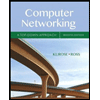
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
here's the 3rd.
Vehicle.cpp
// Vehicle.cpp
#include <iostream>
using namespace std;
class Vehicle
{
public:
void setSpeed(double);
double getSpeed();
void accelerate(double);
void setFuelCapacity(double);
double getFuelCapacity();
void setMaxSpeed(double);
double getMaxSpeed();
private:
double fuelCapacity;
double maxSpeed;
double currentSpeed;
};
void Vehicle::setSpeed(double speed)
{
currentSpeed = speed;
return;
}
double Vehicle::getSpeed()
{
return currentSpeed;
}
void Vehicle::accelerate(double mph)
{
if(currentSpeed + mph < maxSpeed)
currentSpeed = currentSpeed + mph;
else
cout << "This vehicle cannot go that fast." << endl;
}
void Vehicle::setFuelCapacity(double fuel)
{
fuelCapacity = fuel;
}
double Vehicle::getFuelCapacity()
{
return fuelCapacity;
}
void Vehicle::setMaxSpeed(double max)
{
maxSpeed = max;
}
double Vehicle::getMaxSpeed()
{
return maxSpeed;
}
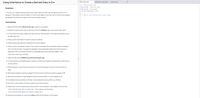
Transcribed Image Text:Using Inheritance to Create a Derived Class in C++
Motorcycle.cpp
MyMotorcycleClassPr. Vehicle.cpp
1 // Motorcycle.cpp
2 #include "Vehicle.cpp"
Summary
3 #include <iostream>
4 using namespace std;
In this lab, you create a derived class from a base class, and then use the derived class in a C++
program. The program should create two Motorcycle objects, and then set the Motorcycle's speed,
6 // Write the Motorcycle class here
7
accelerate the Motorcycle object, and check its sidecar status.
Instructions
1. Ensure the file named Motorcycle.cpp is open in your editor.
2. Create the Motorcycle class by deriving it from the Vehicle class. Use a public derivation.
3. In the Motorcycle class, create a private attribute named sidecar. The sidecar attribute should
be data type bool.
4. Write a public set method to set the value for sidecar.
5. Write a public get method to retrieve the value of sidecar.
6. Write a public accelerate() method. This method overrides the accelerate() method inherited
from the Vehicle class. Change the message in the accelerate() method so the following is
displayed when the Motorcycle tries t
accelerate beyond its maximum speed: "This
motorcycle cannot go that fast".
7. Open the file named MyMotorcycleClassProgram.cpp.
8. In the MyMotorcycleClassProgram, create two Motorcycle objects named motorcycleOne and
motorcycle Two.
9. Set the sidecar value of motorcycleOne to true and the sidecar value
motorcycleTwo to
false.
10. Set motorcycleOne's maximum speed to 90 and motorcycleTwo's maximum speed to 85
11. Set motorcycleOne's current speed to 65 and motorcycleTwo's current speed to 60.
12. Accelerate motorcycleOne by 30 mph, and accelerate motorcycleTwo by 20 mph.
13. Print the current speed of motorcycleOne and motorcycleTwo.
14. Determine if motorcycleOne and motorcycleTwo have sidecars. If yes, display the following:
This motorcycle has a side car. If not, display the following:
This motorcycle does not have a side car.
15. Execute the program by clicking the Run button at the bottom of the screen.
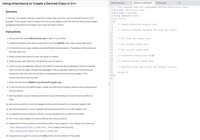
Transcribed Image Text:Using Inheritance to Create a Derived Class in C++
Motorcycle.cpp
MyMotorcycleClassPr. Vehicle.cpp
| +
1// This program uses the programmer-defined Motorcycle class.
2 #include "Motorcycle.cpp"
Summary
3 #include <iostream>
4 using namespace std;
5 int main()
6 1
In this lab, you create a derived class from a base class, and then use the derived class in a C++
program. The program should create two Motorcycle objects, and then set the Motorcycle's speed.
accelerate the Motorcycle object, and check its sidecar status.
7
// Create Motorcyle objects here
8
9
// Create a boolean variable for side car status
Instructions
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25 }
1. Ensure the file named Motorcycle.cpp is open in your editor.
// Set side car status here
2. Create the Motorcycle class by deriving it from the Vehicle class. Use a public derivation.
// Set maximum speed here
3. In the Motorcycle class, create a private attribute named sidecar. The sidecar attribute should
// Set current speed here
be data type bool.
// Accelerate motorcyles here
4. Write a public set method to set the value for sidecar.
5. Write a public get method to retrieve the value of sidecar.
// Display current speed here
6. Write a public accelerate() method. This method overrides the accelerate() method inherited
// Determine side car status and display results.
from the Vehicle class. Change the message in the accelerate() method so the following is
return 0;
displayed when the Motorcycle tries to accelerate beyond its maximum speed: "This
motorcycle cannot go that fast".
26
7. Open the file named MyMotorcycleClassProgram.cpp.
8. In the MyMotorcycleClassProgram, create two Motorcycle objects named motorcycleOne and
motorcycle Two.
9. Set the sidecar value of motorcycleOne to true and the sidecar value of motorcycleTwo to
false.
10. Set motorcycleOne's maximum speed to 90 and motorcycleTwo's maximum speed to 85.
11. Set motorcycleOne's current speed to 65 and motorcycle Two's current speed to 60.
.
12. Accelerate motorcycleOne by 30 mph, and accelerate motorcycleTwo by 20 mph.
13. Print the current speed of motorcycleOne and motorcycleTwo.
14. Determine if motorcycleOne and motorcycleTwo have sidecars. If yes, display the following:
This motorcycle has a side car. If not, display the following:
This motorcycle does not have a side car.
15. Execute the program by clicking the Run button at the bottom of the screen.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- C++ #include <iostream>using namespace std; //creating struct to hold //student name,age and letter gradestruct student{ //data members string name; int age; char grade;};int main(){ //declaring object for the struct student *k = new student; //filling it with data k->name = "Kate"; k->age=24; k->grade='B'; ///then printing student data cout<<"Name:"<<k->name<<endl; cout<<"Age:"<<k->age<<endl; cout<<"Grade:"<<k->grade<<endl; return 0;}arrow_forward#include <iostream>using namespace std;class Player{private:int id;static int next_id;public:int getID() { return id; }Player() { id = next_id++; }};int Player::next_id = 1;int main(){Player p1;Player p2;Player p3;cout << p1.getID() << " ";cout << p2.getID() << " ";cout << p3.getID();return 0;} Run the program and give its output.arrow_forwardIn C++ class bookType { public: void setBookTitle(string s); //sets the bookTitle to s void setBookISBN(string ISBN); //sets the private member bookISBN to the parameter void setBookPrice(double cost); //sets the private member bookPrice to cost void setQuantityInStock (int noOfCopies); //sets the private member quantityInStock to noOfCopies void printInfo() const; //prints the bookTitle, bookISBN, the bookPrice and the quantityInStock string getBookISBN() const; //returns the bookISBN double getBookPrice() const; //returns the bookPrice int showQuantityInStock() const; //returns the quantityInStock void updateQuantity(int addBooks); //adds addBooks to the quantityInStock, so that the quantity in stock now has it original value plus the parameter sent to this function private: string bookTitle; string bookISBN; double bookPrice; int quantityInStock; }; Write the full function definition for updateQuantity();arrow_forward
- briefly explain this code #include<iostream>#include<string.h> using namespace std; class bank_account{int acno;char nm[100], acctype[100];float bal;public:bank_account(int acc_no, char *name, char *acc_type, float balance){acno=acc_no;strcpy(nm, name);strcpy(acctype, acc_type);bal=balance;}void deposit();void withdraw();void display();};void bank_account::deposit(){int damt1;cout<<"\n Enter Deposit Amount = ";cin>>damt1;bal+=damt1;}void bank_account::withdraw(){int wamt1;cout<<"\n Enter Withdraw Amount = ";cin>>wamt1;if(wamt1>bal)cout<<"\n Cannot Withdraw Amount";bal-=wamt1;}void bank_account::display(){cout<<"\n Name : "<<nm;cout<<"\n Account Type : "<<acctype;cout<<"\n Balance : "<<bal;}int main(){int acc_no;char name[100], acc_type[100];float balance;cout<<"\n Name : ";cin>>name;cout<<"\n Account Type : ";cin>>acc_type;cout<<"\n Balance : ";cin>>balance;bank_account…arrow_forwardChallenge 3: Vehicle.java, Automobile.java, Tank.java, Truck.java, Car.java, and TestVehicle.java Implement the classes that are given in the class diagram. Also implement a TestVehicle class where you will create three objects and execute their toString() methods. >> Automobile assignment2 a licensePlate: String make: String Automobile() Automobile(int,double, String, String) getLicensePlate(): String setLicense Plate(String):void ●getMake(): String setMake(String):void .toString(): String >> Ⓒ Truck assignment2 a towCapacity: double Truck() Truck(int, double, String, String, double) getTowCapacity():double setTowCapacity (double):void toString(): String Sample output > Vehicle assignment2 □ year: int weight: double Vehicle() Vehicle(int, double) getYear(): int setYear(int):void getWeight():double setWeight(double):void .toString(): String > Car assignment2 a maxPassengers: int Car() Car(int,double, String, String,int) getMaxPassengers() int setMaxPassengers (int):void toString():…arrow_forwardQuestion is in the image #include <iostream> using namespace std; class Game { public: Game() { cout <<"Game Started"<<endl; } //destructor goes here }; class Quiz: public Game { public: Quiz() { cout <<"Quiz Started"<<endl; } //destructor goes here }; int main() { Quiz q; }arrow_forward
- c++ programmingarrow_forward/ CONSTANT// static const int DEFAULT_CAPACITY = ____// IntSet::DEFAULT_CAPACITY is the initial capacity of an// IntSet that is created by the default constructor (i.e.,// IntSet::DEFAULT_CAPACITY is the highest # of distinct// values "an IntSet created by the default constructor"// can accommodate).//// CONSTRUCTOR// IntSet(int initial_capacity = DEFAULT_CAPACITY)// Post: The invoking IntSet is initialized to an empty// IntSet (i.e., one containing no relevant elements);// the initial capacity is given by initial_capacity if// initial_capacity is >= 1, otherwise it is given by// IntSet:DEFAULT_CAPACITY.// Note: When the IntSet is put to use after construction,// its capacity will be resized as necessary.//// CONSTANT MEMBER FUNCTIONS (ACCESSORS)// int size() const// Pre: (none)// Post: Number of elements in the invoking IntSet is returned.// bool isEmpty() const// Pre: (none)//…arrow_forward// CONSTANT// static const int DEFAULT_CAPACITY = __10__// IntSet::DEFAULT_CAPACITY is the initial capacity of an// IntSet that is created by the default constructor (i.e.,// IntSet::DEFAULT_CAPACITY is the highest # of distinct// values "an IntSet created by the default constructor"// can accommodate).//// CONSTRUCTOR// IntSet(int initial_capacity = DEFAULT_CAPACITY)// Post: The invoking IntSet is initialized to an empty// IntSet (i.e., one containing no relevant elements);// the initial capacity is given by initial_capacity if// initial_capacity is >= 1, otherwise it is given by// IntSet:DEFAULT_CAPACITY.// Note: When the IntSet is put to use after construction,// its capacity will be resized as necessary.//// CONSTANT MEMBER FUNCTIONS (ACCESSORS)// int size() const// Pre: (none)// Post: Number of elements in the invoking IntSet is returned.// bool isEmpty() const// Pre: (none)//…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
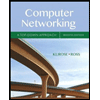
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
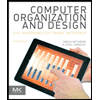
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
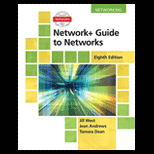
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
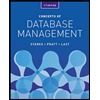
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
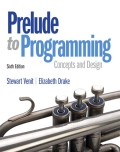
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
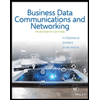
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY