Using pyhton coding to solve: Suppose the Great Frederick Fair wants to update its ticketing software. They need you to write a program to handle the price calculations, using the rules*: ● The basic price of a ticket is $40. Senior citizens (age >= 65) get a 50% discount. Children under 6 are free (100% discount). For residents of Frederick County, the basic price is $35; the same discounts still apply. So the individual ticket prices range from $0 to $40. ● . Your program should request age and county name from the user. The age will be entered as an integer and the county name as a string. Before calculating the price, confirm that the user's age is valid - not negative and not more than 110. If it is not, give a message and do not do the price calculation. Also, the county name should not be case sensitive - for example, Frederick, frederick, and FREDERICK should all be acceptable. Your program should then calculate and print out the ticket price, using the appropriate discounts. Test your program with a variety of ages and counties to be sure you have considered all the conditions. Here are some samples. Test run # 1 2 3 4 5 6 7 8 9 County Frederick Frederick Carroll Howard Washington Frederick Montgomery Carroll Frederick Age 12 72 2 65 0 5 6 35 -15
Using pyhton coding to solve: Suppose the Great Frederick Fair wants to update its ticketing software. They need you to write a program to handle the price calculations, using the rules*: ● The basic price of a ticket is $40. Senior citizens (age >= 65) get a 50% discount. Children under 6 are free (100% discount). For residents of Frederick County, the basic price is $35; the same discounts still apply. So the individual ticket prices range from $0 to $40. ● . Your program should request age and county name from the user. The age will be entered as an integer and the county name as a string. Before calculating the price, confirm that the user's age is valid - not negative and not more than 110. If it is not, give a message and do not do the price calculation. Also, the county name should not be case sensitive - for example, Frederick, frederick, and FREDERICK should all be acceptable. Your program should then calculate and print out the ticket price, using the appropriate discounts. Test your program with a variety of ages and counties to be sure you have considered all the conditions. Here are some samples. Test run # 1 2 3 4 5 6 7 8 9 County Frederick Frederick Carroll Howard Washington Frederick Montgomery Carroll Frederick Age 12 72 2 65 0 5 6 35 -15

Here is a Python code that solves the problem:-
# ask the user for age and county name
age = int(input("Enter your age: "))
county = input("Enter your county name: ").lower()
# check if age is valid
if age < 0 or age > 110:
print("Invalid age.")
else:
# calculate price based on age and county
if age < 6:
price = 0
elif age >= 65:
price = 20
else:
price = 40
if county == "frederick":
price -= 5
# print ticket price
print("Your ticket price is $", price, ".", sep="")
To test the code, you can run it with different age and county inputs, like the ones given in the problem statement.
Step by step
Solved in 3 steps with 1 images

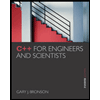
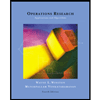
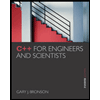
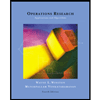