want my cashier system to automatically give the price after inputting the item name (not like what I did in this code) so that I can calculate the total cost per item in the receipt. import java.util.Scanner; class List { Scanner sc = new Scanner(System.in); private String name; private int item; private String[] des; private double[] price; private int[] quan; private double total; public List(String name, int item, String[] des, double total, double[] price) { this.name = name; this.item = item; this.des = des; this.price = price; this.total = total; } public void Welcome() { System.out.println(" \n\tWelcome to Favorite Store" + "\n\t Philippines\n" + "\n VAT Reg TIN: 123-456-789-000"); } public void Input() { System.out.print("\n Enter name of customer: "); name = sc.nextLine(); //accepts spaces as well System.out.print(" Enter number of items: "); item = sc.nextInt(); des = new String[item]; price = new double[item]; quan = new int[item]; total = 0; for (int i = 0; i < item; i++) { System.out.print("\n Enter item name: "); des[i] = sc.next(); System.out.print(" Enter price: "); price[i] = sc.nextDouble(); System.out.print(" Enter quantity: "); quan[i] = sc.nextInt(); total+=price[i]*quan[i]; //updates total } } public void Menu() { System.out.print("\n Item list:\n"); System.out.println(" Crayons\t120.00\n" + " Eraser 17.25\n" + " Folder 12.75\n" + " Glue\t\t59.75\n" + " Highlighter 36.75\n" + " Notebook\t44.75\n" + " Paper 50.00\n" + " Pen\t\t23.50\n" + " Pencil 12.00\n" + " Ruler 16.00\n" + " Scisscors 79.75\n"); } public void Receipt() { System.out.println("\n\n **********************************"); System.out.println("\t\tRECEIPT"); System.out.println(" **********************************"); System.out.println("\t We'll always be your"); System.out.println("\t Favorite Store"); System.out.println(); System.out.print(" S.No "); System.out.print(" Quantity "); System.out.print("Item name "); System.out.print(" Price "); System.out.println(); for (int i = 0; i < item; i++) { System.out.print(" 100" + i + " "); System.out.print(quan[i]+"\t " ); System.out.print(des[i] + "\t "); System.out.print(price[i]+ " "); System.out.println(); } System.out.println("\n\tSubtotal: " + this.total); double vat = (float) 0.12 * this.total; System.out.println(" \t12% VAT: "+ vat); double gtotal = (float) this.total + vat; System.out.println(" \n\tAmount Due: " + gtotal); System.out.print(" \tCash: "); float cash = (float) sc.nextDouble(); double change = cash - gtotal; System.out.println(" \tChange: " + change); int qty = 0 ; System.out.println("\n\t 12% VAT included"); for (int i = 0; i
I want my cashier system to automatically give the price after inputting the item name (not like what I did in this code) so that I can calculate the total cost per item in the receipt.
import java.util.Scanner;
class List {
Scanner sc = new Scanner(System.in);
private String name;
private int item;
private String[] des;
private double[] price;
private int[] quan;
private double total;
public List(String name, int item, String[] des, double total, double[] price) {
this.name = name;
this.item = item;
this.des = des;
this.price = price;
this.total = total;
}
public void Welcome() {
System.out.println(" \n\tWelcome to Favorite Store"
+ "\n\t Philippines\n"
+ "\n VAT Reg TIN: 123-456-789-000");
}
public void Input() {
System.out.print("\n Enter name of customer: ");
name = sc.nextLine(); //accepts spaces as well
System.out.print(" Enter number of items: ");
item = sc.nextInt();
des = new String[item];
price = new double[item];
quan = new int[item];
total = 0;
for (int i = 0; i < item; i++) {
System.out.print("\n Enter item name: ");
des[i] = sc.next();
System.out.print(" Enter price: ");
price[i] = sc.nextDouble();
System.out.print(" Enter quantity: ");
quan[i] = sc.nextInt();
total+=price[i]*quan[i]; //updates total
}
}
public void Menu() {
System.out.print("\n Item list:\n");
System.out.println(" Crayons\t120.00\n"
+ " Eraser 17.25\n"
+ " Folder 12.75\n"
+ " Glue\t\t59.75\n"
+ " Highlighter 36.75\n"
+ " Notebook\t44.75\n"
+ " Paper 50.00\n"
+ " Pen\t\t23.50\n"
+ " Pencil 12.00\n"
+ " Ruler 16.00\n"
+ " Scisscors 79.75\n");
}
public void Receipt() {
System.out.println("\n\n **********************************");
System.out.println("\t\tRECEIPT");
System.out.println(" **********************************");
System.out.println("\t We'll always be your");
System.out.println("\t Favorite Store");
System.out.println();
System.out.print(" S.No ");
System.out.print(" Quantity ");
System.out.print("Item name ");
System.out.print(" Price ");
System.out.println();
for (int i = 0; i < item; i++) {
System.out.print(" 100" + i + " ");
System.out.print(quan[i]+"\t " );
System.out.print(des[i] + "\t ");
System.out.print(price[i]+ " ");
System.out.println();
}
System.out.println("\n\tSubtotal: " + this.total);
double vat = (float) 0.12 * this.total;
System.out.println(" \t12% VAT: "+ vat);
double gtotal = (float) this.total + vat;
System.out.println(" \n\tAmount Due: " + gtotal);
System.out.print(" \tCash: ");
float cash = (float) sc.nextDouble();
double change = cash - gtotal;
System.out.println(" \tChange: " + change);
int qty = 0 ;
System.out.println("\n\t 12% VAT included");
for (int i = 0; i<item; i++) {
qty = qty + quan[i];
}
System.out.println(" \n\tQty purchased: " + qty);
System.out.println("\n This serves as your official receipt\n"
+ " Tell us about your experience\n"
+ " Send us feedback at bit.ly/fscaresph\n"
+ " Visit us also at www.favestore.com.ph\n"
+ " Thank you and please come again.");
System.out.println("\n ***********************************");
System.out.println("\n Customer Name: " + this.name);
System.out.println("\n Techy System Philippines, Inc."
+ "\n 7f Reyes Tower, Manila"
+ "\n VAT Reg TIN: 123-456-789-000"
+ "\n Date issued 03/10/2003"
+ "\n and Valid until 07/31/2025"
+ "\n THIS INVOICE/RECEIPT SHALL BE VALID"
+ "\n FIVE (5) YEARS FROM THE DATE OF "
+ "\n THE PERMIT TO USE");
}
}
public class Pajanconi {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int reply, total = 0;
double[] quan = null;
String name = "";
List obj = new List(name, total, args, total, quan);
do {
obj.Welcome();
obj.Menu();
obj.Input();
obj.Receipt();
System.out.println("\nPerform another transaction? yes (1) / no (2)");
reply = sc.nextInt();
if (reply == 2) {
System.out.println("\n\tThank you for using the system!");
}
} while (reply == 1);
sc.close();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

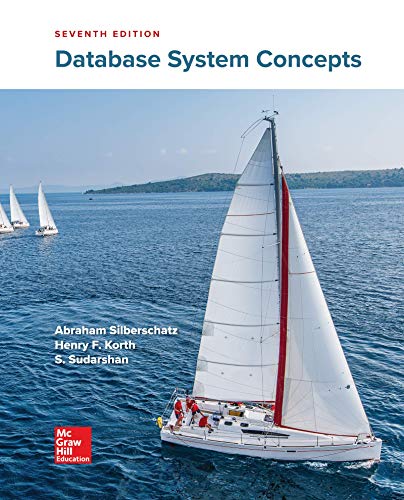
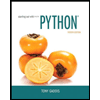
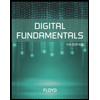
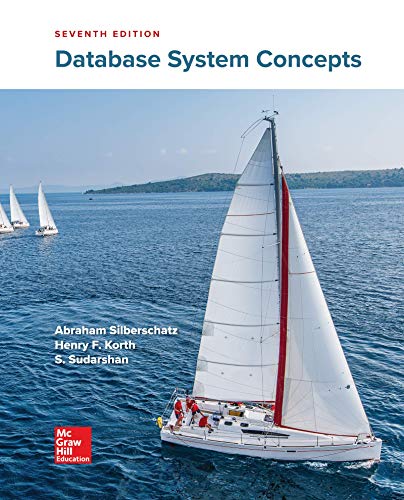
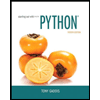
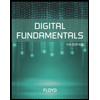
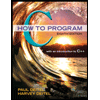
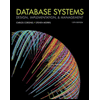
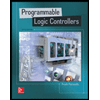