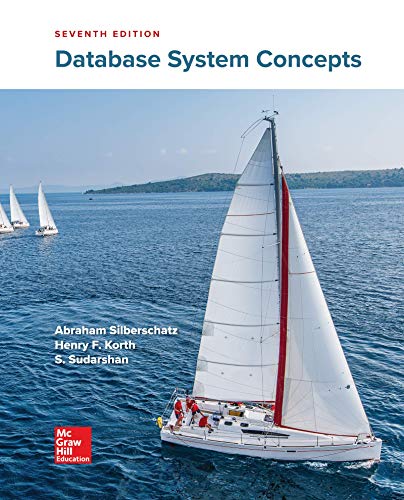
Concept explainers
// Add to this partially built code.
// fill in code where there is a TODO
#include<iostream>
using namespace std;
int main()
{
cout.setf(ios::fixed);
cout.setf(ios::showpoint); // show decimals even if not needed
cout.precision(2); // two places to the right of the decimal
// TODO: enter the missing types below
int time;
float ticketPrice;
destination; //'C'=Chicago, 'P'=Portland, 'M'=Miami
typeOfDay; //'D'=weekDay 'E'=weekEnd
cout << "Welcome to Airlines!" << endl;
cout << "What is your destination? ([C]hicago, [M]iami, [P]ortland) ";
cin >> destination;
cout << "What time will you travel? (Enter time between 0-2359) ";
cin >> time;
// TODO: set isDayTime to true if time 5AM or later, but before 7PM
cout << "What type of day are you traveling? (week[E]nd or week[D]ay) ";
cin >> typeOfDay;
// TODO: set isWeekend to true if typeOfDay is 'E', otherwise false
// Depending upon the destination, and whether it is weekend, day/night
// set the appropriate price
// I recommend using a switch
// I am providing much of the Input and Output dialog to simplify this program
cout << "Each ticket will cost: $" << ticketPrice << endl;
int numTickets;
cout << "How many tickets do you want? ";
cin >> numTickets;
// TODO: calculate the totalCost based on the number of tickets and ticketPrice, then print it
cout << "You owe: $" << totalCost << endl;
// TODO: declare a variable that will hold the user's payment
// prompt the user for "Amount paid? "
// read in the user's Payment
// TODO: calculate the change (a float)
// TODO: If the user's payment is too little, then print this message:
cout << "That is too little! No tickets ordered.";
// otherwise print these 2 lines:
cout << "You will get in change: $" << change << endl;
cout << "Your tickets have been ordered!";
return 0;
}
![// Add to this partially built code
// fill in code where there is a TODO
#include<iostream>
using namespace std;
change (a float)
// TODO: calculate the
int main()
cout.setf(ios:fixed):
cout.setf(ios:showpoint); // show decimals even if not needed
cout.precision(2); // two places to the right of the decimal
// TODO: enter the missing types below
int time;
float ticketPrice;
destination; //'c'=Chicago, 'P'=Portland, 'M'=Miami
typeOfDay; //'D'=weekDay 'E'=weekEnd
// TODO: If the user's payment is too little, then print this message:
cout << "Welcome to Airlines!"
<< endl;
cout <« "What is your destination? ([C]hicago, [M]iami, [P]ortland) ";
cin >> destination;
cout << "What time will you travel? (Enter time between 0-2359) ";
cin >> time;
cout << "That is too little! No tickets ordered.";
// TODO: set isDayTime to true if time 5AM or later, but before
7PM
cout << "What type of day are you traveling? (week[E]nd or week[D]ay) ";
cin >> typeOfDay;
// TODO: set isWeekend to true if typeOfDay is 'E', otherwise false
// otherwise print these 2 lines:
// Depending upon the destination, and whether it is weekend, day/night
// set the appropriate price
//I recommend using a switch
// I am providing much of the Input and Output dialog to simplify this program
cout << "Each ticket will cost: Ş" << ticketPrice << endl;
cout << "You will get in change: $" << change << endl;
int numTickets;
cout << "How many tickets do you want? ";
cout << "Your tickets have been ordered!";
cin >> num Tickets;
// TODO: calculate the totalCost based on the number of tickets and ticketPrice, then print it
cout << "You owe: $" << totalCost << endl;
return 0;
// TODO: declare a variable that will hold the user's payment
// prompt the user for "Amount paid?"
