What students will learn: . The purpose of repetition structures • Three kinds of loops that are found in most languages Knowing when to use a specific kind of loop How to apply repetition to solve problems Overview: If there's one thing computers are good at, it's repeating something over and over. The concept of repetition (which some call iteration" and others "looping") is not terribly difficult since we humans repeat things in our daily lives. Any decent programming language is going to support iteration and usually allows for three different kinds of "looping templates. These templates are exactly what this lab is going to cover. The three kinds of loops we'll cover are the for, while and do-while loop. You want to memorize the templates for these. Before that, it's important to know when to use them. Here's an overall guideline to help you out: 1. Use a for loop when you want to repeat something a certain number of times. For example, if you want to repeat something 100 times, and a for loop is a good candidate for that. Or, if you wanted to count from 50 to 3000 in increments of 10, you could do that too. 2. Use a while loop is useful when you don't know how many times something will repeat; the loop could "go on forever". As an example, if you ask a user to enter a number between 1- 10 and they consistently enter 45, this could go on forever. Eventually (and hopefully), the user would enter a valid number. 3. Use a do-while loop when the loop must execute at least one time. The loops above can execute 0 times, but not this one! The reason is because, for all loops, there is a test to see if the loop should continue repeating. With a do-while loop, that test is at the bottom. The best way to show these loops is through examples. The code below sums the numbers from 1 to 100. You should see that all loops have a 1) test to continue, and 2) make progress towards completing. int sum - Op for (int i = 1; i <= 100; i++) { sum + 17 int sum= 0; int i = 1; while (i <100) sum int sun- 0; int i = 1: do ( sum } while(i <=100); • The for loop starts a counter (called T) at 1. So long as i is<= 100, the loop continues. We then execute sum += i. Finally, i++ occurs and the test to continue is evaluated again. • The while and do-while loops are nearly identical. However, notice that the do-while has the test to continue at the bottom- and also has a semicolon at the end. Be careful! Remember, the class name should be Lab6A The user input is indicated in bold. Sample output: Please enter 10 numbers and this program will display the largest. Please enter number 1: 50 Please enter number 2: 51 Please enter number 3: 10 Please enter number 4: 1 Please enter number 5: 99 Please enter number 6: 1000 Please enter number 7: 1010 Please enter number 8: 42 Please enter number 9: 89 Please enter number 10: 1000 The largest number was 1010 Intentionally Left Empty, please proceed to Next Page
What students will learn: . The purpose of repetition structures • Three kinds of loops that are found in most languages Knowing when to use a specific kind of loop How to apply repetition to solve problems Overview: If there's one thing computers are good at, it's repeating something over and over. The concept of repetition (which some call iteration" and others "looping") is not terribly difficult since we humans repeat things in our daily lives. Any decent programming language is going to support iteration and usually allows for three different kinds of "looping templates. These templates are exactly what this lab is going to cover. The three kinds of loops we'll cover are the for, while and do-while loop. You want to memorize the templates for these. Before that, it's important to know when to use them. Here's an overall guideline to help you out: 1. Use a for loop when you want to repeat something a certain number of times. For example, if you want to repeat something 100 times, and a for loop is a good candidate for that. Or, if you wanted to count from 50 to 3000 in increments of 10, you could do that too. 2. Use a while loop is useful when you don't know how many times something will repeat; the loop could "go on forever". As an example, if you ask a user to enter a number between 1- 10 and they consistently enter 45, this could go on forever. Eventually (and hopefully), the user would enter a valid number. 3. Use a do-while loop when the loop must execute at least one time. The loops above can execute 0 times, but not this one! The reason is because, for all loops, there is a test to see if the loop should continue repeating. With a do-while loop, that test is at the bottom. The best way to show these loops is through examples. The code below sums the numbers from 1 to 100. You should see that all loops have a 1) test to continue, and 2) make progress towards completing. int sum - Op for (int i = 1; i <= 100; i++) { sum + 17 int sum= 0; int i = 1; while (i <100) sum int sun- 0; int i = 1: do ( sum } while(i <=100); • The for loop starts a counter (called T) at 1. So long as i is<= 100, the loop continues. We then execute sum += i. Finally, i++ occurs and the test to continue is evaluated again. • The while and do-while loops are nearly identical. However, notice that the do-while has the test to continue at the bottom- and also has a semicolon at the end. Be careful! Remember, the class name should be Lab6A The user input is indicated in bold. Sample output: Please enter 10 numbers and this program will display the largest. Please enter number 1: 50 Please enter number 2: 51 Please enter number 3: 10 Please enter number 4: 1 Please enter number 5: 99 Please enter number 6: 1000 Please enter number 7: 1010 Please enter number 8: 42 Please enter number 9: 89 Please enter number 10: 1000 The largest number was 1010 Intentionally Left Empty, please proceed to Next Page
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
provide java code
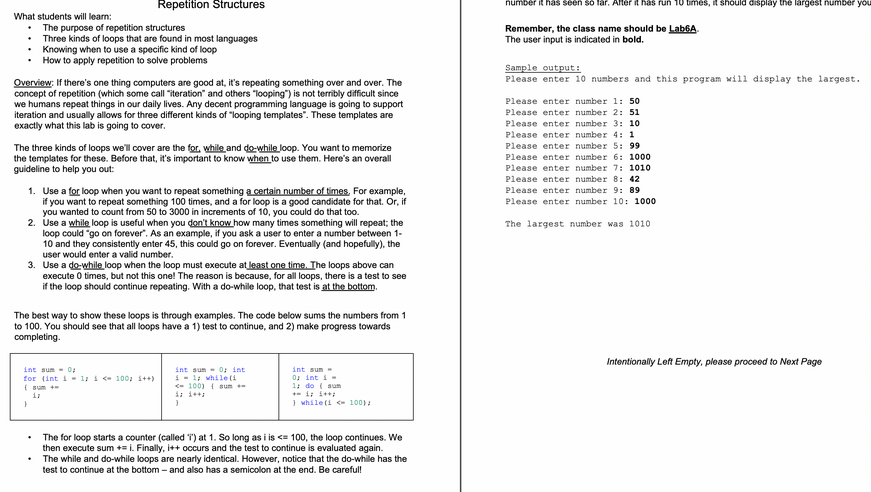
Transcribed Image Text:What students will learn:
The purpose of repetition structures
• Three kinds of loops that are found in most languages
• Knowing when to use a specific kind of loop
• How to apply repetition to solve problems
Overview: If there's one thing computers are good at, it's repeating something over and over. The
concept of repetition (which some call "iteration" and others "looping") is not terribly difficult since
we humans repeat things in our daily lives. Any decent programming language is going to support
iteration and usually allows for three different kinds of "looping templates". These templates are
exactly what this lab is going to cover.
Repetition Structures
The three kinds of loops we'll cover are the for, while and do-while loop. You want to memorize
the templates for these. Before that, it's important to know when to use them. Here's an overall
guideline to help you out:
1. Use a for loop when you want to repeat something a certain number of times. For example,
if you want to repeat something 100 times, and a for loop is a good candidate for that. Or, if
you wanted to count from 50 to 3000 in increments of 10, you could do that too.
2. Use a while loop is useful when you don't know how many times something will repeat; the
loop could "go on forever". As an example, if you ask a user to enter a number between 1-
10 and they consistently enter 45, this could go on forever. Eventually (and hopefully), the
user would enter a valid number.
3. Use a do-while loop when the loop must execute at least one time. The loops above can
execute 0 times, but not this one! The reason is because, for all loops, there is a test to see
if the loop should continue repeating. With a do-while loop, that test is at the bottom.
The best way to show these loops is through examples. The code below sums the numbers from 1
to 100. You should see that all loops have a 1) test to continue, and 2) make progress towards
completing.
int sum = 0;
for (int i = 1; i <= 100; i++)
}
17
int sum= 0; int
i 1; while (i
<100) sum
}
int sum-
0; int i =
1; do sum
+= 1; i++;
} while (i < 100);
The for loop starts a counter (called ") at 1. So long as i is <= 100, the loop continues. We
then execute sum += i. Finally, i++ occurs and the test to continue is evaluated again.
The while and do-while loops are nearly identical. However, notice that the do-while has the
test to continue at the bottom - and also has a semicolon at the end. Be careful!
number it has seen so far. After it has run 10 times, it should display the largest number you
Remember, the class name should be Lab6A
The user input is indicated in bold.
Sample output:
Please enter 10 numbers and this program will display the largest.
Please enter number 1: 50
Please enter number 2: 51
Please enter number 3: 10
Please enter number 4: 1
Please enter number 5: 99
Please enter number 6: 1000
Please enter number 7: 1010
Please enter number 8: 42
Please enter number 9: 89
Please enter number 10: 1000
The largest number was 1010
Intentionally Left Empty, please proceed to Next Page
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
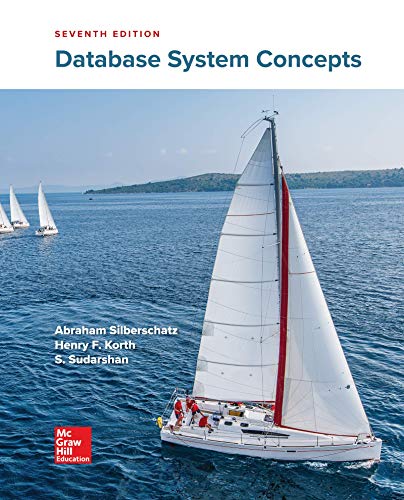
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
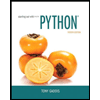
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
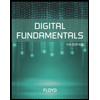
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
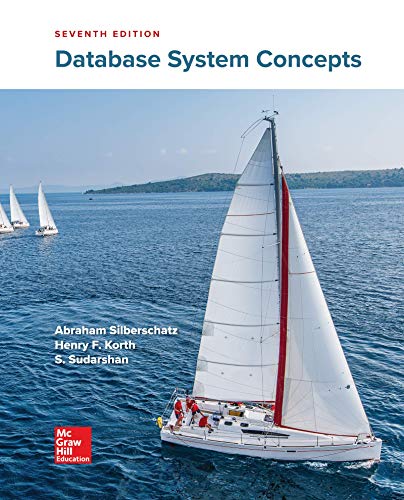
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
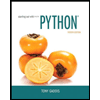
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
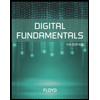
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
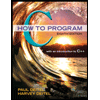
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
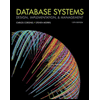
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
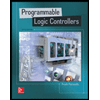
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education