what seems to be wrong with this C program. Please debug it for me. Copy-paste the new source code then provide a screenshot that yours is working here is the code: #include int main() { //prompting user for non negative integer printf("Enter a non-negative integer number: "); //reading number int n;//to store number scanf("%d",&n);//reading integer //now finding integer is prime or not //note: a number is prime it is divisible by 1 and the number itself int flag=1;//initially assuming n is prime, 1:prime, 0:not prime //now checking if any other number other than 1 and n, divides n, then it is not prime for(int i=2;i
what seems to be wrong with this C program. Please debug it for me. Copy-paste the new source code then provide a screenshot that yours is working
here is the code:
#include <stdio.h>
int main()
{
//prompting user for non negative integer
printf("Enter a non-negative integer number: ");
//reading number
int n;//to store number
scanf("%d",&n);//reading integer
//now finding integer is prime or not
//note: a number is prime it is divisible by 1 and the number itself
int flag=1;//initially assuming n is prime, 1:prime, 0:not prime
//now checking if any other number other than 1 and n, divides n, then it is not prime
for(int i=2;i<n;i++)//i is from i=2 to n-1
if(n%i==0)//if such i found, (i divides n)
{//then n is to prime, since we found i that divides n, where i is not 1 and n
flag=0;//setting flag to 0, indicates it is not prime
break;//breaking loop
}
//displaying output
if(flag==1)//flag 1 means n is prime
{
printf("%d is a prime number\n",n);
}
else{//flag=0 means n is not prime
printf("%d is a non-prime number\n",n);
}
return 0;
}
output:
![C:\Users\Pluto\Documents\mp6\CCG-MP7.c - Dev-C++ 5.11
File
Edit
Search View Project Execute Tools AStyle Window Help
目唱
品口国盟
TDM-GCC 4.9.2 64-bit Release
(globals)
Project Classes Debug
CCG-MP6.c CCG-MP7.c
1
#include <stdio.h>
2
int main()
3E {
//prompting user for non negative integer
printf("Enter a non-negative integer number: ");
//reading number
int n;//to store number
scanf("%d",&n);//reading integer
4
6
7
9
//now finding integer is prime or not
//note: a number is prime it is divisible by 1 and the number itself
int flag=1;//initially assuming n is prime, 1:prime, 0:not prime
//now checking if any other number other than 1 and n, divides n, then it is not prime
for (int i=2;i<n;i++)//i is from i=2 to n-1
if(n%i==0)//if such i found, (i divides n)
10
11
12
13
14
15
16 E
{//then n is to prime, since we found i that divides n, where i is not 1 and n
flag=0;//setting flag to 0, indicates it is not prime
break;//breaking loop
17
18
19
//displaying output
if(flag==1)//flag 1 means n is prime
{
printf("%d is a prime number\n",n);
}
else{//flag=0 means n is not prime
printf("%d is a non-prime number\n",n);
}
return e;
20
21
22 E
23
24
25 =
26
27
28
29
}
30
31
output:
88 Compiler (5)
Resources dih Compile Log Debug a Find Results Close
Line
Col
File
Message
C:\Users\Pluto\Documents\mp6\CCG-MP7.c
C:\Users\Pluto\Documents\mp6\CCG-MP7.c
C:\Users\Pluto\Documents\mp6\CCG-MP7.c
In function 'main':
[Error] 'for' loop initial declarations are only allowed in C99 or C11 mode
[Note] use option -std=c99, -std=gnu99, -std=c11 or -std3gnu11 to compile your code
14
14
5
C:\Users\Pluto\Documents\mp6\CCG-MP7.c
C:\Users\Pluto\Documents\mp6\CCG-MP7.c
At top level:
31
[Error] expected '=', ',',|
", 'asm' or '_attribute_' before ':' token
Line:
20
Col: 24
Sel: 0
Lines: 31
Length: 1078
Insert
Done parsing in 0.016 seconds
8:41 pm
IN
O Type here to search
DEV
! 21°C
O 4»)) A ENG
PC
30/01/2022](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F16c2a176-7258-426d-a8e7-141f3bb3e8d7%2F1ae522a9-935b-4c75-b5c1-c7bc5debfd13%2Fnuaq50r_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps with 2 images

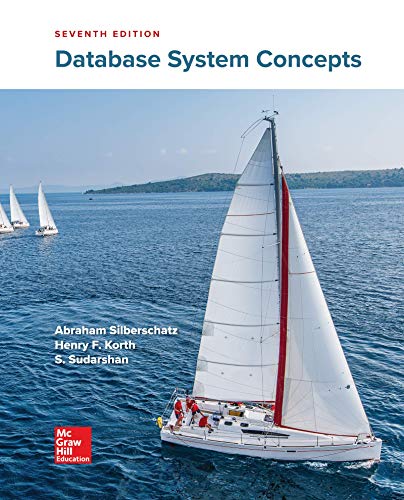
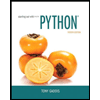
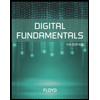
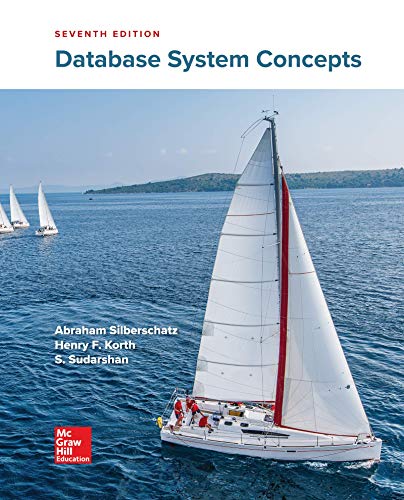
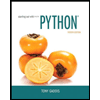
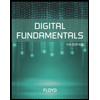
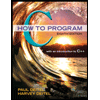
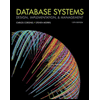
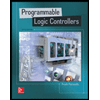