Using python update the code below to play 1,000,000 games of craps. Use a wins dictionary to keep track of the number of games won for a particular number of rolls. Similarly, use a losses dictionary to keep track of the number of games lost for a particular number of rolls. As the simulation proceeds, keep updating the dictionaries. A typical key–value pair in the wins dictionary might be 4: 50217 indicating that 50217 games were won on the 4th roll. Display a summary of the results including: a) the percentage of the total games played that were won. b) the percentage of the total games played that were lost. c) the percentages of the total games played that were won or lost on a given roll (column 2 of the sample output). d) the cumulative percentage of the total games played that were won or lost up to and including a given number of rolls (column 3 of the sample output). import random def roll_dice(): die1 = random.randrange(1, 7) die2 = random.randrange(1, 7) return (die1, die2) def display_dice(dice): die1, die2 = dice print(f'Player rolled {die1} + {die2} = {sum(dice)}') die_values = roll_dice() display_dice(die_values) sum_of_dice = sum(die_values) if sum_of_dice in (7, 11): game_status = 'WON' elif sum_of_dice in (2, 3, 12): game_status = 'LOST' else: game_status = 'CONTINUE' my_point = sum_of_dice print('Point is', my_point) while game_status == 'CONTINUE': die_values = roll_dice() display_dice(die_values) sum_of_dice = sum(die_values) if sum_of_dice == my_point: game_status = 'WON' elif sum_of_dice == 7: game_status = 'LOST' if game_status == 'WON': print('Player wins') else: print('Player loses')
Using python update the code below
to play 1,000,000 games of craps.
Use a wins dictionary to keep track of the number of games won for a particular number of rolls. Similarly, use a losses dictionary to keep track of the number of games lost for a particular number of rolls. As the simulation proceeds, keep updating the dictionaries.
A typical key–value pair in the wins dictionary might be
4: 50217
indicating that 50217 games were won on the 4th roll.
Display a summary of the results including:
a) the percentage of the total games played that were won.
b) the percentage of the total games played that were lost.
c) the percentages of the total games played that were won or lost on a given roll (column 2 of the sample output).
d) the cumulative percentage of the total games played that were won or lost up to and including a given number of rolls (column 3 of the sample output).
import random
def roll_dice():
die1 = random.randrange(1, 7)
die2 = random.randrange(1, 7)
return (die1, die2)
def display_dice(dice):
die1, die2 = dice
print(f'Player rolled {die1} + {die2} = {sum(dice)}')
die_values = roll_dice()
display_dice(die_values)
sum_of_dice = sum(die_values)
if sum_of_dice in (7, 11):
game_status = 'WON'
elif sum_of_dice in (2, 3, 12):
game_status = 'LOST'
else:
game_status = 'CONTINUE'
my_point = sum_of_dice
print('Point is', my_point)
while game_status == 'CONTINUE':
die_values = roll_dice()
display_dice(die_values)
sum_of_dice = sum(die_values)
if sum_of_dice == my_point:
game_status = 'WON'
elif sum_of_dice == 7:
game_status = 'LOST'
if game_status == 'WON':
print('Player wins')
else:
print('Player loses')

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

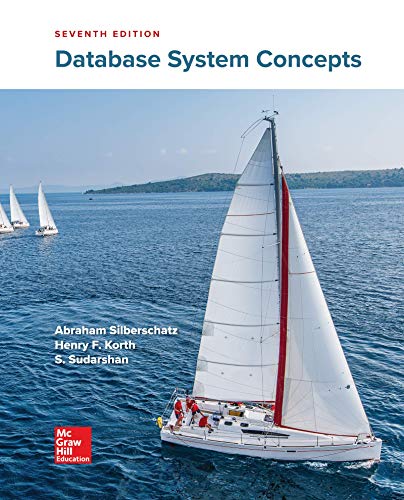
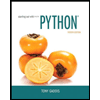
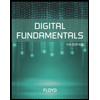
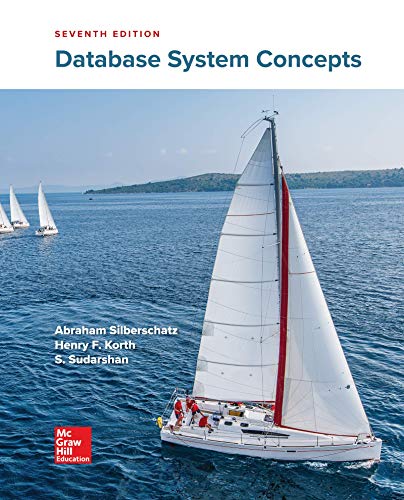
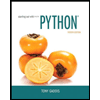
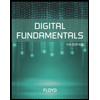
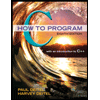
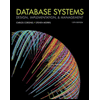
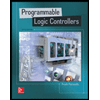