when people clicks the Login button, your app should be able to check whether user has entered the right email and password combination. For now, please check against email admin@example.com and password "1234". If user enters the wrong combination, app should display an error message on a TextView as shown in the second screenshot above. Please note that on the click of the Login button, the keyboard should be dismissed if the combination is not correct. Please use the following code for dismissing the keyboard: nputMethodManager imm = (InputMethod Manager) getSystemService(Activity.INPUT_METHOD_SERVICE); mm.toggleSoftInput(Input Method Manager.HIDE IMPLICIT_ONLY, 0); of the email and password combination is correct, your app should move to a new Activity which should use a customized Toolbar. Please create a toolbar in a separate XML file using . The toolbar should contain at least 3 components, TextView for name, an ImageView in a circular form for an image and a TextView for points. For now, you can hardcode the values and the image on the toolbar. Your customized toolbar should look something like005 Add the custom toolbar to the Second Activity which will be shown on the successful login from the First Activity. The Second Activity should also contain a ListView which has each row customized to show a TextView for serial number, an ImageView for a circular image, a TextView for name and a TextView for score. You are required to create a separate XML file for row layout of the ListView. For now, please hardcode 10 items for each serial number, images, names and scores in Java arrays. Create your own Adapter class that extends from the BaseAdapter class that helps in inflating the row layout into the List View. Please note that the screenshot above shows only 7 rows, however, your ListView should have at least 10 rows. f your ListView is not showing the last row, please use the following code in your ListView in XML file: android:paddingBottom="100dp"
this is a login page of a android studio app
activity_main.xml -------------
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
tools:layout_editor_absoluteX="-10dp"
tools:layout_editor_absoluteY="-74dp">
<ImageView
android:id="@+id/imageView2"
android:layout_width="140dp"
android:layout_height="100dp"
android:contentDescription="TODO"
android:src="@drawable/ccc"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.542"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.35" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginEnd="48dp"
android:text="Email"
android:textColor="@color/black"
android:textSize="16sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toStartOf="@+id/editTextTextEmailAddress"
app:layout_constraintHorizontal_bias="0.881"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.517" />
<EditText
android:id="@+id/editTextTextEmailAddress"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10"
android:hint="some@exa.com"
android:inputType="textEmailAddress"
android:minHeight="48dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.726"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.499" />
<EditText
android:id="@+id/editTextTextPassword2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10"
android:hint="password"
android:inputType="textPassword"
android:minHeight="48dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.726"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.6" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password"
android:textColor="@color/black"
android:textSize="16sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toStartOf="@+id/editTextTextPassword2"
app:layout_constraintHorizontal_bias="0.702"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.598" />
<Button
android:id="@+id/button2"
android:layout_width="193dp"
android:layout_height="48dp"
android:background="#FF0000"
android:text="Login"
android:textColor="#FFFFFF"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.669"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.69"
tools:ignore="TextContrastCheck" />
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Fill your content"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.956" />
</androidx.constraintlayout.widget.ConstraintLayout>
mainactivity.class
package com.example.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
--so the question is in the picture
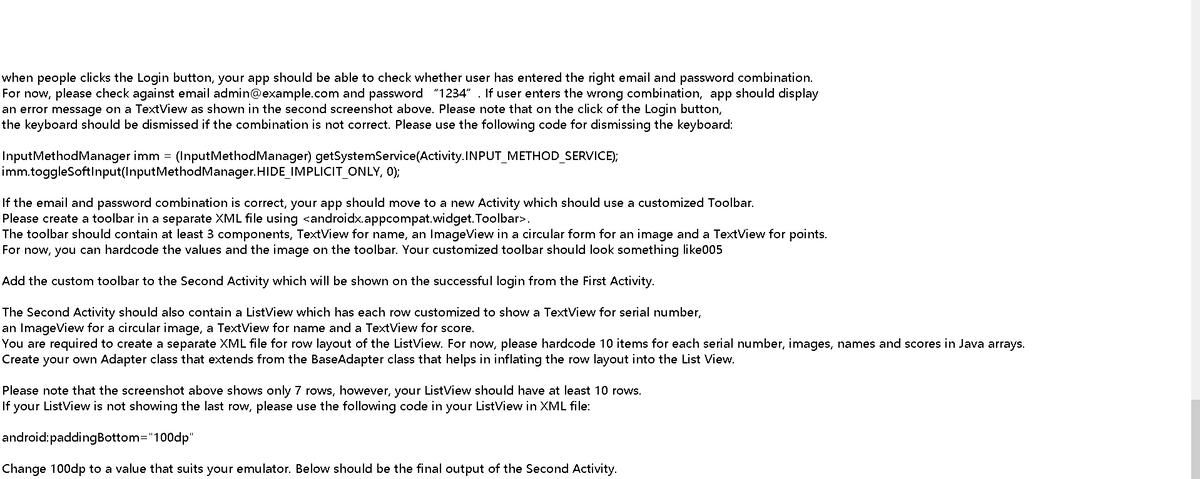
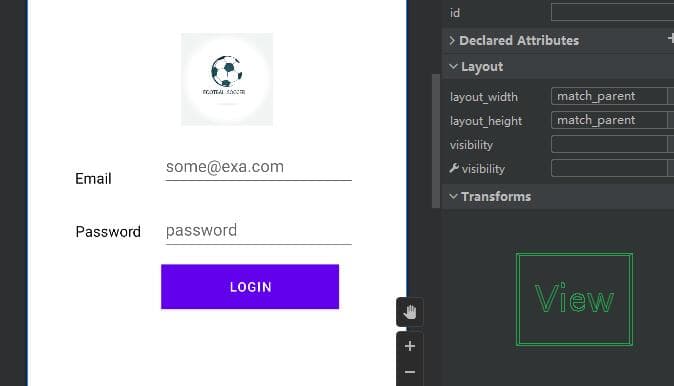

Step by step
Solved in 3 steps

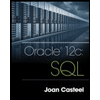
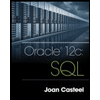