Can you help me fix my code because the total sum per item does not show in the receipt and when choosing to perform another transaction, the input for the customer's name and the number of items overlaps as seen in the image below. package cashier; import java.util.Scanner; class List { Scanner sc = new Scanner(System.in); private String name; private int item; private int[] des; private double price; private int[] quan; private double total; private double sum; public List(String name, int item, int[] des, double total, int[] quan, double sum) { this.name = name; this.item = item; this.des = des; this.quan = quan; this.total = total; this.sum = sum; } public void Input() { System.out.print("\n Enter name of customer: "); name = sc.nextLine(); System.out.print(" Enter number of items: "); item = sc.nextInt(); des = new int[item]; quan = new int[item]; total = 0; for (int i = 0; i < item; i++) { System.out.print("\n Enter item code: "); des[i] = sc.nextInt(); for (int j = 0; j < item; j++) { if (des[j]==1001) { price = 120.00; System.out.println(" 1001 Item name: Crayons Php 120.00\n"); } else if (des[j]==1002) { price = 17.25; System.out.println(" 1002 Item name: Eraser Php 17.25\n"); } else if (des[j]==1003) { System.out.println(" 1003 Item name: Folder Php 12.75"); } else if (des[j]==1004) { System.out.println(" 1004 Item name: Glue Php 59.75"); } else if (des[j]==1005) { System.out.println(" 1005 Item name: Highlighter Php 36.75"); } else if (des[j]==1006) { System.out.println(" 1006 Item name: Notebook Php 44.75"); } else if (des[j]==1007) { System.out.println(" 1007 Item name: Paper Php 50.00"); } else if (des[j]==1008) { System.out.println(" 1008 Item name: Pen Php 23.50"); } else if (des[j]==1009) { System.out.println(" 1009 Item name: Pencil Php 12.00"); } else if (des[j]==1010) { System.out.println(" 1010 Item name: Ruler Php 16.00"); } else if (des[j]==1011) { System.out.println(" 1011 Item name: Scissors Php 79.75"); } sum = price *quan[i]; } System.out.print(" Enter quantity: "); quan[i] = sc.nextInt(); total+=price*quan[i]; } } public void Receipt() { System.out.println("\n\n **********************************"); System.out.println("\t\tRECEIPT"); System.out.println(" **********************************"); System.out.println("\t We'll always be your"); System.out.println("\t Favorite Store"); System.out.println(); System.out.print(" S.No "); System.out.print("Item name "); System.out.print(" Quantity "); System.out.print(" Price "); System.out.println(); for (int i = 0; i < item; i++) { if (des[i]==1001) { System.out.println(" 1001 Crayons "+quan[i]+" "+sum); System.out.println(" 120.00"); } else if (des[i]==1002) { System.out.println(" 1002 Eraser "+quan[i]+" "+sum); System.out.println(" 17.25"); } else if (des[i]==1003) { System.out.println(" 1003 Folder "+quan[i]+" "+sum); System.out.println(" 12.75"); } else if (des[i]==1004) { System.out.println(" 1004 Glue "+quan[i]+" "+sum); System.out.println(" 59.75"); } else if (des[i]==1005) { System.out.println(" 1005 Highlighter "+quan[i]+" "+sum); System.out.println(" 36.75"); } else if (des[i]==1006) { System.out.println(" 1006 Notebook "+quan[i]+" "+sum); System.out.println(" 44.75"); } else if (des[i]==1007) { System.out.println(" 1007 Paper "+quan[i]+" "+sum); System.out.println(" 50.00"); } else if (des[i]==1008) { System.out.println(" 1008 Pen "+quan[i]+" "+sum); System.out.println(" 23.50"); } else if (des[i]==1009) { System.out.println(" 1009 Pencil "+quan[i]+" "+sum); System.out.println(" 12.00"); } else if (des[i]==1010) { System.out.println(" 1010 Ruler "+quan[i]+" "+sum); System.out.println(" 16.00"); } else if (des[i]==1011) { System.out.println(" 1011 Scissors "+quan[i]+" "+sum); System.out.println(" 79.75"); } } System.out.println(); System.out.println("\n\tSubtotal: " + this.total); double vat = (float) 0.12 * this.total; System.out.println(" \t12% VAT: "+ vat); double gtotal = (float) this.total + vat; System.out.println(" \n\tAmount Due: " + gtotal); System.out.print(" \tCash: "); float cash = (float) sc.nextDouble(); double change = cash - gtotal; System.out.println(" \tChange: " + change); int qty = 0 ; System.out.println("\n\t 12% VAT included"); for (int i = 0; i
Can you help me fix my code because the total sum per item does not show in the receipt and when choosing to perform another transaction, the input for the customer's name and the number of items overlaps as seen in the image below.
package cashier;
import java.util.Scanner;
class List {
Scanner sc = new Scanner(System.in);
private String name;
private int item;
private int[] des;
private double price;
private int[] quan;
private double total;
private double sum;
public List(String name, int item, int[] des, double total, int[] quan, double sum) {
this.name = name;
this.item = item;
this.des = des;
this.quan = quan;
this.total = total;
this.sum = sum;
}
public void Input() {
System.out.print("\n Enter name of customer: ");
name = sc.nextLine();
System.out.print(" Enter number of items: ");
item = sc.nextInt();
des = new int[item];
quan = new int[item];
total = 0;
for (int i = 0; i < item; i++) {
System.out.print("\n Enter item code: ");
des[i] = sc.nextInt();
for (int j = 0; j < item; j++) {
if (des[j]==1001) {
price = 120.00;
System.out.println(" 1001 Item name: Crayons Php 120.00\n");
}
else if (des[j]==1002) {
price = 17.25;
System.out.println(" 1002 Item name: Eraser Php 17.25\n");
}
else if (des[j]==1003) {
System.out.println(" 1003 Item name: Folder Php 12.75");
}
else if (des[j]==1004) {
System.out.println(" 1004 Item name: Glue Php 59.75");
}
else if (des[j]==1005) {
System.out.println(" 1005 Item name: Highlighter Php 36.75");
}
else if (des[j]==1006) {
System.out.println(" 1006 Item name: Notebook Php 44.75");
}
else if (des[j]==1007) {
System.out.println(" 1007 Item name: Paper Php 50.00");
}
else if (des[j]==1008) {
System.out.println(" 1008 Item name: Pen Php 23.50");
}
else if (des[j]==1009) {
System.out.println(" 1009 Item name: Pencil Php 12.00");
}
else if (des[j]==1010) {
System.out.println(" 1010 Item name: Ruler Php 16.00");
}
else if (des[j]==1011) {
System.out.println(" 1011 Item name: Scissors Php 79.75");
}
sum = price *quan[i];
}
System.out.print(" Enter quantity: ");
quan[i] = sc.nextInt();
total+=price*quan[i];
}
}
public void Receipt() {
System.out.println("\n\n **********************************");
System.out.println("\t\tRECEIPT");
System.out.println(" **********************************");
System.out.println("\t We'll always be your");
System.out.println("\t Favorite Store");
System.out.println();
System.out.print(" S.No ");
System.out.print("Item name ");
System.out.print(" Quantity ");
System.out.print(" Price ");
System.out.println();
for (int i = 0; i < item; i++) {
if (des[i]==1001) {
System.out.println(" 1001 Crayons "+quan[i]+" "+sum);
System.out.println(" 120.00");
}
else if (des[i]==1002) {
System.out.println(" 1002 Eraser "+quan[i]+" "+sum);
System.out.println(" 17.25");
}
else if (des[i]==1003) {
System.out.println(" 1003 Folder "+quan[i]+" "+sum);
System.out.println(" 12.75");
}
else if (des[i]==1004) {
System.out.println(" 1004 Glue "+quan[i]+" "+sum);
System.out.println(" 59.75");
}
else if (des[i]==1005) {
System.out.println(" 1005 Highlighter "+quan[i]+" "+sum);
System.out.println(" 36.75");
}
else if (des[i]==1006) {
System.out.println(" 1006 Notebook "+quan[i]+" "+sum);
System.out.println(" 44.75");
}
else if (des[i]==1007) {
System.out.println(" 1007 Paper "+quan[i]+" "+sum);
System.out.println(" 50.00");
}
else if (des[i]==1008) {
System.out.println(" 1008 Pen "+quan[i]+" "+sum);
System.out.println(" 23.50");
}
else if (des[i]==1009) {
System.out.println(" 1009 Pencil "+quan[i]+" "+sum);
System.out.println(" 12.00");
}
else if (des[i]==1010) {
System.out.println(" 1010 Ruler "+quan[i]+" "+sum);
System.out.println(" 16.00");
}
else if (des[i]==1011) {
System.out.println(" 1011 Scissors "+quan[i]+" "+sum);
System.out.println(" 79.75");
}
}
System.out.println();
System.out.println("\n\tSubtotal: " + this.total);
double vat = (float) 0.12 * this.total;
System.out.println(" \t12% VAT: "+ vat);
double gtotal = (float) this.total + vat;
System.out.println(" \n\tAmount Due: " + gtotal);
System.out.print(" \tCash: ");
float cash = (float) sc.nextDouble();
double change = cash - gtotal;
System.out.println(" \tChange: " + change);
int qty = 0 ;
System.out.println("\n\t 12% VAT included");
for (int i = 0; i<item; i++) {
qty = qty + quan[i];
}
}
}
public class Cashier {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int reply, total = 0;
int[] quan = null;
String name = "";
int[] des = null;
double sum = 0;
List obj = new List(name, total, des, total, quan, sum);
do {
System.out.println(" \n\tWelcome to Favorite Store"
+ "\n\t Philippines\n"
+ "\n VAT Reg TIN: 123-456-789-000");
obj.Input();
obj.Receipt();
System.out.println("\nPerform another transaction? yes (1) / no (2)");
reply = sc.nextInt();
if (reply == 2) {
System.out.println("\n\tThank you for using the system!");
}
} while (reply == 1);
sc.close();
}
}
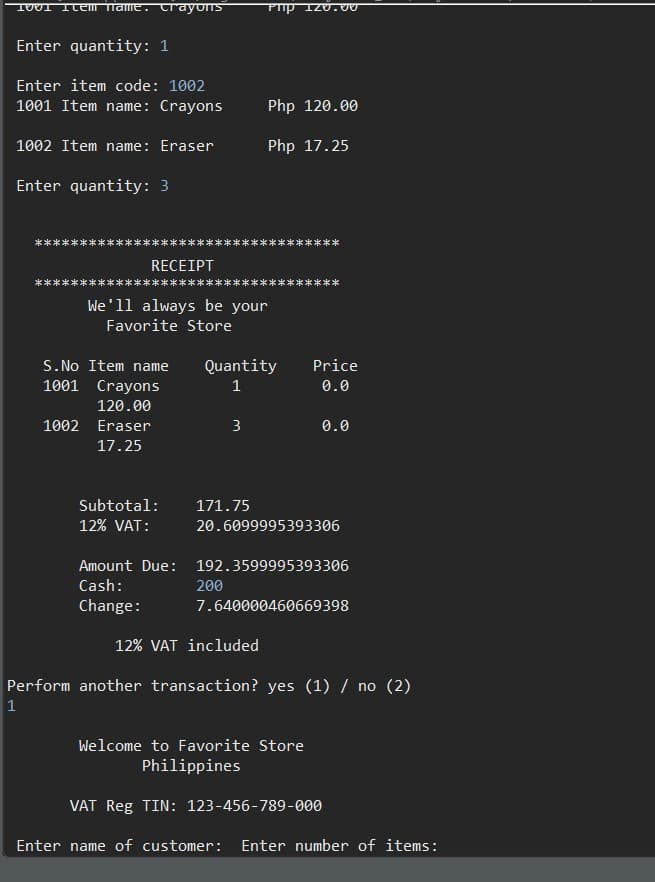
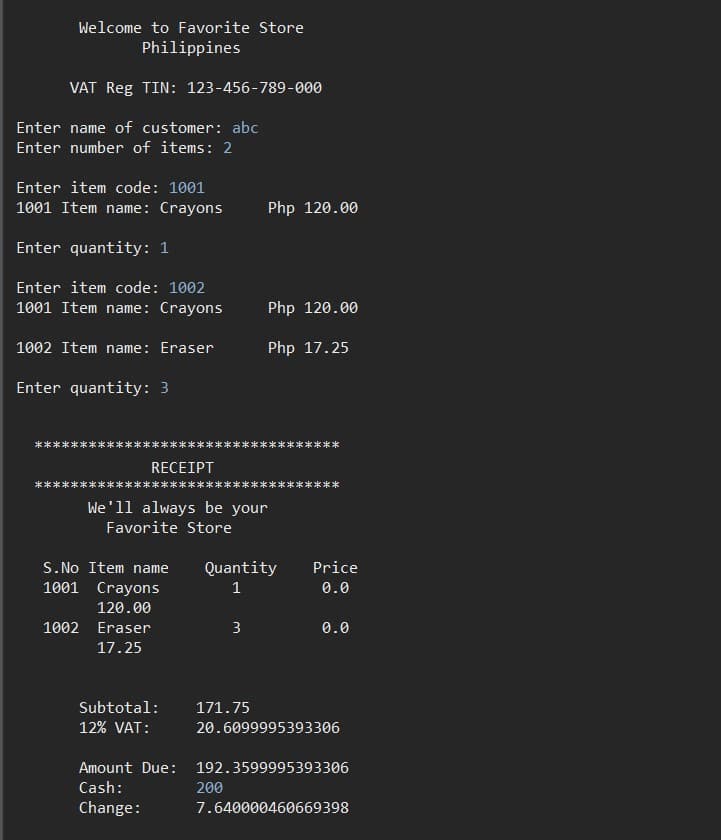

Step by step
Solved in 3 steps with 2 images
