Write a program that first reads in the name of an input file and then reads the input file using the file.readlines() method. The input file contains an unsorted list of number of seasons followed by the corresponding TV show. Your program should put the contents of the input file into a dictionary where the number of seasons are the keys, and a list of TV shows are the values (since multiple shows could have the same number of seasons). Sort the dictionary by key (least to greatest) and output the results to a file named output_keys.txt, separating multiple TV shows associated with the same key with a semicolon (;). Next, sort the dictionary by values (alphabetical order), and output the results to a file named output_titles.txt. Ex: If the input is: file1.txt and the contents of file1.txt are: 20 Gunsmoke 30 The Simpsons 10 Will & Grace 14 Dallas 20 Law & Order 12 Murder, She Wrote the file output_keys.txt should contain: 10: Will & Grace 12: Murder, She Wrote 14: Dallas 20: Gunsmoke; Law & Order 30: The Simpsons and the file output_titles.txt should contain: Dallas Gunsmoke Law & Order Murder, She Wrote The Simpsons Will & Grace Note: There is a newline at the end of each output file, and file1.txt is available to download.
7.9 LAB: Sorting TV Shows (dictionaries and lists)
Write a program that first reads in the name of an input file and then reads the input file using the file.readlines() method. The input file contains an unsorted list of number of seasons followed by the corresponding TV show. Your program should put the contents of the input file into a dictionary where the number of seasons are the keys, and a list of TV shows are the values (since multiple shows could have the same number of seasons).
Sort the dictionary by key (least to greatest) and output the results to a file named output_keys.txt, separating multiple TV shows associated with the same key with a semicolon (;). Next, sort the dictionary by values (alphabetical order), and output the results to a file named output_titles.txt.
Ex: If the input is:
file1.txtand the contents of file1.txt are:
20the file output_keys.txt should contain:
10: Will & Graceand the file output_titles.txt should contain:
DallasNote: There is a newline at the end of each output file, and file1.txt is available to download.
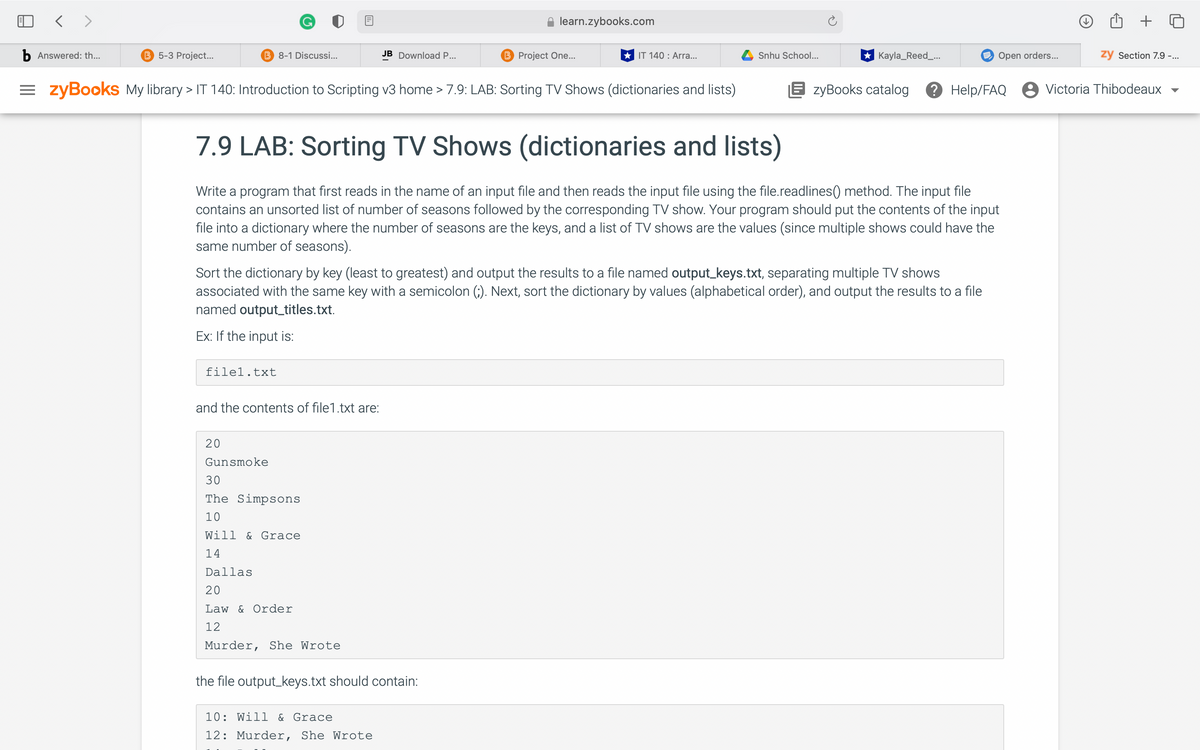
![learn.zybooks.com
b Answered: th...
