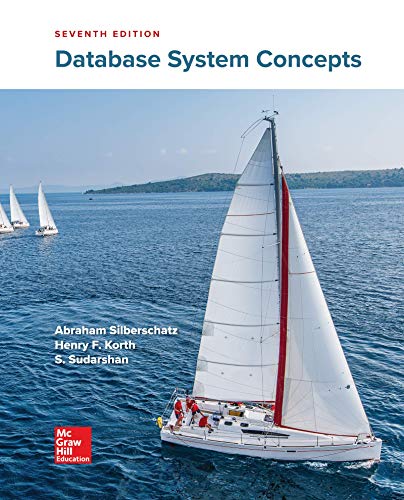
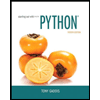
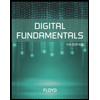
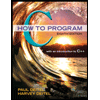
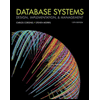
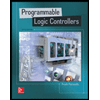