Variables: • String name - the name of the dog (can not change once set) This field should be visible to any descendant classes. An invalid value should default this field to "Clifford". A value is invalid if it is an empty String, blank String, or null. • Double length -the end-to-end length of the dog in inches. This field should be visible to any descendant classes. An invalid value should default this field to 8.0. A value is invalid if it is not a number, infinite, nonpositive, or null. (Double API) • Double weight -the weight of the Dog in ounces This field should be visible to any descendant classes. An invalid value should default this field to 2.0. A value is invalid if it is not a number, infinite, nonpositive, or null. • int totalDogs - a value tracking the number of dogs that have been introduced to the yard. This variable should only be subject to modification in the body of Dog constructors.(Consider what keyword enables a value to be tracked at the class, rather than at an instance level.) Constructors: A) A constructor that takes in name, length, and weight. B) A constructor that takes in no parameters and sets name to "Clifford", length to 5.0, and weight to 12.0. C) A copy constructor that deep copies all necessary instance fields. (Assume inputs not null) Methods: • formatLength Returns the length of the dog as a String, formatted precisely as follows "feet" ft "inches" in" feet should be displayed as an integer, and inches should be displayed to 2 decimal places with rounding. (String API) format Weight .toString Returns the weight of the dog as a String, formatted precisely as follows "pounds" lbs "ounces" oz If the weight of the dog is only one pound, the unit should instead be❝lb". pounds should be displayed as an integer, and ounces should be displayed to 2 decimal places with rounding. Overrides the corresponding method in its super class, such that a String with the following format is returned without trailing or leading whitespace): "I'm a talking dog named "name" My length is "feet" ft "inches" in and my weight is "pounds" lbs" ounces
Variables: • String name - the name of the dog (can not change once set) This field should be visible to any descendant classes. An invalid value should default this field to "Clifford". A value is invalid if it is an empty String, blank String, or null. • Double length -the end-to-end length of the dog in inches. This field should be visible to any descendant classes. An invalid value should default this field to 8.0. A value is invalid if it is not a number, infinite, nonpositive, or null. (Double API) • Double weight -the weight of the Dog in ounces This field should be visible to any descendant classes. An invalid value should default this field to 2.0. A value is invalid if it is not a number, infinite, nonpositive, or null. • int totalDogs - a value tracking the number of dogs that have been introduced to the yard. This variable should only be subject to modification in the body of Dog constructors.(Consider what keyword enables a value to be tracked at the class, rather than at an instance level.) Constructors: A) A constructor that takes in name, length, and weight. B) A constructor that takes in no parameters and sets name to "Clifford", length to 5.0, and weight to 12.0. C) A copy constructor that deep copies all necessary instance fields. (Assume inputs not null) Methods: • formatLength Returns the length of the dog as a String, formatted precisely as follows "feet" ft "inches" in" feet should be displayed as an integer, and inches should be displayed to 2 decimal places with rounding. (String API) format Weight .toString Returns the weight of the dog as a String, formatted precisely as follows "pounds" lbs "ounces" oz If the weight of the dog is only one pound, the unit should instead be❝lb". pounds should be displayed as an integer, and ounces should be displayed to 2 decimal places with rounding. Overrides the corresponding method in its super class, such that a String with the following format is returned without trailing or leading whitespace): "I'm a talking dog named "name" My length is "feet" ft "inches" in and my weight is "pounds" lbs" ounces
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 7RQ
Related questions
Question
In Java, given the parameters:
I'm having a bit of trouble how to check if the inputs would be null for Constructor A and how to create the copy constructor for Constructor C. Otherwise, is the rest of the program fine for the specific instructions?
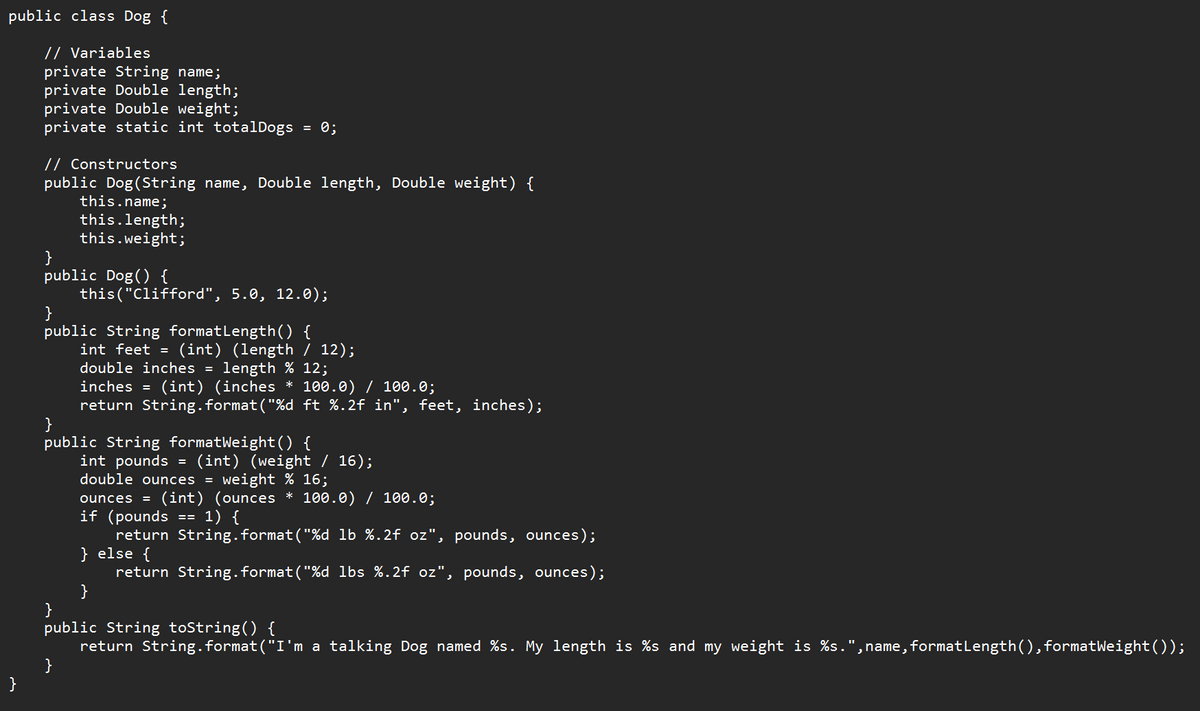
Transcribed Image Text:public class Dog {
}
// Variables
private String name;
private Double length;
private Double weight;
private static int totalDogs = 0;
// Constructors
public Dog (String name, Double length, Double weight) {
this.name;
this.length;
this.weight;
}
public Dog() {
this ("Clifford", 5.0, 12.0);
}
public String formatLength() {
}
int feet = (int) (length / 12);
double inches = length % 12;
inches = (int) (inches * 100.0) / 100.0;
return String.format("%d ft %.2f in", feet, inches);
}
public String formatWeight() {
int pounds = (int) (weight / 16);
double ounces = weight % 16;
ounces = (int) (ounces * 100.0) / 100.0;
if (pounds == 1) {
return String.format("%d lb %.2f oz", pounds, ounces);
} else {
return String.format("%d lbs %.2f oz". pounds, ounces);
}
}
public String toString() {
return String.format("I'm a talking Dog named %s. My length is %s and my weight is %s.",name, format Length(), formatWeight());
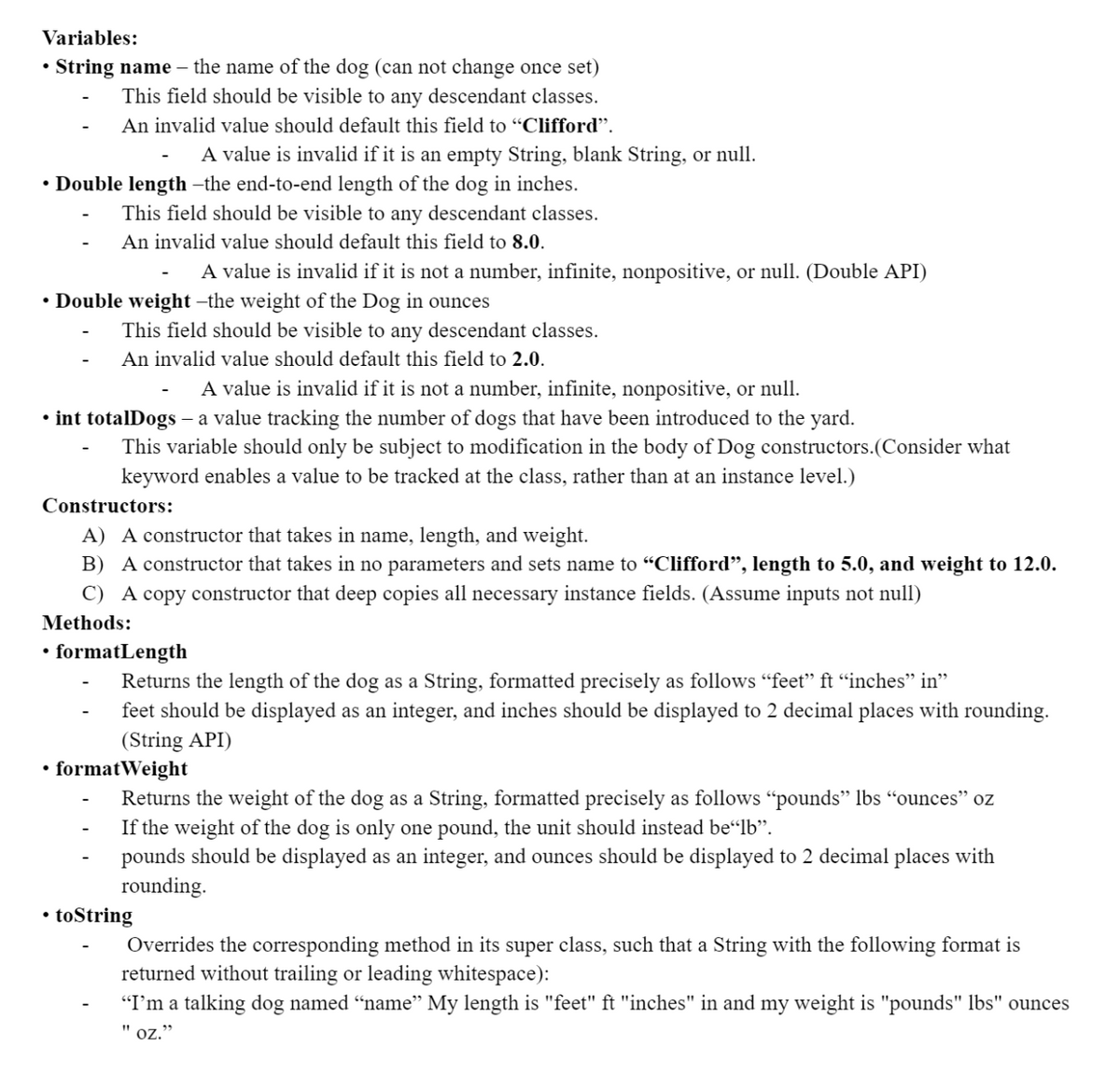
Transcribed Image Text:Variables:
• String name - the name of the dog (can not change once set)
This field should be visible to any descendant classes.
An invalid value should default this field to “Clifford”.
• Double length -the end-to-end length of the dog in inches.
This field should be visible to any descendant classes.
An invalid value should default this field to 8.0.
A value is invalid if it is an empty String, blank String, or null.
• Double weight -the weight of the Dog in ounces
A value is invalid if it is not a number, infinite, nonpositive, or null. (Double API)
-
This field should be visible to any descendant classes.
An invalid value should default this field to 2.0.
