Write a C++ program to find the distance walked by them and display the result using the operator overloading concept.
Write a C++ program to find the distance walked by them and display the result using the operator overloading concept.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter12: Adding Functionality To Your Classes
Section12.2: Providing Class Conversion Capabilities
Problem 6E
Related questions
Question
QUESTION PROVIDED IN ATTACH IMAGE KINDLY SEE. PROVIDE OUTPUT AS IT IS SHOWN IN QUESTION.
AND BELOW TEMPLATES PROVIDED CHECK THIS BEFORE MAKING SOLUTION ( main.cpp , distance.cpp )
----------------- TEMPLATES BELOW FOR SOLUTION -----------------------
main.cpp
#include<iostream> #include "Distance.cpp" using namespace std; int main() { //Fill your code here } |
Distance.cpp
#include<iostream> class Distance void dispDistance() { Distance operator+(Distance &dist1) { |

Transcribed Image Text:Reena and Kavya planned to go walking daily in the morning. Both of them started to walk long
distances. One day they decided to find how much distance they were walking.
Write a C++ program to find the distance walked by them and display the result using the operator
overloading concept.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class
names, member variable names, and function names should be the same as specified in the problem
statement.
Consider a class named Distance with the following member variables.
Datatype
int
Variable
feet
linches
int
Define the following public methods in the class Distance.
Method Name
Description
void readDistance()
This function is used to get the input value from the user.
void displayDistance()
This function is used to display the total distance travelled by them.
This function is used to perform an addition operation.
The '+' operator is overloaded in this function.
It is used to calculate the distance traveled by them.
Distance operator+(Distance &dist1)
In the main method call all the methods to implement the respective methods and call the
readDistance() method twice for getting the inputs of feet and inches for Reena and Kavya.
Input and Output Format :
Refer sample input and output for formatting specifications.
All text in bold corresponds to input and the rest corresponds to output.
Sample input and output 1:
Enter the distance travelled by Reena:
Enter feet:
Enter inches:
2
Enter the distance travelled by Kavya:
Enter feet:
6
Enter inches:
Total Distance travelled by them:
Feet:11
Inches:5
Sample input and output 2:
Enter the distance travelled by Reena:
Enter feet:
7
Enter inches:
3
Enter the distance travelled by Kavya:
Enter feet:
8
Enter inches:
4
Total Distance travelled by them:
Feet:15
Inches:7
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
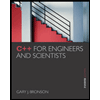
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
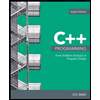
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
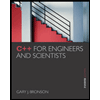
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
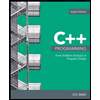
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning