Write a class called Game that contains a video game's name, genre, and difficulty Level. Include a default constructor and destructor for the class. The constructor should print out the following message: "Creating a new game". The destructor should print out the following message: "In the Game destructor." Include appropriate get/set functions for the class. In main(), prompt the user to enter the number of games he or she has played in the past year. Dynamically create a built-in array based on this number (not a vector or object of the array class) to hold pointers to Game objects. Construct a loop in main() that executes once for each of the number of games that the user indicated. Within this loop, ask the user to enter the name and genre of each game. Using a random number generator, generate a difficulty Level between 1-10 (inclusive). Seed this random number generator with 100. Next, dynamically create a Game object (remember that this requires the use of the "new" keyword which returns a pointer to the location in memory where this game object was created.) Create each object using the default constructor of the class, and call the set functions to store the name, genre, and difficulty level of each game. Store each Game pointer in the array. After all of the Game objects have been constructed and added to the array, print out the contents of the array. Because the program uses dynamic memory to store the array as well as the objects in the array, be sure to de-allocate all of the memory before exiting.
Write a class called Game that contains a video game's name, genre, and difficulty Level. Include a default constructor and destructor for the class. The constructor should print out the following message: "Creating a new game". The destructor should print out the following message: "In the Game destructor." Include appropriate get/set functions for the class. In main(), prompt the user to enter the number of games he or she has played in the past year. Dynamically create a built-in array based on this number (not a vector or object of the array class) to hold pointers to Game objects. Construct a loop in main() that executes once for each of the number of games that the user indicated. Within this loop, ask the user to enter the name and genre of each game. Using a random number generator, generate a difficulty Level between 1-10 (inclusive). Seed this random number generator with 100. Next, dynamically create a Game object (remember that this requires the use of the "new" keyword which returns a pointer to the location in memory where this game object was created.) Create each object using the default constructor of the class, and call the set functions to store the name, genre, and difficulty level of each game. Store each Game pointer in the array. After all of the Game objects have been constructed and added to the array, print out the contents of the array. Because the program uses dynamic memory to store the array as well as the objects in the array, be sure to de-allocate all of the memory before exiting.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
LOOK AT THE OUTPUT IMAGE CAREFULLY. IT NEEDS TO BE SAME.please make sure you input all details as like the output image.
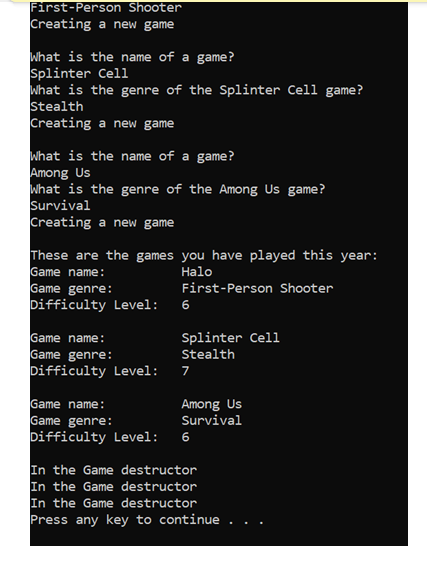
Transcribed Image Text:First-Person Shooter
Creating a new game
what is the name of a game?
Splinter Cell
What is the genre of the Splinter Cell game?
Stealth
Creating a new game
What is the name of a game?
Among Us
what is the genre of the Among Us game?
Survival
Creating a new game
These are the games you have played this year:
Game name:
Наlo
Game genre:
Difficulty Level:
First-Person Shooter
6
Game name:
Game genre:
Difficulty Level:
Splinter Cell
Stealth
7
Game name:
Game genre:
Difficulty Level:
Among Us
Survival
6
In the Game destructor
In the Game destructor
In the Game destructor
Press any key to continue .
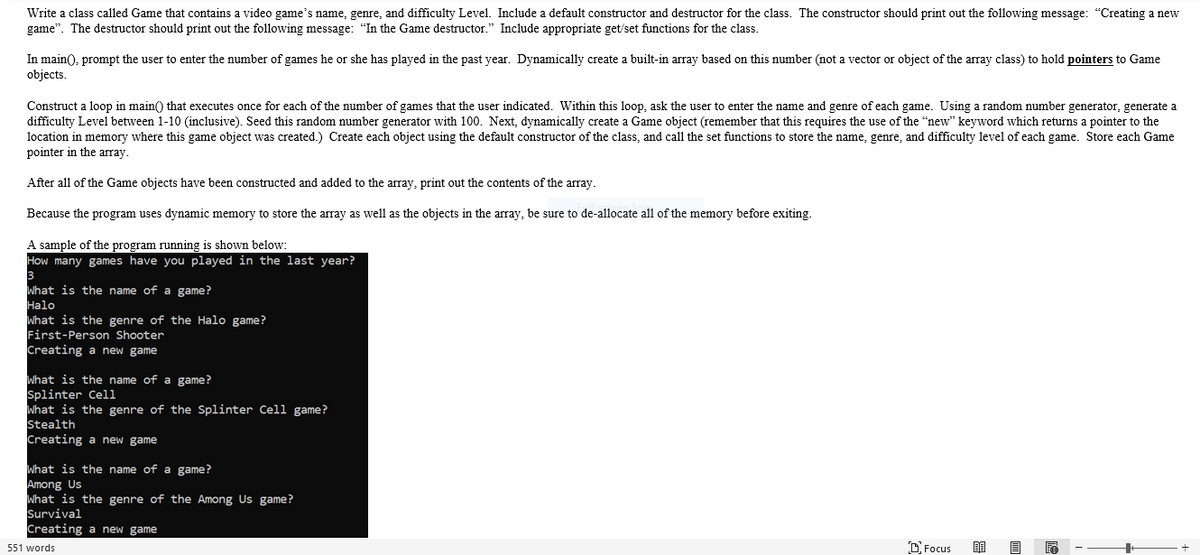
Transcribed Image Text:Write a class called Game that contains a video game's name, genre, and difficulty Level. Include a default constructor and destructor for the class. The constructor should print out the following message: “Creating a new
game". The destructor should print out the following message: "In the Game destructor." Include appropriate get/set functions for the class.
In main(), prompt the user to enter the number of games he or she has played in the past year. Dynamically create a built-in array based on this number (not a vector or object of the array class) to hold pointers to Game
objects.
Construct a loop in main() that executes once for each of the number of games that the user indicated. Within this loop, ask the user to enter the name and genre of each game. Using a random number generator, generate a
difficulty Level between 1-10 (inclusive). Seed this random number generator with 100. Next, dynamically create a Game object (remember that this requires the use of the "new" keyword which returns a pointer to the
location in memory where this game object was created.) Create each object using the default constructor of the class, and call the set functions to store the name, genre, and difficulty level of each game. Store each Game
pointer in the array.
After all of the Game objects have been constructed and added to the array, print out the contents of the array.
Because the program uses dynamic memory to store the array as well as the objects in the array, be sure to de-allocate all of the memory before exiting.
A sample of the program running is shown below:
How many games have you played in the last year?
3
What is the name of a game?
Halo
What is the genre of the Halo game?
First-Person Shooter
Creating a new game
What is the name of a game?
Splinter Cell
What is the genre of the Splinter Cell game?
Stealth
Creating a new game
What is the name of a game?
Among Us
What is the genre of the Among Us game?
Survival
Creating a new game
551 words
D Focus
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Recommended textbooks for you
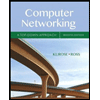
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
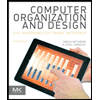
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
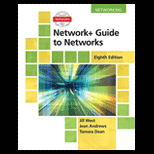
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
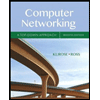
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
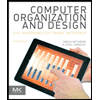
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
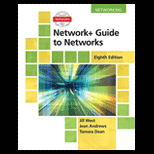
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
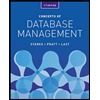
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
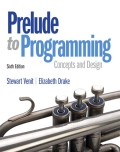
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
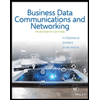
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY