whenever you enter the date of birth as gibberish it still takes it, I want it to be a conditional statement where it forces the user to input the date of birth in the format of mm/dd/yyyy and it has to be an 18 years of age for the year if younger than that it will give an input validation message saying you have to be 18 to create an account, import tkinter as tk class ATM: def __init__(self): self.accounts = {} def create_account(self): first_name = first_name_entry.get() last_name = last_name_entry.get() dob = dob_entry.get() address = address_entry.get() debit_card = debit_card_entry.get() while len(debit_card) != 16 or not debit_card.isdigit(): debit_card = input("Invalid debit card number. Enter debit card number (16 digits): ") pin = pin_entry.get() while len(pin) != 4 or not pin.isdigit(): pin = input("Invalid pin. Enter 4-digit pin: ") self.accounts[debit_card] = {"first_name": first_name, "last_name": last_name, "dob": dob, "address": address, "pin": pin, "balance": 0} status_label.config(text="Account created successfully!") def login(self): debit_card = login_debit_card_entry.get() pin = login_pin_entry.get() if debit_card in self.accounts and self.accounts[debit_card]["pin"] == pin: while True: choice = login_choice_entry.get() if choice == "1": amount = float(deposit_entry.get()) self.accounts[debit_card]["balance"] += amount status_label.config(text="Deposit successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"]) break elif choice == "2": amount = float(withdraw_entry.get()) if amount <= self.accounts[debit_card]["balance"]: self.accounts[debit_card]["balance"] -= amount status_label.config(text="Withdraw successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"]) break else: status_label.config(text="Insufficient balance.") break elif choice == "3": status_label.config(text="Current balance: $%.2f" % self.accounts[debit_card]["balance"]) break elif choice == "4": status_label.config(text="Logged out.") break else: status_label.config(text="Invalid choice.") break else: status_label.config(text="Invalid debit card or pin.") atm = ATM() # create GUI root = tk.Tk() root.title("ATM software") create_account_frame = tk.Frame(root) create_account_frame.pack(padx=10, pady=10) tk.Label(create_account_frame, text="Enter first name:").grid(row=0, column=0) first_name_entry = tk.Entry(create_account_frame) first_name_entry.grid(row=0, column=1) tk.Label(create_account_frame, text="Enter last name:").grid(row=1, column=0) last_name_entry = tk.Entry(create_account_frame) last_name_entry.grid(row=1, column=1) tk.Label(create_account_frame, text="Enter date of birth (dd/mm/yyyy):").grid(row=2, column=0) dob_entry = tk.Entry(create_account_frame) dob_entry.grid(row=2, column=1) tk.Label(create_account_frame, text="Enter address:").grid(row=3, column=0) address_entry = tk.Entry(create_account_frame) address_entry.grid(row=3, column=1) tk.Label(create_account_frame, text="Enter debit card number (16 digits):").grid(row=4, column=0) debit_card_entry = tk.Entry(create_account_frame) debit_card_entry.grid(row=4, column=1) tk.Label(create_account_frame, text="Enter 4-digit pin:").grid(row=5, column=0) pin_entry = tk.Entry(create_account_frame, show="*") pin_entry.grid(row=5, column=1) create_account_button = tk.Button(create_account_frame, text="Create account", command=atm.create_account) create_account_button.grid(row=6, column=0, columnspan=2) login_frame = tk.Frame(root) login_frame.pack(padx=10, pady=10) tk.Label(login_frame, text="Enter debit card number:").grid(row=0, column=0) login_debit_card_entry = tk.Entry(login_frame) login_debit_card_entry.grid(row=0, column=1) tk.Label(login_frame, text="Enter 4-digit pin:").grid(row=1, column=0) login_pin_entry = tk.Entry(login_frame, show="*") login_pin_entry.grid(row=1, column=1) tk.Label(login_frame, text="1. Deposit\n2. Withdraw\n3. Check balance\n4. Logout").grid(row=2, column=0) login_choice_entry = tk.Entry(login_frame) login_choice_entry.grid(row=2, column=1) deposit_entry = tk.Entry(login_frame) deposit_entry.grid(row=3, column=0) withdraw_entry = tk.Entry(login_frame) withdraw_entry.grid(row=3, column=1) login_button = tk.Button(login_frame, text="Login", command=atm.login) login_button.grid(row=4, column=0, columnspan=2) status_label = tk.Label(root, text="") status_label.pack() root.mainloop()
whenever you enter the date of birth as gibberish it still takes it, I want it to be a conditional statement where it forces the user to input the date of birth in the format of mm/dd/yyyy and it has to be an 18 years of age for the year if younger than that it will give an input validation message saying you have to be 18 to create an account, import tkinter as tk class ATM: def __init__(self): self.accounts = {} def create_account(self): first_name = first_name_entry.get() last_name = last_name_entry.get() dob = dob_entry.get() address = address_entry.get() debit_card = debit_card_entry.get() while len(debit_card) != 16 or not debit_card.isdigit(): debit_card = input("Invalid debit card number. Enter debit card number (16 digits): ") pin = pin_entry.get() while len(pin) != 4 or not pin.isdigit(): pin = input("Invalid pin. Enter 4-digit pin: ") self.accounts[debit_card] = {"first_name": first_name, "last_name": last_name, "dob": dob, "address": address, "pin": pin, "balance": 0} status_label.config(text="Account created successfully!") def login(self): debit_card = login_debit_card_entry.get() pin = login_pin_entry.get() if debit_card in self.accounts and self.accounts[debit_card]["pin"] == pin: while True: choice = login_choice_entry.get() if choice == "1": amount = float(deposit_entry.get()) self.accounts[debit_card]["balance"] += amount status_label.config(text="Deposit successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"]) break elif choice == "2": amount = float(withdraw_entry.get()) if amount <= self.accounts[debit_card]["balance"]: self.accounts[debit_card]["balance"] -= amount status_label.config(text="Withdraw successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"]) break else: status_label.config(text="Insufficient balance.") break elif choice == "3": status_label.config(text="Current balance: $%.2f" % self.accounts[debit_card]["balance"]) break elif choice == "4": status_label.config(text="Logged out.") break else: status_label.config(text="Invalid choice.") break else: status_label.config(text="Invalid debit card or pin.") atm = ATM() # create GUI root = tk.Tk() root.title("ATM software") create_account_frame = tk.Frame(root) create_account_frame.pack(padx=10, pady=10) tk.Label(create_account_frame, text="Enter first name:").grid(row=0, column=0) first_name_entry = tk.Entry(create_account_frame) first_name_entry.grid(row=0, column=1) tk.Label(create_account_frame, text="Enter last name:").grid(row=1, column=0) last_name_entry = tk.Entry(create_account_frame) last_name_entry.grid(row=1, column=1) tk.Label(create_account_frame, text="Enter date of birth (dd/mm/yyyy):").grid(row=2, column=0) dob_entry = tk.Entry(create_account_frame) dob_entry.grid(row=2, column=1) tk.Label(create_account_frame, text="Enter address:").grid(row=3, column=0) address_entry = tk.Entry(create_account_frame) address_entry.grid(row=3, column=1) tk.Label(create_account_frame, text="Enter debit card number (16 digits):").grid(row=4, column=0) debit_card_entry = tk.Entry(create_account_frame) debit_card_entry.grid(row=4, column=1) tk.Label(create_account_frame, text="Enter 4-digit pin:").grid(row=5, column=0) pin_entry = tk.Entry(create_account_frame, show="*") pin_entry.grid(row=5, column=1) create_account_button = tk.Button(create_account_frame, text="Create account", command=atm.create_account) create_account_button.grid(row=6, column=0, columnspan=2) login_frame = tk.Frame(root) login_frame.pack(padx=10, pady=10) tk.Label(login_frame, text="Enter debit card number:").grid(row=0, column=0) login_debit_card_entry = tk.Entry(login_frame) login_debit_card_entry.grid(row=0, column=1) tk.Label(login_frame, text="Enter 4-digit pin:").grid(row=1, column=0) login_pin_entry = tk.Entry(login_frame, show="*") login_pin_entry.grid(row=1, column=1) tk.Label(login_frame, text="1. Deposit\n2. Withdraw\n3. Check balance\n4. Logout").grid(row=2, column=0) login_choice_entry = tk.Entry(login_frame) login_choice_entry.grid(row=2, column=1) deposit_entry = tk.Entry(login_frame) deposit_entry.grid(row=3, column=0) withdraw_entry = tk.Entry(login_frame) withdraw_entry.grid(row=3, column=1) login_button = tk.Button(login_frame, text="Login", command=atm.login) login_button.grid(row=4, column=0, columnspan=2) status_label = tk.Label(root, text="") status_label.pack() root.mainloop()
Chapter6: Looping
Section: Chapter Questions
Problem 10PE
Related questions
Question
100%
whenever you enter the date of birth as gibberish it still takes it, I want it to be a conditional statement where it forces the user to input the date of birth in the format of mm/dd/yyyy and it has to be an 18 years of age for the year if younger than that it will give an input validation message saying you have to be 18 to create an account,
import tkinter as tk
class ATM:
def __init__(self):
self.accounts = {}
def create_account(self):
first_name = first_name_entry.get()
last_name = last_name_entry.get()
dob = dob_entry.get()
address = address_entry.get()
debit_card = debit_card_entry.get()
while len(debit_card) != 16 or not debit_card.isdigit():
debit_card = input("Invalid debit card number. Enter debit card number (16 digits): ")
pin = pin_entry.get()
while len(pin) != 4 or not pin.isdigit():
pin = input("Invalid pin. Enter 4-digit pin: ")
self.accounts[debit_card] = {"first_name": first_name, "last_name": last_name, "dob": dob, "address": address,
"pin": pin, "balance": 0}
status_label.config(text="Account created successfully!")
def login(self):
debit_card = login_debit_card_entry.get()
pin = login_pin_entry.get()
if debit_card in self.accounts and self.accounts[debit_card]["pin"] == pin:
while True:
choice = login_choice_entry.get()
if choice == "1":
amount = float(deposit_entry.get())
self.accounts[debit_card]["balance"] += amount
status_label.config(text="Deposit successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"])
break
elif choice == "2":
amount = float(withdraw_entry.get())
if amount <= self.accounts[debit_card]["balance"]:
self.accounts[debit_card]["balance"] -= amount
status_label.config(text="Withdraw successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"])
break
else:
status_label.config(text="Insufficient balance.")
