What change do i make to fix my code so it will not output negative numbers for the future years. Here is the code. and output. HOW CAN I FIX THE ARITHMETIC OVERFLOW? and here is the question. If P is the population of the first day of the year, B is the birth rate and D is the death rate, the estimated population at the end of the next year is given by formula: P + ( B * P ) / 100 – ( D * P ) / 100 The population growth rate is given by the formula: B - D Write a program that prompts the user to enter the starting population, birth and death rates and the number of years to project. The program should then calculate and print the growth rate and the estimated population after each year. Your program must contain the following functions: GrowthRate: This function takes as its parameters the birth and death rates and returns the population growth rate. Estimated Population: This function takes as its parameters the current population, the birth rate and the death rate. It returns the estimated population for the next year. Your program should not accept negative birth rate, negative death rate or population of less than 2.
What change do i make to fix my code so it will not output negative numbers for the future years. Here is the code. and output. HOW CAN I FIX THE ARITHMETIC OVERFLOW?
and here is the question.
If P is the population of the first day of the year, B is the birth rate and D is the death rate, the estimated population at the end of the next year is given by formula:
P + ( B * P ) / 100 – ( D * P ) / 100
The population growth rate is given by the formula: B - D
Write a
GrowthRate: This function takes as its parameters the birth and death rates and returns the population growth rate.
Estimated Population: This function takes as its parameters the current population, the birth rate and the death rate. It returns the estimated population for the next year. Your program should not accept negative birth rate, negative death rate or population of less than 2.



Step by step
Solved in 3 steps with 1 images

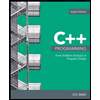
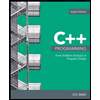