B 5-3 Project...
B 8-1 Discussi...
JB Download P...
B Project One...
* IT 140 : Arra...
Snhu School...
* Kayla_Reed_...
E Open orders...
zy Section 7.9 -...
= zyBookS My library > IT 140: Introduction to Scripting v3 home > 7.9: LAB: Sorting TV Shows (dictionaries and lists)
E zyBooks catalog
2Help/FAQ
Victoria Thibodeaux
10: Will & Grace
12: Murder, She Wrote
14: Dallas
20: Gunsmoke; Law & Order
30: The Simpsons
and the file output_titles.txt should contain:
Dallas
Gunsmoke
Law & Order
Murder, She Wrote
The Simpsons
Will & Grace
Note: There is a newline at the end of each output file, and file1.txt is available to download.
247772.1992070.qx3zqy7
LAB
7.9.1: LAB: Sorting TV Shows (dictionaries and lists)
0/10
АCTIVITY
Downloadable files
filel.txt
Download
main.py
Load default template.
1 # accepting user input for filename
2 file = input("Enter your filename :")
3 # empty dictionary
4 D = {}
5 try:
6 # opening user file
7
fin = open(file,"r")
8 # reading file using readlines
9 L =
fin.readlines()
10 # assigning index = 0
11 index = 0
12 # loop till index + 1 is less than len(L)
13 while index + 1 < len(L):
14 # get the key from the line
15 key = L[index].strip("\n")
16 # get the value from the nextline
17 value = L[index+1].strip("\n")
18 # check if key not in Dictioanry
Run your program as often as you'd like, before submitting for grading. Below, type any needed
input values in the first box, then click Run program and observe the program's output in the
Develop mode
Submit mode
second box.
Enter program input (optional)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0fb6fbe8-c93b-4e2e-8100-eedf21471e21%2F72fa6fd9-9976-4aa8-8fc9-34356c641ef1%2Fihc5hz_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

After working on it some more this is what I am getting now.
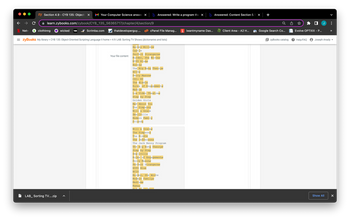
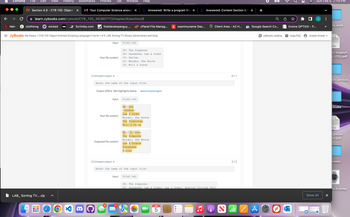
I've tried so many things and it still isnt working
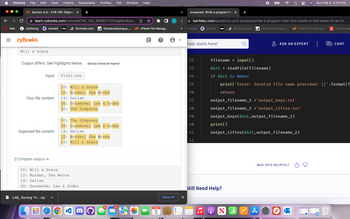
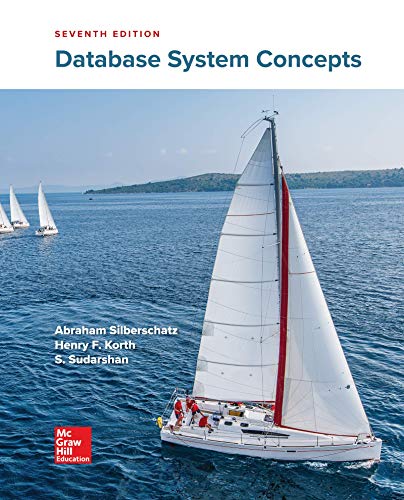
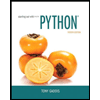
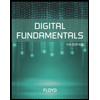
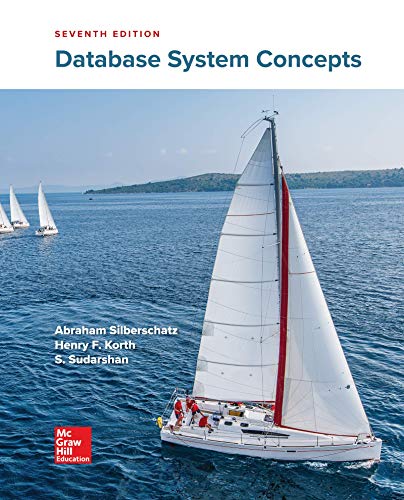
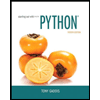
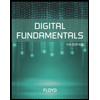
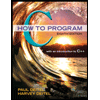
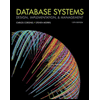
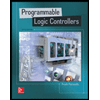