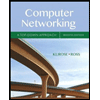
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
![Q1.1.
Write a NumPy program to get the following array by using loops
Output:
array([[ 1, 2, 3, 4, 5, 6, 7, 8, 9],
[2, 4, 6, 8, 10, 12, 14, 16, 18],
[3, 6, 9, 12, 15, 18, 21, 24, 27],
[4, 8, 12, 16, 20, 24, 28, 32, 36],
[5, 10, 15, 20, 25, 30, 35, 40, 45],
[6, 12, 18, 24, 30, 36, 42, 48, 54],
[7, 14, 21, 28, 35, 42, 49, 56, 63],
[8, 16, 24, 32, 40, 48, 56, 64, 72],
[9, 18, 27, 36, 45, 54, 63, 72, 81]])
Q1.2. Replace all odd numbers in array with -1.
Q1.3. By using numpy functions and prog array, get the result.
prog = np.array([1,2,3,4,6]).
Result= array([1, 1, 1, 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 2, 2, 2, 3, 3, 3, 3, 3, 3,
3, 3, 4, 4, 4, 4, 4, 4, 4, 4, 6, 6, 6, 6, 6, 6, 6, 6, 1, 2, 3, 4,
6, 1, 2, 3, 4, 6, 1, 2, 3, 4, 6, 1, 2, 3, 4, 6])
Q1.4. Write a NumPy program to create random 2 array and size of arrays should be 1x15. In
your array there should be integer numbers from 0 to 9 and by using numpy function, find
common numbers in your array.
Q1.5. Write a NumPy program to generate 50 random number between 0 and 150. If your
number bigger than 100, make this number 100 and if your number smaller than 50 make this
number 50 by using numpy functions.
Q1.6. Write a NumPy program to generate 16 random integer numbers. Then convert this array
to 4x4 array. Repeat this operation and create 3 different 4x4 arrays. By getting summation of
these 3 arrays, find the average array, and calculate the maximum, minimum, mean, and
standard deviation of each line of matrix values.](https://content.bartleby.com/qna-images/question/2e0b3a7a-a57f-4179-a98f-952aae65c7c9/ec390c95-6fb1-4809-be80-fb50e9de4f47/0n3l8q_thumbnail.png)
Transcribed Image Text:Q1.1.
Write a NumPy program to get the following array by using loops
Output:
array([[ 1, 2, 3, 4, 5, 6, 7, 8, 9],
[2, 4, 6, 8, 10, 12, 14, 16, 18],
[3, 6, 9, 12, 15, 18, 21, 24, 27],
[4, 8, 12, 16, 20, 24, 28, 32, 36],
[5, 10, 15, 20, 25, 30, 35, 40, 45],
[6, 12, 18, 24, 30, 36, 42, 48, 54],
[7, 14, 21, 28, 35, 42, 49, 56, 63],
[8, 16, 24, 32, 40, 48, 56, 64, 72],
[9, 18, 27, 36, 45, 54, 63, 72, 81]])
Q1.2. Replace all odd numbers in array with -1.
Q1.3. By using numpy functions and prog array, get the result.
prog = np.array([1,2,3,4,6]).
Result= array([1, 1, 1, 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 2, 2, 2, 3, 3, 3, 3, 3, 3,
3, 3, 4, 4, 4, 4, 4, 4, 4, 4, 6, 6, 6, 6, 6, 6, 6, 6, 1, 2, 3, 4,
6, 1, 2, 3, 4, 6, 1, 2, 3, 4, 6, 1, 2, 3, 4, 6])
Q1.4. Write a NumPy program to create random 2 array and size of arrays should be 1x15. In
your array there should be integer numbers from 0 to 9 and by using numpy function, find
common numbers in your array.
Q1.5. Write a NumPy program to generate 50 random number between 0 and 150. If your
number bigger than 100, make this number 100 and if your number smaller than 50 make this
number 50 by using numpy functions.
Q1.6. Write a NumPy program to generate 16 random integer numbers. Then convert this array
to 4x4 array. Repeat this operation and create 3 different 4x4 arrays. By getting summation of
these 3 arrays, find the average array, and calculate the maximum, minimum, mean, and
standard deviation of each line of matrix values.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Similar questions
- python exercise Given the following list: testlist2 = (23,50,12,80,10,90,34,78,4,19,53) Use testlist2 to create Numpy array of int16 and then display the array. Display the number of dimension of testnp. Display the shape of testnp. Display the number of bytes of testnp. Display the mean, sum, max, and min of testnp.arrow_forwardWrite a loop that will fill an array that holds 30 integers in C. Declare the array & any mecessary variables. Remember to prompt the user for each integer.arrow_forwardUse Python code with: "def recolorImage(img,color):" without using cv2 or PIL recolor(img, color) - Changes all non-white pixels of the image referred to by the parameter img to the specified color, and returns a new image with the changes. img is a 2D array of RGB pixels which represent images. color is a list containing 3 integers, each from 0 to 255, representing RGB values. To create a new image use the cmpt120image.getBlackImage() function which returns a new canvas to draw on.arrow_forward
- Implement an empty integer array of capacity 5. a) Use a loop to input values from the console into the array. b) Use a loop to determine the smallest element in the array. c) Use a loop to determine the largest element in the array. d) Use a loop to output the array. e) Output the smallest and largest elements. Example Output [0] = 4 [1] = 24 [2] = 123 [3] = 54 [4] = 3 Array: 4 24 123 54 3 Max: 123 Min: 3 programming language is c++ pleasw do it in a easy wayarrow_forwardThe Lo Shu Magic Square is a grid with 3 rows and 3 columns shown below. The Lo Shu Magic Square has the following properties: The grid contains the numbers 1 – 9 exactly The sum of each row, each column and each diagonal all add up to the same number. This is shown below: Write a program that simulates a magic square using 3 one dimensional parallel arrays of integer type. Do not use two-dimensional array. Each one the arrays corresponds to a row of the magic square. The program asks the user to enter the values of the magic square row by row and informs the user if the grid is a magic square or not. Processing Requirements - c++ Use the following template to start your project: #include<iostream> using namespace std; // Global constants const int ROWS = 3; // The number of rows in the array const int COLS = 3; // The number of columns in the array const int MIN = 1; // The value of the smallest number const int MAX = 9; // The value of the largest number //…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
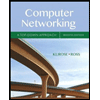
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
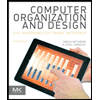
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
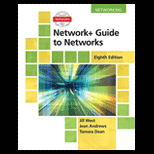
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
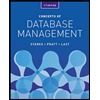
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
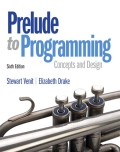
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
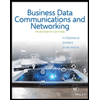
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY