You must implement a C++ program that calculates the position and velocity of a body thrown with an initial velocity (v0) and with an angle to the horizontal (angle). The results should be displayed on the screen every some time (0.1 seconds) in a horizontal row. To validate your program, use the following example run where v0=10 and angle=53o as shown in the image: The data must be printed with two decimal places, find out how to do this in C++. Once all the calculations are printed, the program must ask if you want to repeat or if you want to exit the program. You implement the way to do it. As always the report should include (use the standard report): Cover page with title, course and section, names and student numbers of your team members in alphabetical order by last name. Introduction indicating in one paragraph what the program does and pseudocode/algorithm of the program. Detailed flowchart. Source code. Pictures of a running example. Next, the theory of ballistics problems: Ballistics problems all start with an initial velocity due to the force of the gun. It is divided into two components, horizontal and vertical velocity.Suppose a cannon is fired with an angle to the horizontal of θ=53 degrees and initial velocity v0=10m/s. The velocity is decomposed into two components: v0x = v0cosθ = 6.01815m/s. v0y = v0sinθ = 7.98636m/s. The vertical velocity at each instant is calculated at any time t by subtracting from the initial velocity the negative velocity generated by the force of gravity (g=9.81). The formula is as follows: vy(t) = v0y - gt. The horizontal velocity at each instant is constant at all times (it is assumed that the air friction is is assumed to be zero). vx(t) = v0x The horizontal position at each instant is calculated with the formula: x(t)= vx(t)t The vertical position at each instant is calculated taking into account the initial vertical velocity and the force of gravity according to the formula: y(t) = v0yt - 0.5gt2. The program should end when the bullet returns to the ground (y=0). To calculate the time where the bullet reaches the ground the ground we must know the following. The time at which the ball reaches the maximum height is calculated by obtaining the time at which the vertical velocity is zero: vy(t) = 0 = v0y - gthmax. Therefore, thmax = v0y/g When the ball reaches the ground it has had to go up and down, therefore, tmax = 2thmax. The program must do the calculations up to that maximum time when the bullet is in the air. In short, the first thing the program has to do is to calculate the time the bullet will be in the air (tmax). To calculate the positions and velocities you must implement a for-loop, do the calculations every 0.1 seconds and print up to the maximum time, even if it is not a multiple of 0.1. Translated with www.DeepL.com/Translator (free version)
You must implement a C++ program that calculates the position and velocity of a body thrown with an initial velocity (v0) and with an angle to the horizontal (angle). The results should be displayed on the screen every some time (0.1 seconds) in a horizontal row. To validate your program, use the following example run where v0=10 and angle=53o as shown in the image:
The data must be printed with two decimal places, find out how to do this in C++.
Once all the calculations are printed, the program must ask if you want to repeat or if you want to exit the program. You implement the way to do it.
As always the report should include (use the standard report):
Cover page with title, course and section, names and student numbers of your team members in alphabetical order by last name.
Introduction indicating in one paragraph what the program does and pseudocode/
Next, the theory of ballistics problems:
Ballistics problems all start with an initial velocity due to the force of the gun. It is divided into two components, horizontal and vertical velocity.Suppose a cannon is fired with an angle to the horizontal of θ=53 degrees and initial velocity v0=10m/s. The velocity is decomposed into two components:
v0x = v0cosθ = 6.01815m/s. v0y = v0sinθ = 7.98636m/s.
The vertical velocity at each instant is calculated at any time t by subtracting from the initial velocity the negative velocity generated by the force of gravity (g=9.81). The formula is as follows:
vy(t) = v0y - gt.
The horizontal velocity at each instant is constant at all times (it is assumed that the air friction is
is assumed to be zero).
vx(t) = v0x
The horizontal position at each instant is calculated with the formula:
x(t)= vx(t)t
The vertical position at each instant is calculated taking into account the initial vertical velocity and the force of gravity according to the formula:
y(t) = v0yt - 0.5gt2.
The program should end when the bullet returns to the ground (y=0). To calculate the time where the bullet reaches the ground the ground we must know the following.
The time at which the ball reaches the maximum height is calculated by obtaining the time at which the vertical velocity is zero:
vy(t) = 0 = v0y - gthmax.
Therefore, thmax = v0y/g
When the ball reaches the ground it has had to go up and down, therefore, tmax = 2thmax. The program must do the calculations up to that maximum time when the bullet is in the air.
In short, the first thing the program has to do is to calculate the time the bullet will be in the air (tmax). To calculate the positions and velocities you must implement a for-loop, do the calculations every 0.1 seconds and print up to the maximum time, even if it is not a multiple of 0.1.