break
elif choice == "3":
status_label.config(text="Current balance: $%.2f" % self.accounts[debit_card]["balance"])
break
elif choice == "4":
status_label.config(text="Logged out.")
break
else:
status_label.config(text="Invalid choice.")
break
else:
status_label.config(text="Invalid debit card or pin.")
atm = ATM()
# create GUI
root = tk.Tk()
root.title("ATM software")
create_account_frame = tk.Frame(root)
create_account_frame.pack(padx=10, pady=10)
tk.Label(create_account_frame, text="Enter first name:").grid(row=0, column=0)
first_name_entry = tk.Entry(create_account_frame)
first_name_entry.grid(row=0, column=1)
tk.Label(create_account_frame, text="Enter last name:").grid(row=1, column=0)
last_name_entry = tk.Entry(create_account_frame)
last_name_entry.grid(row=1, column=1)
tk.Label(create_account_frame, text="Enter date of birth (dd/mm/yyyy):").grid(row=2, column=0)
dob_entry = tk.Entry(create_account_frame)
dob_entry.grid(row=2, column=1)
tk.Label(create_account_frame, text="Enter address:").grid(row=3, column=0)
address_entry = tk.Entry(create_account_frame)
address_entry.grid(row=3, column=1)
tk.Label(create_account_frame, text="Enter debit card number (16 digits):").grid(row=4, column=0)
debit_card_entry = tk.Entry(create_account_frame)
debit_card_entry.grid(row=4, column=1)
tk.Label(create_account_frame, text="Enter 4-digit pin:").grid(row=5, column=0)
pin_entry = tk.Entry(create_account_frame, show="*")
pin_entry.grid(row=5, column=1)
create_account_button = tk.Button(create_account_frame, text="Create account", command=atm.create_account)
create_account_button.grid(row=6, column=0, columnspan=2)
login_frame = tk.Frame(root)
login_frame.pack(padx=10, pady=10)
tk.Label(login_frame, text="Enter debit card number:").grid(row=0, column=0)
login_debit_card_entry = tk.Entry(login_frame)
login_debit_card_entry.grid(row=0, column=1)
tk.Label(login_frame, text="Enter 4-digit pin:").grid(row=1, column=0)
login_pin_entry = tk.Entry(login_frame, show="*")
login_pin_entry.grid(row=1, column=1)
tk.Label(login_frame, text="1. Deposit\n2. Withdraw\n3. Check balance\n4. Logout").grid(row=2, column=0)
login_choice_entry = tk.Entry(login_frame)
login_choice_entry.grid(row=2, column=1)
deposit_entry = tk.Entry(login_frame)
deposit_entry.grid(row=3, column=0)
withdraw_entry = tk.Entry(login_frame)
withdraw_entry.grid(row=3, column=1)
login_button = tk.Button(login_frame, text="Login", command=atm.login)
login_button.grid(row=4, column=0, columnspan=2)
status_label = tk.Label(root, text="")
status_label.pack()
root.mainloop()
Expert Solution

Step 1
Algorithm steps to solve the given problem:
- Start
- Create a class called ATM which will hold all the account details.
- Create an empty dictionary called 'accounts' inside the ATM class to store the details of the accounts.
- Define a function called 'create_account' which will get user inputs for account creation.
- Validate the user inputs such as date of birth, debit card number and pin.
- If the user is under 18 years old, display an error message.
- If the user inputs are valid, create an account and update the accounts dictionary with the account details.
- Define a function called 'login' which will get user inputs for login and perform transactions.
- Check if the entered debit card and pin match with the account details in the accounts dictionary.
- If the details match, ask the user for the choice of transaction.
- If the choice is deposit, ask for the amount and update the account balance.
- If the choice is withdraw, ask for the amount and check if there is enough balance. If there is enough balance, update the account balance.
- If the choice is check balance, display the account balance.
- If the choice is logout, display a message and exit the loop.
- If the entered debit card or pin is incorrect, display an error message.
- Create a GUI using tkinter library with two frames- one for account creation and another for login.
- Inside the create account frame, create labels and entry boxes for user inputs such as first name, last name, date of birth, address, debit card number and pin.
- Create a button called 'Create account' which will call the 'create_account' function when clicked.
- Inside the login frame, create labels and entry boxes for debit card number, pin, transaction choice, deposit amount and withdraw amount.
- Create a button called 'Login' which will call the 'login' function when clicked.
- Create a label called 'status_label' to display status messages for the user.
- Run the tkinter mainloop to display the GUI.
- Stop.
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
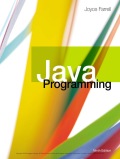
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
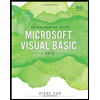
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
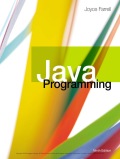
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
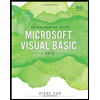
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
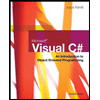
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,