// read in the user's Payment](https://content.bartleby.com/qna-images/question/6900813d-7c23-46b7-a2db-4b3d463f1913/2797c049-729f-4d11-9ca1-fb4f01afd10b/e42du8h.jpeg)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- C++ Coding Help (Operators: new delete) Assign pointer myGas with a new Gas object. Call myGas's Read() to read the object's fields. Then, call myGas's Print() to output the values of the fields. Finally, delete myGas. Ex: If the input is 14 45, then the output is: Gas's volume: 14 Gas's temperature: 45 Gas with volume 14 and temperature 45 is deallocated. #include <iostream>using namespace std; class Gas { public: Gas(); void Read(); void Print(); ~Gas(); private: int volume; int temperature;};Gas::Gas() { volume = 0; temperature = 0;}void Gas::Read() { cin >> volume; cin >> temperature;} void Gas::Print() { cout << "Gas's volume: " << volume << endl; cout << "Gas's temperature: " << temperature << endl;} Gas::~Gas() { // Covered in section on Destructors. cout << "Gas with volume " << volume << " and temperature " << temperature << " is deallocated."…arrow_forwardint x1 = 66; int y1 = 39; int d; _asm { } mov EAX, X1; mov EBX, y1; push EAX; push EBX; pop ECX mov d, ECX; What is d in decimal format?arrow_forwardC++ Double Pointer: Can you draw picture of what this means : Food **table? struct Food { int expiration_date; string brand; } Food **table; What is this double pointer saying? I know its a 2D matrix but I don't understand what I'm dealing with here. Does the statement mean that we have an array of pointers of type Food and what is the other * mean? I'm confused.arrow_forward
- #include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> class A { public: T func(T a, T b){ return a/b; } }; int main(int argc, char const *argv[]) { A <float>a1; cout<<a1.func(3,2)<<endl; cout<<a1.func(3.0,2.0)<<endl; return 0; } Give output for this code.arrow_forward#include <iostream>using namespace std; struct Person{ int weight ; int height;}; int main(){ Person fred; Person* ptr = &fred; fred.weight=190; fred.height=70; return 0;} What is a correct way to subtract 5 from fred's weight using the pointer variable 'ptr'?arrow_forward#include <iostream> #include <iomanip> #include <string> #include <vector> using namespace std; class Movie { private: string title = ""; int year = 0; public: void set_title(string title_param); string get_title() const; // "const" safeguards class variable changes within function string get_title_upper() const; void set_year(int year_param); int get_year() const; }; // NOTICE: Class declaration ends with semicolon! void Movie::set_title(string title_param) { title = title_param; } string Movie::get_title() const { return title; } string Movie::get_title_upper() const { string title_upper; for (char c : title) { title_upper.push_back(toupper(c)); } return title_upper; } void Movie::set_year(int year_param) { year = year_param; } int Movie::get_year() const { return year; } int main() { cout << "The Movie List program\n\n"…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
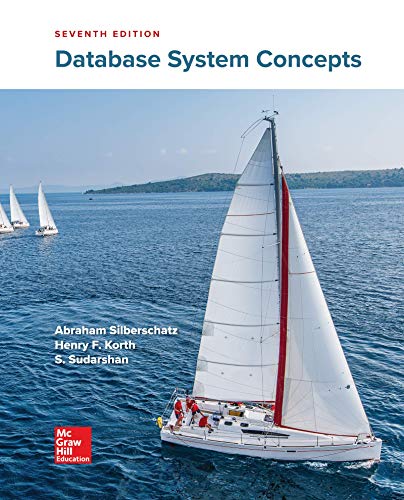
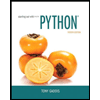
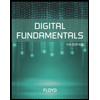
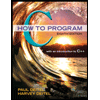
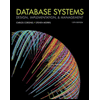
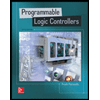