Translated with www.DeepL.com/Translator (free version)
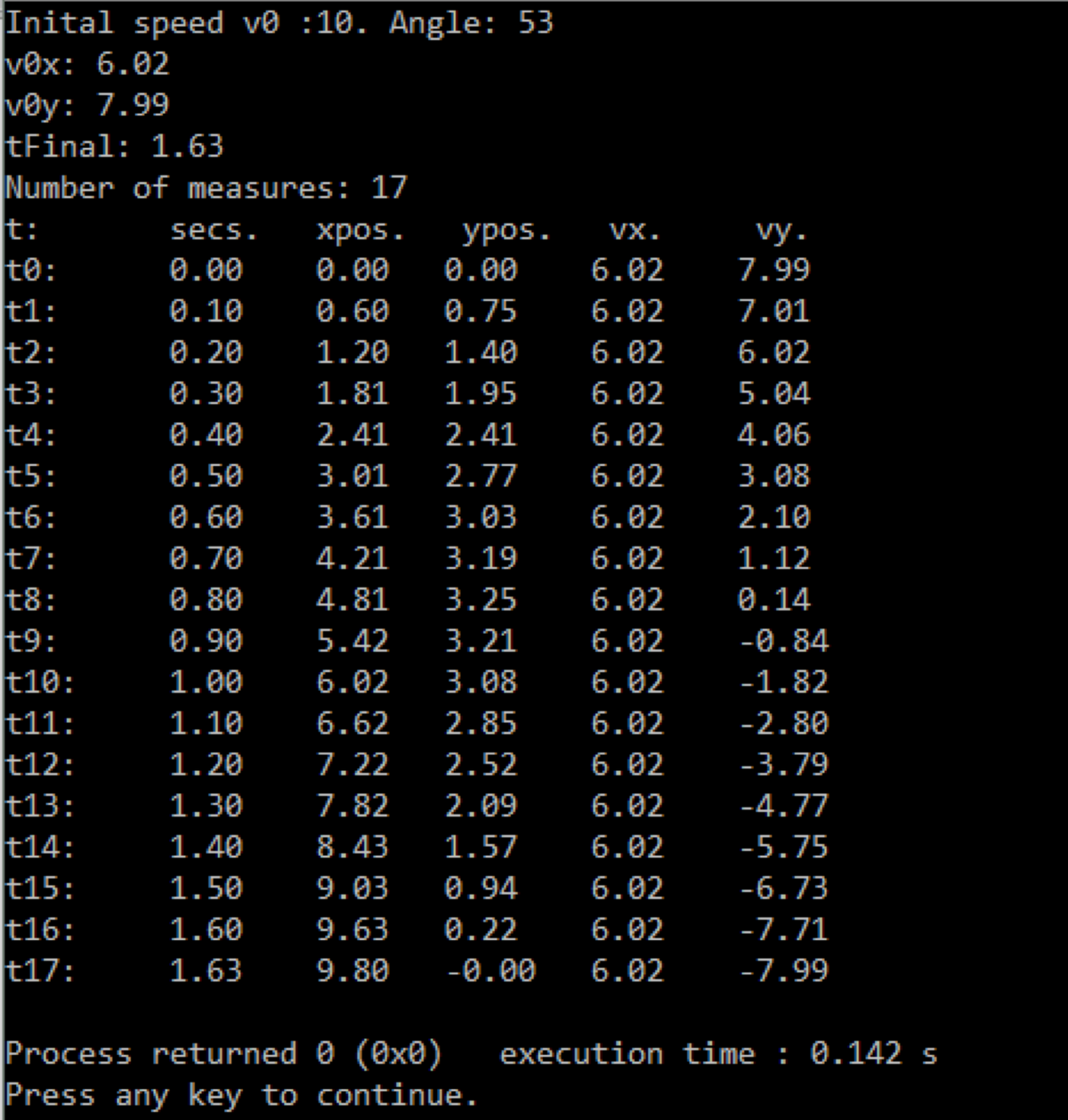

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

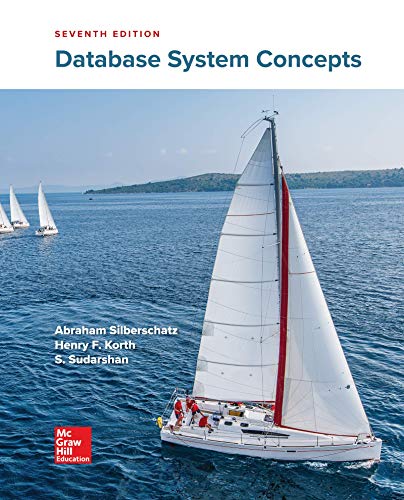
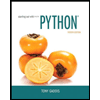
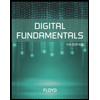
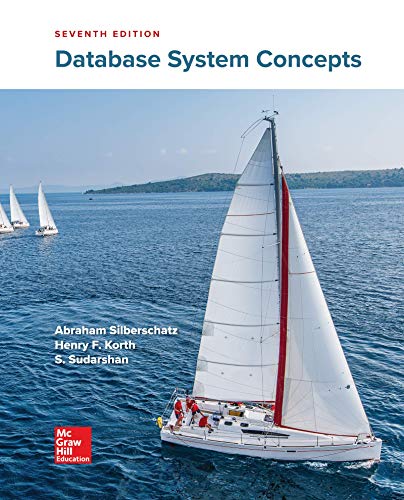
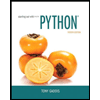
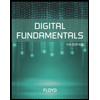
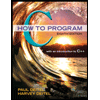
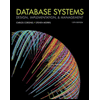
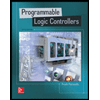