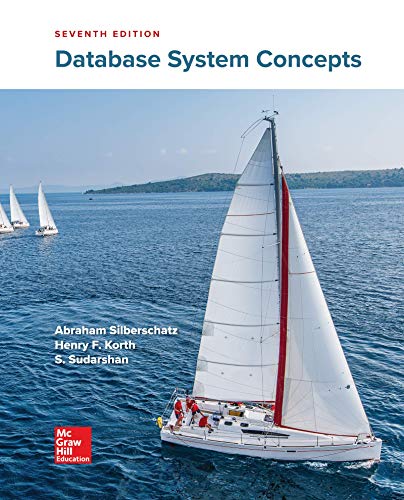
Concept explainers
Write a C program to implement a queue of at most elements using an array of size n.
⦁ At the beginning of your code (outside all subprograms), declare a datatype named `struct Queue`. It must be designed according to the pseudo codes (refer to Figure 2) and the provided main function
⦁ Define the subprograms to implement the build, enqueue, and dequeue operations. The meaning of enqueue and dequeue is explained in the pseudocodes in Figure 2. The pseudocodes do not include error checking for underflow and overflow. You are required to extend and implement them to prevent underflow and overflow of the queue.
⦁ Additionally, your code must check for any other potential errors, such as accessing null pointers. Also, add a few comments to your code to explain the behavior of the main and subprograms. Ensure that your build, enqueue, and dequeue operations print informative messages as demonstrated in Figure 3.
Ensure you test your code with varying sequences of enqueue and dequeue operations. Including five test cases should be sufficient
![API
void build(struct Queue **q, unsigned int length)
void enqueue(struct Queue *q, char x)
char dequeue(struct Queue *q)
(a) Q
Return value
Builds the queue of the specified length'. Initializes
the queue elements. The memory address of the
created queue is stored in q.
No return value. Side effect; x stored at the tail of
Q. The tail is incremented by one.
1
(b) Q35
Returns the value stored at the head. Head
incremented. Returns error code, if some
encountered.
1 2 3 4 5 6 7 8 9
15 69
Q.head = 7
2 3 4 5 6 7 8 9
10 11 12
84
Q.tail 3 Q.head = 7
Q.tail = 12
10 11 12
15 69 84 17
1
2 3 4 5 6 7 8 9 10 11 12
(c) Q35
15 698 4 17
Q.tail = 3
Q.head=8
Figure 1 A queue implemented using an array Q[1..12].
Figure 1 shows the effects of the enqueue and dequeue operations. Queue elements
appear only in the lightly shaded positions. Figure 1(a) shows a case where the queue
has 5 elements, in locations Q[7..11]. Figure 1(b) shows the configuration of the queue
after the calls ENQUEUE(Q.17), ENQUEUE(Q, 3), and ENQUEUE(Q, 5). Figure
1(c) shows the configuration of the queue after the call DEQUEUE(Q) which returns
the key value 15 formerly at the head of the queue. The new head has the value 6
(stored at the position 8).
The following pseudo codes describe the meaning of the enqueue and dequeue
operations.](https://content.bartleby.com/qna-images/question/877b16ea-bdd0-4584-a6e2-d20d1a116b05/480260b6-66e3-4f68-9da1-6c91c64e9471/crqjgm_thumbnail.png)
![ENQUEUE (Q, x)
Q[Q.tail] = X
if Q.tail == Q.length
Q.tail 1
=
else Q.tail = Q.tail + 1
1
2
3
4
DEQUEUE(Q)
1 x
2
3
x = Q[Q.head]
if Q.head == Q.length
Q.head = 1
4
5 return x
Figure 2 Pseudocodes for enqueue and dequeue subprograms
else Q.head =
enqueue(qptr, 1);
enqueue(qptr, 2);
enqueue(qptr, 3);
enqueue(qptr, 4);
enqueue(qptr, 5);
enqueue(qptr, 6);
build(&qptr, 5);
//====== Beginn Test Case
Test
Your code will be tested using the following main function:
int main(int argc, const char * argv[]) {
struct Queue *qptr = NULL;
Q. head + 1
printf("Dequeue() %d\n", dequeue(qptr));
printf("Dequeue() %d\n", dequeue(qptr));
printf("Dequeue() %d\n", dequeue(qptr));
printf("Dequeue() %d\n", dequeue(qptr));
printf("Dequeue() %d\n", dequeue(qptr));
printf("Dequeue() %d\n", dequeue(qptr));
//====== End Test Case
// free anything that was created with malloc
if (qptr->data) free(qptr->data);
if(qptr) free(qptr);](https://content.bartleby.com/qna-images/question/877b16ea-bdd0-4584-a6e2-d20d1a116b05/480260b6-66e3-4f68-9da1-6c91c64e9471/71r7k9_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- Write a C++ program that: (1) defines and implements a hash class that constructs a 15 element array (may be implemented using a vector, a deque, or a list, if you prefer), storing strings, using the following hash function: ((first_letter) + (second_letter) + (last_letter))% 15 (2) the driver program should: a. query the user for ten words and store them using the hash technique described above. b. print out the contents of each position of the array (or vector, deque, or whatever you used), showing vacant as well as filled positions. Remember, only 10 of the 15 positions will be filled. c. repeatedly query the user for a target word, hash the word, check for its inclusion in the list of stored words, and report the result. Continue doing this task until the user signals to stop (establish a sentinel condition).arrow_forwardWrite a C++ program that: (1) defines and implements a hash class that constructs a 15 element array (may be implemented using a vector, a deque, or a list, if you prefer, (using the STL implementations), storing strings, using the following hash function: ((first_letter) + (last_letter) - (second_letter))% 15 (2) the driver program should: a. query the user for ten words and store them using the hash technique described above. b. print out the contents of each position of the array (or vector, deque, or whatever you used), showing vacant as well as filled positions. Remember, only 10 of the 15 positions will be filled. c. repeatedly query the user for a target word, hash the word, check for its inclusion in the list of stored words, and report the result. Continue doing this task until the user signals to stop (establish a sentinel condition).arrow_forwardIn a programming language (Pascal), the declaration of a node in a singly linked list is shown in Figure P6.7(a). The list referred to for the problem is shown in Figure P6.7(b). Given P to be a pointer to a node, the instructions DATA(P) and LINK(P) referring to the DATA and LINK fields, respectively, of node P are equivalently represented by P↑. DATA and P↑. LINK in the programming language. What do the following commands do to the logical representation of the list T? i) ii) TYPE POINTER=NODE; NODE RECORD DATA: integer; LINK: POINTER END; VAR P, Q R: T POINTER (a) Declaration of a node in a singly linked list T 31 TE 57 12 (b) A singly linked list T iii) R₁.LINK: = Q iv) R₁.DATA: = P₁.DATA: Q₁.DATA + R₁.DATA Q: = P |R Figure P6.7. (a and b) Declaration of a node in a programming language and the logical representation of a singly linked list T Q.LINK.DATA + 10 91 Linked Lists 189arrow_forward
- Write C++ code to copy elements from an old queue to new queue utilizing the enqueue() and dequeue() methods, thereby leaving the old queue empty.arrow_forwardWrite a C++ code that compare the times to traverse a list (containing a large number of elements) implemented in an array, in a simple linked list, and in an unrolled linked list.arrow_forwardYou will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
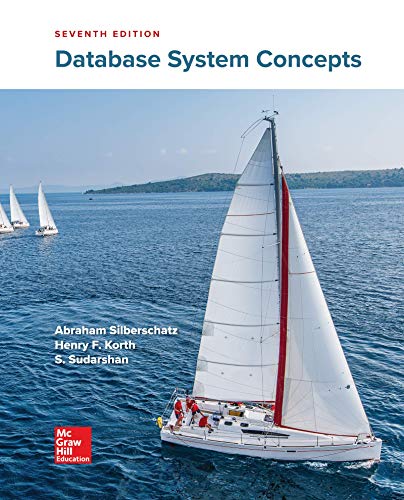
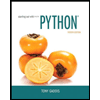
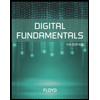
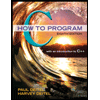
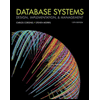
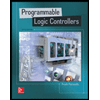