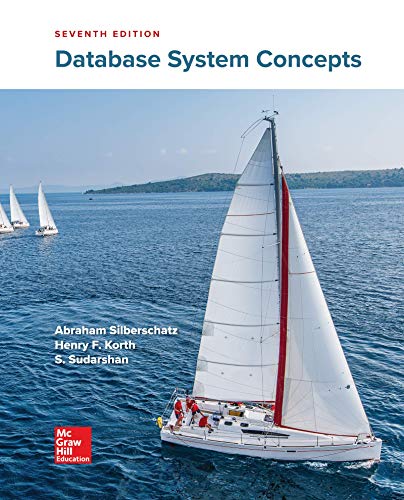
Concept explainers
Please fix this error. Using react.js. Also there will be submit button, after click submut button then will back, edit, and delete button. Show screenshot
Using react.js, create a inventory page where screen will display Inventory: Name Storage Type Max Item Capacity Resources: Resource Name Resource Type Max Number of Resources Current Number of Resources
body {
padding: 30px 20%;
}
h1 {
font-size: 2em;
text-align: center;
}
<div id ="app"></div>
class ContactForm extends React.Component{
constructor(props) {
super(props);
this.state = {
name: '',
email: '',
};
this.handleInputChange = this.handleInputChange.bind(this);
this.handleSubmit = this.handleSubmit.bind(this);
}
handleInputChange(event){
const target = event.target;
const value = target.type === 'checkbox'? target.checked : target.value;
const name = target.name;
this.setState({
[name]: value
})
console.log('Change detected. State updated' + name + ' = ' + value);
}
handleSubmit(event){
alert('A form was submitted: ' + this.state.name + ' // ' + this.state.email);
event.preventDefault();
}
render() {
return (
<div>
<form onSubmit={this.handleSubmit}>
<div className="form-group">
<h1> Inventory </h1>
<label for="nameInput">Name</label>
<input type="text" name="name" value= {this.state.name} onChange ={this.handleChange}
className="form-control" id="nameInput" placeholder="Name" />
</div>
<div className="form-group">
<label for="emailInput">Storage Type</label>
<input name="email" type="email" value={this.state.email} onChange={this.handleChange}
className="form-control" id="emailInput" placeholder="Stroage Type" />
</div>
<div className="form-group">
<label for="nameInput">Max Item Capacity</label>
<input type="text" name="email" value={this.state.name} onChange={this.handleChange}
className="form-control" id="nameInput" placeholder="Max Item Capacity" />
</div>
<div className="form-group">
<h1> Resources </h1>
<label for="nameInput">Resource Name</label>
<input type="text" name="name" value={this.state.name} onChange={this.handleChange}
className="form-control" id="nameInput" placeholder="Resource Name" />
</div>
<div className="form-group">
<label for="nameInput">Max Number of Resources</label>
<input name="text" name="email" value={this.state.name} onChange={this.handleChange}
className="form-control" id="nameInput" placeholder="Max Number of Resources" />
</div>
<div className="form-group">
<label for="nameInput">Current Number of Resources</label>
<input type="text" name="name" value={this.state.name} onChange={this.handleChange}
className="form-control" id="nameInput" placeholder="Current number of Resources" />
</div>
</form>
</div>
)
}
}
class MainTitle extends React.Component {
render(): React.ReactNode {
return(
<h1> Add Inventory </h1>
)
}
}
class App extends React.Component{
render(){
return(
<div>
<MainTitle/>
<ContactForm/>
</div>
)
}
}

Step by stepSolved in 3 steps with 2 images

- First Column: Merge column 1, column 2 and column 3. Set the background color as "blue" and align the contents to the right. Insert a Search Form. Insert a suitable input type to select items like SUBJECTS, COURSES, CV HELP and JOB MARKET. Set any name for the form field. Insert a suitable input type to enter the search text. Set any name for the text field and the maximum length is 40. Insert a submit button with a value SEARCH COURSES. • Set a form validation using JAVASCRIPT. If the form field (search) is empty. display an alert message and return false to prevent the form from being submitted.arrow_forwardHTML/CSS JAVASCRIPT please help me answer this question I will give you a good rating Thank you! Given the following word search application, which searches for words in paragraphs, modify it so that it Adds two spans tab within the HTML within the designated new "Illustate" text segments as shown below. Has a new click event which searches the occurrence of the word within all span tabs residing within the first div container , then highlights the text in the span tab which contains the word c) In the same routine, count the number of times ( using a global variable) that the word is found Within the object array loop for span tabs ( where the indexOf statement is located) and after all span tabs are highlighted, send that number to a new html element ( a div, h1, span, p choice is up to you). The count should be continually accumulated and should show the total number of times a word is found in a span tab for all user activated clicks of the search button for…arrow_forwardHow to create a product table page: To create the product list page, you can copy the index.html file you worked on in exercise 7 to the products folder. Then, you can delete the content from the main section and modify the URLs on the page as necessary. • Modify the horizontal navigation menu so it indicates that the product list page is the current page. • Add a table to the section with a caption, a header, and a body as shown above. Be sure to merge the rows in the first column for each category so the category name is displayed only in the first row. Create a new style sheet named summary.css for the product list page, and copy the styles you need from the main.css file to this style sheet. Then, modify the link element for the style sheet in the products/index.html file so it points to the correct style sheet. • Align the caption, headings, and data, and apply any other required formatting as shown above. Use a structural pseudo-class selector to apply a background color of…arrow_forward
- Event Listeners Return to the mas_register.js file in your editor. Directly below the initial comment section, insert an event listener for the window load event. Run an anonymous function in response to the event containing the following commands: Call the calcCart() function (which you will create shortly.) Create an onclick event handler for the regSubmit button that runs the sessionTest() function when the button is clicked. Create onblur event handlers for the input boxes with the ids: fnBox, lnBox, groupBox, mailBox, phoneBox, and banquetBox, running the calcCart() function in response to each event. Create an onchange event handler for the sessionBox selection list, running the calcCart() function when the selection list is changed. Create an onclick event handler for the mediaCB check box, running the calcCart() function in response. JavaScript Functions Create the sessionTest() function. The purpose of this function is to provide a validation test for the conference session…arrow_forwardthis is my menu- need to code jquery selector & .change handler so that when these are selected & image shows, i have done other things with the html, its just this i am stuck on- keep getting the same image for all 4 when i want a different image per option! <p>Please select a direction:<br/><select id="mydirection" name="direction"><option value="N">North</option><option value="S">South</option><option value="E">East</option><option value="W">West</option></select></p>arrow_forwardEvent Listeners Go to the co_credit.js file in your editor. Create an event listener for the window load event that retrieves the field values attached to the query string of the page’s URL. Add the following to the event listener’s anonymous function: Create the orderData variable that stores the query string text from the URL. Slice the orderData text string to remove the first ? character, replace every occurrence of the + character with a blank space, and decode the URI-encoded characters. Split the orderData variable at every occurrence of a & or = character and store the substrings in the orderFields array variable. Write the following values from the orderFields array into the indicated fields of the order form: orderFields[3] into the modelName field orderFields[5] into the modelQty field orderFields[7] into the orderCost field orderFields[9] into the shippingType field orderFields[13] into the shippingCost field orderFields[15] into the subTotal field orderFields[17]…arrow_forward
- 1. Form Validation (do not use validation plugin) A.Create the following form using html Register With Our Web Site Email Address Re-enter Email Address First Name: Last Name 2-Character State Code 5-Digit Zip Code Jen our List B. write Javascript code to add the following validations 1. All the text boxes should have required field validator Register With Our Web Site Email Address This teld s requared. Re-enter Email Address paanbar pio First Name peanber s po Last Name. penbor s pre 2-Character State Code paanbar s poy 5-Digit Zip Code Thes bold is required. oin our List Resetarrow_forward1. It is possible to display the date on which the file was last saved using: Detail View Open Access Page layout Review Pane Developer Panearrow_forwardChoose: ANY keyboard event ANY mouse event and either Scroll, Resize, Focus, Blur, Select, or Change Make sure it's clear in the HTML what the user (aka ME) should be doing to trigger your events.Make 3 HTML/JavaScript files (use embeded aka <script> tags to make it easier) one for the old HTML method, the DOM Event Handler method, and the Event Listener method. They should look all the same just have the events coded differently.Subject matter can be whatever you want, the assignment is not dependent on content as it is functionality. Just make it PG, obviously! You can have fun with it. Silly is fine.arrow_forward
- Event Listeners Go to the co_cart.js file in your editor. Directly below the initial comment section, add an event listener for the window load event that does the following when the page is loaded: Runs the calcCart() function. Add an event handler to the modelQty field in the cart form that runs the calcCart() function when the field value is changed. A for loop that loops through every option in the group of shipping option buttons, adding an event handler to run the calcCart() function when each option button is clicked. JavaScript Functions Create the calcCart() function to calculate the cost of the customer’s order using field values in the cart form. Within the calcCart() function do the following: Create a variable named orderCost that is equal to the cost of the espresso machine stored in the modelCost field multiplied by the quantity of machines ordered as stored in the modelQty field. Display the value of the orderCost variable in the orderCost field, formatted as U.S.…arrow_forward7. You would like to add a drop down list box to your web page showing each department but your code below is only showing d.DepartmentName not the actual department name when you run your application. How can you change the code so it works correctly? @foreach (Department d in ViewBag.Departments) { <option value="d.DepartmentID">d.DepartmentName</option> } 1. @foreach (Department d in ViewBag.Departments) should be changed to: @foreach (Department d in model.Departments) 2. @foreach (Department d in ViewBag.Departments) should be changed to: list(d in ViewBag.Departments) 3. <option value="d.DepartmentID">d.DepartmentName</option> should be changed to: <option value="d.DepartmentName">d.DepartmentName</option> 4. <option value="d.DepartmentID">d.DepartmentName</option> should be changed to: <option value="@d.DepartmentID">@d.DepartmentName</option>arrow_forwardPlease answer in python with showing the code. (this is a 1 ques in 3 part please answer the ques) i. Create a python dictionary to organize the following info: a) The capital of the USA is Washington. The capital of France is Paris. The capital of India is New Delhi. b) Print the dictionary. ii. Create the dictionary to organize the following info: a) Our 2 students are Dembe and Kate. Dembe’s major is History. Kate’s major is Anthropology. Dembe’s hobbies are chess, judo, and drawing. Kate’s hobbies are bird-watching, stained-glass, poetry, and skating. b) Print the dictionaries. iii. Create a list of dictionaries to organize the following state info: a) Alaska’s capital is Juneau. Alaska’s population is 731,545. Its size is 665,000 square miles b) Hawaii’s capital is Honolulu. Hawaii’s population is 1,415,872. Its size is 10,931 square miles. c)Print the listarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
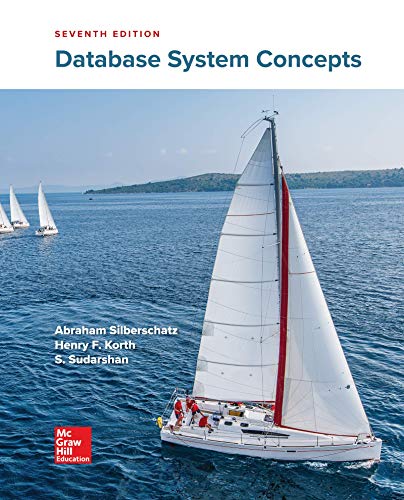
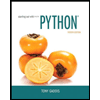
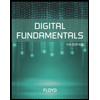
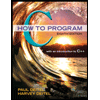
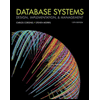
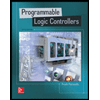