wish to further break it down into (i) a function that walks the grid in a direction you specify the string read-out that it encounters; and (ii) a function that checks if the word appears in read-out string. make_unique(lst: list[str]) -> list[str] takes a list of words 1st (with possible d cates) and returns a list of words from 1st, each with exactly one copy. ple: Suppose you call word_sleuth with words = ["bog", "moon", "rabbit", "the", "bit", "raw"] he following grid: [["r","a","w","b","i","t"], ["x","a","y","z","c","h"], ["p","q","b" ["t","r", ["u","w","x","v". ["n","m","r" "i","e"], "b","o","g"], ,"t"], ,"t"]] 'w" should return a list with the following members ["raw", "bit","rabbit", "bog", "th program must output the same set of words but may (and probably will) output them in a diffe . Note: even though bit appears twice in the grid, we only list it once in the output. nd Rules: For this task, you must not import any package. You must build everything ch. rmance Expectations: While in this class, we aren't crazy about speedy code, your code e excessively slow. For this problem, it means finishing under 1 second on a 75×75 grid
wish to further break it down into (i) a function that walks the grid in a direction you specify the string read-out that it encounters; and (ii) a function that checks if the word appears in read-out string. make_unique(lst: list[str]) -> list[str] takes a list of words 1st (with possible d cates) and returns a list of words from 1st, each with exactly one copy. ple: Suppose you call word_sleuth with words = ["bog", "moon", "rabbit", "the", "bit", "raw"] he following grid: [["r","a","w","b","i","t"], ["x","a","y","z","c","h"], ["p","q","b" ["t","r", ["u","w","x","v". ["n","m","r" "i","e"], "b","o","g"], ,"t"], ,"t"]] 'w" should return a list with the following members ["raw", "bit","rabbit", "bog", "th program must output the same set of words but may (and probably will) output them in a diffe . Note: even though bit appears twice in the grid, we only list it once in the output. nd Rules: For this task, you must not import any package. You must build everything ch. rmance Expectations: While in this class, we aren't crazy about speedy code, your code e excessively slow. For this problem, it means finishing under 1 second on a 75×75 grid
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Python Language:
Word Sleuth Program
![wish to further break it down into (i) a function that walks the grid in a direction you specify and
the string read-out that it encounters; and (ii) a function that checks if the word appears in the
read-out string.
make_unique (1st: list[str]) -> list[str] takes a list of words 1st (with possible dupli-
cates) and returns a list of words from 1st, each with exactly one copy.
Example: Suppose you call word_sleuth with
words =
["bog", "moon", "rabbit", "the", "bit", "raw"]
and the following grid:
[["r","a","w","b","i","t"],
["x",
"y","z","c","h"],
'i","e"],
["p","q"
["t",
["u","w","x",
["n","m","r","w","o","t"]]
,"g"],
,"i","t"],
This should return a list with the following members ["raw", "bit","rabbit", "bog", "the"].
Your program must output the same set of words but may (and probably will) output them in a different
order. Note: even though bit appears twice in the grid, we only list it once in the output.
Ground Rules:
For this task, you must not import any package. You must build everything from
scratch.
Performance Expectations: While in this class, we aren't crazy about speedy code, your code must
not be excessively slow. For this problem, it means finishing under 1 second on a 75x75 grid with
about 50 words in the input.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F48954d8a-c361-4523-b68b-ac12d6ced482%2F23251c3c-e592-446d-81ba-4f94c31be2cf%2Fg7zwf6j_processed.png&w=3840&q=75)
Transcribed Image Text:wish to further break it down into (i) a function that walks the grid in a direction you specify and
the string read-out that it encounters; and (ii) a function that checks if the word appears in the
read-out string.
make_unique (1st: list[str]) -> list[str] takes a list of words 1st (with possible dupli-
cates) and returns a list of words from 1st, each with exactly one copy.
Example: Suppose you call word_sleuth with
words =
["bog", "moon", "rabbit", "the", "bit", "raw"]
and the following grid:
[["r","a","w","b","i","t"],
["x",
"y","z","c","h"],
'i","e"],
["p","q"
["t",
["u","w","x",
["n","m","r","w","o","t"]]
,"g"],
,"i","t"],
This should return a list with the following members ["raw", "bit","rabbit", "bog", "the"].
Your program must output the same set of words but may (and probably will) output them in a different
order. Note: even though bit appears twice in the grid, we only list it once in the output.
Ground Rules:
For this task, you must not import any package. You must build everything from
scratch.
Performance Expectations: While in this class, we aren't crazy about speedy code, your code must
not be excessively slow. For this problem, it means finishing under 1 second on a 75x75 grid with
about 50 words in the input.
![Word sleuth (also known under various other names) is a popular word game found in newspapers
and puzzle books. In such a puzzle, letters of words are placed in a grid, and the objective is to find and
mark all the words hidden inside the grid.
You can read words:
horizontally from left to right;
vertically from top to bottom;
• diagonally from top-left to bottom-right; or
diagonally from bottom-left to top-right.
A word w appears in a grid if w can be found along one of these directions of reading, with the letters
of w appearing consecutively along the path of reading.
In this task, you’ll implement a function
word_sleuth(grid: list[list[str]], words: list[str])
that takes as parameters:
• grid-a 2-dimensional list representing the input grid, and
• words –a list of words whose presence you look for in grid.
Your function will return a list of all the words in the words list that appear in the grid. The words may
appear in any order in the output list but may not contain duplicates.
How to Get Started?
You will want to break this problem into a few functions, each representing a
logical unit of task. We suggest writing at least the following helper functions:
contains_word(grid: list[1ist[str]], w: str) -> bool takes the grid from above and
one single word and checks if the grid contains the word w. To implement this function, you may](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F48954d8a-c361-4523-b68b-ac12d6ced482%2F23251c3c-e592-446d-81ba-4f94c31be2cf%2Fgg1nvqi_processed.png&w=3840&q=75)
Transcribed Image Text:Word sleuth (also known under various other names) is a popular word game found in newspapers
and puzzle books. In such a puzzle, letters of words are placed in a grid, and the objective is to find and
mark all the words hidden inside the grid.
You can read words:
horizontally from left to right;
vertically from top to bottom;
• diagonally from top-left to bottom-right; or
diagonally from bottom-left to top-right.
A word w appears in a grid if w can be found along one of these directions of reading, with the letters
of w appearing consecutively along the path of reading.
In this task, you’ll implement a function
word_sleuth(grid: list[list[str]], words: list[str])
that takes as parameters:
• grid-a 2-dimensional list representing the input grid, and
• words –a list of words whose presence you look for in grid.
Your function will return a list of all the words in the words list that appear in the grid. The words may
appear in any order in the output list but may not contain duplicates.
How to Get Started?
You will want to break this problem into a few functions, each representing a
logical unit of task. We suggest writing at least the following helper functions:
contains_word(grid: list[1ist[str]], w: str) -> bool takes the grid from above and
one single word and checks if the grid contains the word w. To implement this function, you may
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
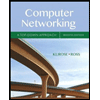
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
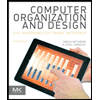
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
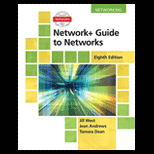
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
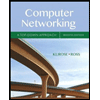
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
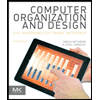
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
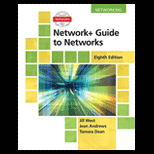
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
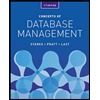
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
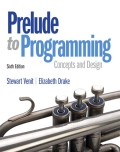
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
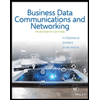
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY