USE C++ ONLY! Consider the following class Time, which represents a time using three ints for hour, minute and second: class Time { private: int hour; int min; int sec; public: Time() { hour = min = sec = 0; } Time(int h, int m, int s) { hour = h; min = m; sec = s; } bool operator==(Time&); Time operator++(); void displayTime(); }; Time objects use a 24 hour clock. You may assume that all objects of class Time are valid, i.e. hour is between 0 and 23, inclusive; and both min and sec are between 0 and 59, inclusive. Write C++ code to do the following: 1. Implement the two overloaded operators for the class Time above: the is equal operator == should return true if the Time objects are equivalent and false if not. the prefix increment operator ++ should increment the Time object by one second. Note that this is quite simple for most times, but you do have to account for the cases where sec and/or min are 59. If the time is 23:59:59, it should increment to 0:0:0. At the end of the function, return *this. 2. Implement displayTime() for the class Time above. It should output the object's time in format hour:minute:second. You don't need to make the formatting pretty. 3. Write a simple main() function which demonstrates that your class works properly by doing the following: Create two valid Time objects. They should be the same time. The names of the objects and the time to use are up to you. Call displayTime() for each of the objects to output their time. Demonstrate that the == operator works by comparing the two time objects and printing out what it returns (it should be 1 for true). Demonstrate that the ++ operator works by calling it on one of the Time objects. Note you have implemented the prefix increment, so if your Time object is t, you can do ++t but not t++. Once again, call displayTime() for each of the objects to output their time. Once again, demonstrate that the == operator works by comparing the two times and printing out what it returns (it should be 0 for false now).
USE C++ ONLY!
Consider the following class Time, which represents a time using three ints for hour, minute and second:
class Time { private: int hour; int min; int sec; public: Time() { hour = min = sec = 0; } Time(int h, int m, int s) { hour = h; min = m; sec = s; } bool operator==(Time&); Time operator++(); void displayTime(); };
Time objects use a 24 hour clock. You may assume that all objects of class Time are valid, i.e. hour is between 0 and 23, inclusive; and both min and sec are between 0 and 59, inclusive.
Write C++ code to do the following:
1. Implement the two overloaded operators for the class Time above:
- the is equal operator == should return true if the Time objects are equivalent and false if not.
- the prefix increment operator ++ should increment the Time object by one second. Note that this is quite simple for most times, but you do have to account for the cases where sec and/or min are 59. If the time is 23:59:59, it should increment to 0:0:0. At the end of the function, return *this.
2. Implement displayTime() for the class Time above. It should output the object's time in format hour:minute:second. You don't need to make the formatting pretty.
3. Write a simple main() function which demonstrates that your class works properly by doing the following:
- Create two valid Time objects. They should be the same time. The names of the objects and the time to use are up to you.
- Call displayTime() for each of the objects to output their time.
- Demonstrate that the == operator works by comparing the two time objects and printing out what it returns (it should be 1 for true).
- Demonstrate that the ++ operator works by calling it on one of the Time objects. Note you have implemented the prefix increment, so if your Time object is t, you can do ++t but not t++.
- Once again, call displayTime() for each of the objects to output their time.
- Once again, demonstrate that the == operator works by comparing the two times and printing out what it returns (it should be 0 for false now).

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

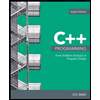
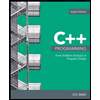