Use C++ In this lab we are going to be calculating the future value. You will need to read in the present value, the monthly interest rate and the number of months for the investment. The formula is going to compute compounded interest (by month). Here is the formula: F = P * pow((1 + i),t) Where F is the future value, P is the present value, i is the monthly interest rate and t is the number of months. The input for the program will be read in from a file. The program will write out to cout with a prompt. You will read in the file name from cin. You will then read in the present value, monthly interest rate, and the number of months from the input file. There may be multiple sets of these values. You need to process them all. The program should end when you reach the end of file on the input file. The output from the program will be written to two different places. Any error messages will be written to cout. You will also be writing out to a file called output.xls. The first thing you will write out are four "headings" separated by tabs (using the \t escape sequence). Here is an output statement (this example is using cout). Your program will instead write to the output file. cout << "Future Value\tPresent Value\tMonthly Interest\tMonths" << endl; The numbers read in must be positive values greater than 0. If they are not you need to output the three read in values to cout and display an error message saying the values are not all greater than 0. Note that the present value should be output in fixed format with two digits to the right of the decimal point. The monthly interest should be output in fixed format with three digits to the right of the decimal point. Assuming the following input in the input file: -10000 1.1 48 The following would be written to cout: Enter input file name -10000.00 1.100 48 One or more of the above values are not greater than zero Your program must have at least four functions (including main). Function to read data from the file One function will read in the input values from the input file. Each call will read in the present value, monthly interest rate, and number of months. Note that the monthly interest rate will be a number such as 10 or 12.5. These are to be read in as percentages (10% and 12.5%). You will need to divide these values by 100 to convert them into the values needed in the function before you call the futureValue function (.1 and .125 for the above values). The function will return an unsigned int value that indicates if the call worked or not. A return value of 1 indicates that the values were read properly, a return value of 2 indicates that the input data was invalid, and a return value of 0 indicates that we have reached the end of file. The calling function (main) will use this return value to indicate how to process any data read in by the function. Note that we have to read three values from a file. The input file has already been opened. That means you will have to pass the input file as a parameter to the read function. The function can update the variables passed to it with the values read in from the file. Function to calculate the future value This function must have the following signature: double futureValue(double presentValue, double interestRate, int months) The function needs to calculate the future value and return it to the calling function. The formula for calculating the future value is show at the start of this lab lesson. Function to write the values to the output file There is no return value from the function Finally the main function The logic for your main will be as follows: Write out a prompt of "Enter input file name" to cout 2 Read in the file name from the console Open the input file If the input file did not open write out an error message and stop processing Open the output file If the open failed close the input file, display an error message and stop processing Write the headings to the output file. Loop while we do not have an end of file condition Call an input function that reads in the present value, interest rate and number of months If the input is valid calculate the future value and display the results. If the input is not valid display the data and an error message End of loop Close the input and output files Assume the following is read in from cin input.txt The input.txt file contains: 100 .99 36 The contents of output.xls after the run: Future Value Present Value Monthly Interest Months 142.57 100.00 0.990 36 To do this it has to find your main function. Right now zyBooks has a problem with this when your int main() statement has a comment on it. For example: If your main looks as follows: int main() // main function You will get an error message: Could not find main function You need to change your code to: // main function int main() If you see extra output if (inputFile >> var1 >> var2 >> var3) { // the read worked. Do your processing here } else { // you have reached end of file }
Use C++
In this lab we are going to be calculating the future value. You will need to read in the present value, the monthly interest rate and the number of months for the investment. The formula is going to compute compounded interest (by month).
Here is the formula:
F = P * pow((1 + i),t)
Where F is the future value, P is the present value, i is the monthly interest rate and t is the number of months.
The input for the program will be read in from a file.
The program will write out to cout with a prompt.
You will read in the file name from cin.
You will then read in the present value, monthly interest rate, and the number of months from the input file.
There may be multiple sets of these values. You need to process them all.
The program should end when you reach the end of file on the input file.
The output from the program will be written to two different places.
Any error messages will be written to cout.
You will also be writing out to a file called output.xls.
The first thing you will write out are four "headings" separated by tabs (using the \t escape sequence).
Here is an output statement (this example is using cout). Your program will instead write to the output file.
cout << "Future Value\tPresent Value\tMonthly Interest\tMonths" << endl;
The numbers read in must be positive values greater than 0. If they are not you need to output the three read in values to cout and display an error message saying the values are not all greater than 0. Note that the present value should be output in fixed format with two digits to the right of the decimal point. The monthly interest should be output in fixed format with three digits to the right of the decimal point.
Assuming the following input in the input file:
-10000 1.1 48
The following would be written to cout:
Enter input file name
-10000.00 1.100 48
One or more of the above values are not greater than zero
Your program must have at least four functions (including main).
Function to read data from the file
One function will read in the input values from the input file. Each call will read in the present value, monthly interest rate, and number of months.
Note that the monthly interest rate will be a number such as 10 or 12.5. These are to be read in as percentages (10% and 12.5%). You will need to divide these values by 100 to convert them into the values needed in the function before you call the futureValue function (.1 and .125 for the above values).
The function will return an unsigned int value that indicates if the call worked or not. A return value of 1 indicates that the values were read properly, a return value of 2 indicates that the input data was invalid, and a return value of 0 indicates that we have reached the end of file. The calling function (main) will use this return value to indicate how to process any data read in by the function. Note that we have to read three values from a file. The input file has already been opened. That means you will have to pass the input file as a parameter to the read function. The function can update the variables passed to it with the values read in from the file.
Function to calculate the future value
This function must have the following signature:
double futureValue(double presentValue, double interestRate, int months)
The function needs to calculate the future value and return it to the calling function.
The formula for calculating the future value is show at the start of this lab lesson.
Function to write the values to the output file
There is no return value from the function
Finally the main function
The logic for your main will be as follows:
- Write out a prompt of "Enter input file name" to cout 2 Read in the file name from the console
- Open the input file
- If the input file did not open write out an error message and stop processing
- Open the output file
- If the open failed close the input file, display an error message and stop processing
- Write the headings to the output file.
- Loop while we do not have an end of file condition
- Call an input function that reads in the present value, interest rate and number of months
- If the input is valid calculate the future value and display the results.
- If the input is not valid display the data and an error message
- End of loop
- Close the input and output files
Assume the following is read in from cin
input.txt
The input.txt file contains:
100 .99 36
The contents of output.xls after the run:
Future Value Present Value Monthly Interest Months 142.57 100.00 0.990 36
To do this it has to find your main function.
Right now zyBooks has a problem with this when your int main() statement has a comment on it.
For example:
If your main looks as follows:
int main() // main function
You will get an error message:
Could not find main function
You need to change your code to:
// main function int main()
If you see extra output
if (inputFile >> var1 >> var2 >> var3) { // the read worked. Do your processing here } else { // you have reached end of file }

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

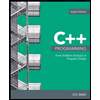
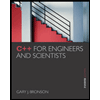
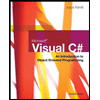
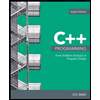
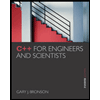
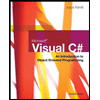