A value is invalid if it is not a number, infinite, nonpositive, or null.
• int totalDogs - a value tracking the number of dogs that have been introduced to the yard.
This variable should only be subject to modification in the body of Dog constructors.(Consider what
keyword enables a value to be tracked at the class, rather than at an instance level.)
Constructors:
A) A constructor that takes in name, length, and weight.
B) A constructor that takes in no parameters and sets name to "Clifford”, length to 5.0, and weight to 12.0.
C) A copy constructor that deep copies all necessary instance fields. (Assume inputs not null)
Methods:
• formatLength
Returns the length of the dog as a String, formatted precisely as follows "feet" ft "inches" in"
feet should be displayed as an integer, and inches should be displayed to 2 decimal places with rounding.
(String API)
format Weight
Returns the weight of the dog as a String, formatted precisely as follows "pounds" lbs "ounces" oz
If the weight of the dog is only one pound, the unit should instead be“lb”.
pounds should be displayed as an integer, and ounces should be displayed to 2 decimal places with
rounding.
• toString
Overrides the corresponding method in its super class, such that a String with the following format is
returned without trailing or leading whitespace):
"I'm a talking dog named “name” My length is "feet" ft "inches" in and my weight is "pounds" lbs" ounces
oz."
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
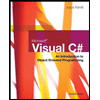
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
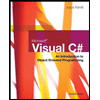
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,