Colorful bubbles In this problem, you will use the Bubble class to make images of colorful bubbles. You will use your knowledge of classes and objects to make an instance of the Bubble class (aka instantiate a Bubble), set its member variables, and use its member functions to draw a Bubble into an image. Every Bubble object has the following member variables: X coordinate Y coordinate Size (i.e. its radius) Color Complete main.cc Your task is to complete main.cc to build and draw Bubble objects based on user input. main.cc already does the work to draw the Bubble as an image saved in bubble.bmp. You should follow these steps: First, you will need to create a Bubble object from the Bubble class. Next, you must prompt the user to provide the following: an int for the X coordinate, an int for the Y coordinate, an int for the Bubble's size, and a std::string for the Bubble's color. Next, you must use the user's input to set the new Bubble object's x and y coordinates, the size, and the color using the member functions of the Bubble class. Finally, you can open the bubble.bmp file to see the bubble you've drawn. You should see a circle centered at (x, y) with a radius of size, in the given color. main.cc file #include #include "bubble.h" #include "cpputils/graphics/image.h" int main() { // Prompts the user for input to create the Bubble image. std::cout << "Tell me about your bubble.\n" << "Valid x, y and size are between 0 and 500.\n" << "Valid colors are red, orange, yellow, green, " << "teal, blue, and purple." << std::endl; std::string color; std::cout << "What color? "; std::cin >> color; int size = 0; std::cout << "What size? "; std::cin >> size; int x = 0; std::cout << "What x? "; std::cin >> x; int y = 0; std::cout << "What y? "; std::cin >> y; // ========================== YOUR CODE HERE ============================= // Instantiate a `Bubble` object into a variable called `my_bubble`. // Then, use the member functions to set color, size, and x, y coordinates // based on the user provided input retrieved above. // ======================================================================= // Don't delete this! // This prints out your bubble to the terminal and also // draws it into an image you can view in "bubble.bmp". constint image_size = 500; graphics::Image image(image_size, image_size); if (image.DrawCircle(my_bubble.GetX(), my_bubble.GetY(), my_bubble.GetSize(), my_bubble.GetColor()) && image.SaveImageBmp("bubble.bmp")) { std::cout << my_bubble.ToString() << std::endl; std::cout << "Your bubble was saved to bubble.bmp" << std::endl; } return0; } bubble.cc file #include "bubble.h" #include #include void Bubble::SetX(int x) { x_ = x; } void Bubble::SetY(int y) { y_ = y; } void Bubble::SetSize(int size) { size_ = size; } graphics::Color Bubble::GetColor() const { return color_; } int Bubble::GetX() const { return x_; } int Bubble::GetY() const { return y_; } int Bubble::GetSize() const { return size_; } void Bubble::SetColor(const std::string& color) { constint max_channel = 255; constint half_channel = 125; // Create a map from strings to graphics::Color. std::map colors; colors["red"] = graphics::Color(max_channel, 0, 0); colors["orange"] = graphics::Color(max_channel, half_channel, 0); colors["yellow"] = graphics::Color(max_channel, max_channel, 0); colors["green"] = graphics::Color(0, max_channel, 0); colors["teal"] = graphics::Color(0, max_channel, max_channel); colors["blue"] = graphics::Color(0, 0, max_channel); colors["purple"] = graphics::Color(half_channel, 0, max_channel); if (colors.find(color) == colors.end()) { // Invalid color was passed in. Default to black. std::cout << "Invalid color: " << color << ". Defaulting to black." << std::endl; color_ = graphics::Color(0, 0, 0); } else { color_ = colors[color]; } } std::string Bubble::ToString() const {
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Colorful bubbles
In this problem, you will use the Bubble class to make images of colorful bubbles. You will use your knowledge of classes and objects to make an instance of the Bubble class (aka instantiate a Bubble), set its member variables, and use its member functions to draw a Bubble into an image.
Every Bubble object has the following member variables:
- X coordinate
- Y coordinate
- Size (i.e. its radius)
- Color
Complete main.cc
Your task is to complete main.cc to build and draw Bubble objects based on user input. main.cc already does the work to draw the Bubble as an image saved in bubble.bmp. You should follow these steps:
- First, you will need to create a Bubble object from the Bubble class.
- Next, you must prompt the user to provide the following: an int for the X coordinate, an int for the Y coordinate, an int for the Bubble's size, and a std::string for the Bubble's color.
- Next, you must use the user's input to set the new Bubble object's x and y coordinates, the size, and the color using the member functions of the Bubble class.
- Finally, you can open the bubble.bmp file to see the bubble you've drawn. You should see a circle centered at (x, y) with a radius of size, in the given color.
main.cc file
#include <iostream> | |
#include "bubble.h" | |
#include "cpputils/graphics/image.h" | |
int main() { | |
// Prompts the user for input to create the Bubble image. | |
std::cout << "Tell me about your bubble.\n" | |
<< "Valid x, y and size are between 0 and 500.\n" | |
<< "Valid colors are red, orange, yellow, green, " | |
<< "teal, blue, and purple." << std::endl; | |
std::string color; | |
std::cout << "What color? "; | |
std::cin >> color; | |
int size = 0; | |
std::cout << "What size? "; | |
std::cin >> size; | |
int x = 0; | |
std::cout << "What x? "; | |
std::cin >> x; | |
int y = 0; | |
std::cout << "What y? "; | |
std::cin >> y; | |
// ========================== YOUR CODE HERE ============================= | |
// Instantiate a `Bubble` object into a variable called `my_bubble`. | |
// Then, use the member functions to set color, size, and x, y coordinates | |
// based on the user provided input retrieved above. | |
// ======================================================================= | |
// Don't delete this! | |
// This prints out your bubble to the terminal and also | |
// draws it into an image you can view in "bubble.bmp". | |
constint image_size = 500; | |
graphics::Image image(image_size, image_size); | |
if (image.DrawCircle(my_bubble.GetX(), my_bubble.GetY(), my_bubble.GetSize(), | |
my_bubble.GetColor()) && | |
image.SaveImageBmp("bubble.bmp")) { | |
std::cout << my_bubble.ToString() << std::endl; | |
std::cout << "Your bubble was saved to bubble.bmp" << std::endl; | |
} | |
return0; | |
} |
bubble.cc file
#include "bubble.h" | |
#include <map> | |
#include <string> | |
void Bubble::SetX(int x) { | |
x_ = x; | |
} | |
void Bubble::SetY(int y) { | |
y_ = y; | |
} | |
void Bubble::SetSize(int size) { | |
size_ = size; | |
} | |
graphics::Color Bubble::GetColor() const { | |
return color_; | |
} | |
int Bubble::GetX() const { | |
return x_; | |
} | |
int Bubble::GetY() const { | |
return y_; | |
} | |
int Bubble::GetSize() const { | |
return size_; | |
} | |
void Bubble::SetColor(const std::string& color) { | |
constint max_channel = 255; | |
constint half_channel = 125; | |
// Create a map from strings to graphics::Color. | |
std::map<std::string, graphics::Color> colors; | |
colors["red"] = graphics::Color(max_channel, 0, 0); | |
colors["orange"] = graphics::Color(max_channel, half_channel, 0); | |
colors["yellow"] = graphics::Color(max_channel, max_channel, 0); | |
colors["green"] = graphics::Color(0, max_channel, 0); | |
colors["teal"] = graphics::Color(0, max_channel, max_channel); | |
colors["blue"] = graphics::Color(0, 0, max_channel); | |
colors["purple"] = graphics::Color(half_channel, 0, max_channel); | |
if (colors.find(color) == colors.end()) { | |
// Invalid color was passed in. Default to black. | |
std::cout << "Invalid color: " << color << ". Defaulting to black." | |
<< std::endl; | |
color_ = graphics::Color(0, 0, 0); | |
} else { | |
color_ = colors[color]; | |
} | |
} | |
std::string Bubble::ToString() const { | |
return"Bubble at (" + std::to_string(x_) + ", " + std::to_string(y_) + | |
") with size " + std::to_string(size_); | |
} |
bubble.h file
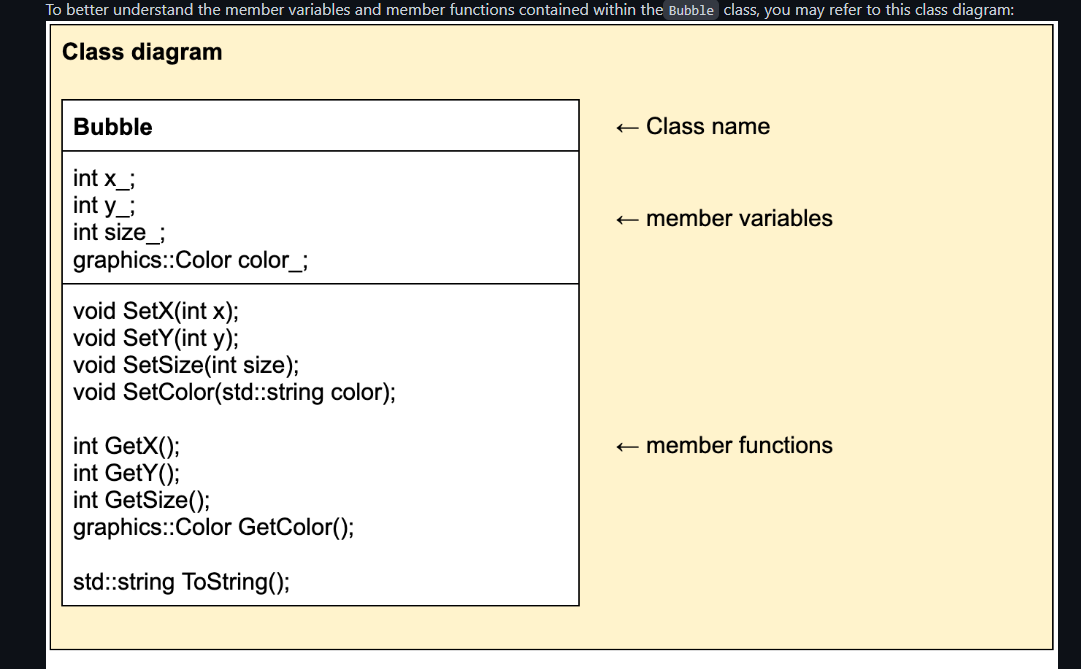
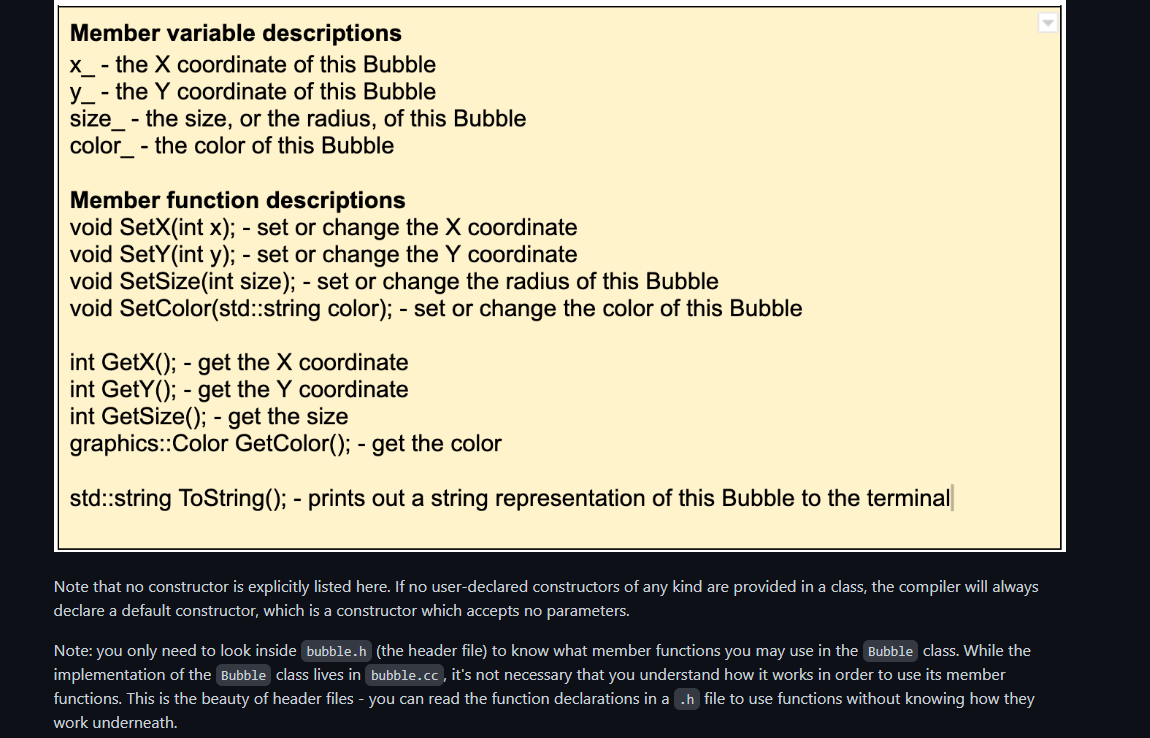

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

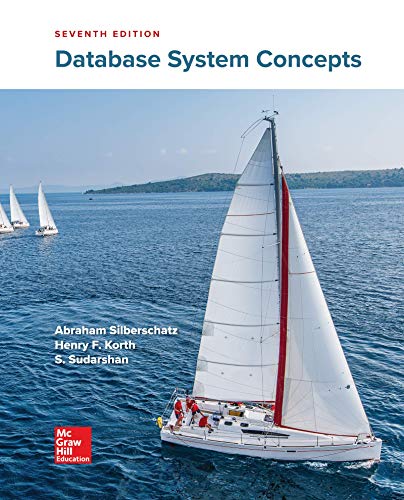
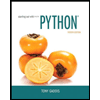
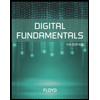
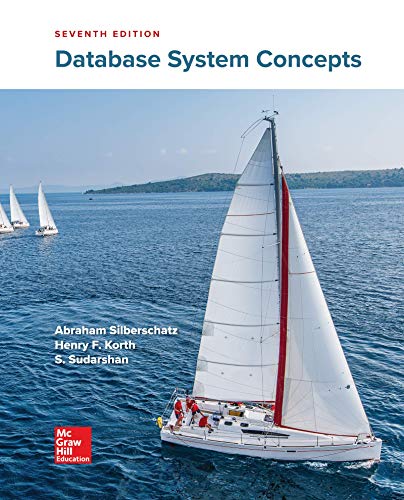
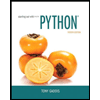
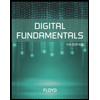
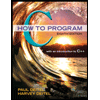
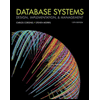
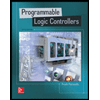