Write a C++ program to calculate the gravity of the petrol using multiple inheritance Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. Consider a class Liguid with the following protected member variable
QUESTION PROVIDED IN ATTACH IMAGE KINDLY SEE. PROVIDE OUTPUT AS IT IS SHOWN IN QUESTION.
AND BELOW TEMPLATES PROVIDED CHECK THIS BEFORE MAKING SOLUTION ( main.cpp , donor.cpp , address.cpp )
----------------- TEMPLATES BELOW FOR SOLUTION -----------------------
main.cpp template
#include<iostream> using namespace std; #include "PetrolBO.cpp" int main() { cout<<"Enter the details\n"; cout<<"Enter the density of the petrol"; cin>>density; cout<<"Enter the rate of the petrol"; cin>>rate; cout<<"Enter the thermal conductivity of the petrol"<<endl; cin>>thermalConductivity; //Fill your code here return 0; } |
Petrol.cpp template
#include<iostream>
|
Fuel.cpp template
#include<iostream>
|
Liquid.cpp template
#include<iostream>
|
petrolBo.cpp template
#include<iostream> using namespace std; #include"Petrol.cpp" class PetrolBO { public: void display(Petrol p) { //Fill your code here } }; |
![Write a C++ program to calculate the gravity of the petrol using multiple inheritance
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class
names, member variable names, and function names should be the same as specified in the problem
statement.
Consider a class Liquid with the following protected member variable.
Datatype
float
Variables
density
Include appropriate getter and setters.
Consider a class Fuel with the following protected member variable.
Datatype
float
Variables
rate
Include appropriate getters and setters
Consider a derived class Petrol derived from Liquid and Fuel and it contains the following member
variables.
Data Type
float
int
Variable
gravity
thermalConductivity
Include appropriate getters and setters
Consider a class PetrolBO with the following method
Method description
Method
void display(Petrol p) This method is used to display the petrol details.
In the main method, inputs are obtained from the user and access the display method of PetrolBO by
creating instances.
Note:To calculate gravity use gravity = (density/997.0).
Input and Output format:
Refer to sample input and output for other further details and format of the output.
[All texts in bold corresponds to the input and rest are output]
Sample Input and Output:
Enter the details
Enter the density of the petrol
560
Enter the rate of the petrol
89
Enter the thermal conductivity of the petrol
465
Density: 560
Rate:89
Thermal Conductivity:465
Gravity: 0.561685](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8c25729a-1e93-4e66-ac0f-a14f9c5cbd04%2Febc464af-055e-49cd-8561-d39f50543ba3%2F289ess_processed.png&w=3840&q=75)

Step by step
Solved in 6 steps with 1 images

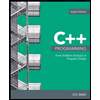
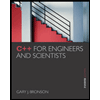
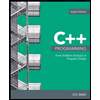
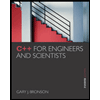