PROBLEM: Write a program that computes the equivalent resistance of a purely series and purely parallel electric circuit. SPECIFICATIONS that you need to follow: • The program will ask the user to choose whether to compute for the equivalent resistance of a series or parallel combination. • The user will input three resistance values R,, R2, and R3 in ohms. • The program will provide an output of equivalent resistance in series (Rs) or parallel (Rp). The program must contain classes and objects. You may only use the codes that we studied under our lectures. All lines of codes should have a comment/pseudocode. To recall, the formula for solving the resistance for a purely series and purely parallel circuit are: a. Series Circuit: R1 120 ohms Rs = RT = R1 + R2 + R3++Rn R2 Vs 100 V 30 ohms R3 50 ohms b. Parallel Circuit: 1 1 1 + + R3 R1 R2 1 %3D Rp RT R1 R2 Vs 40 ohms 10 ohms 40 Volts
I already have a code, please help me to fix the code.
#include <iostream>
#include <iomanip>
using namespace std;
class Circuit {
public:
void series();
void parallel();
double R1,R2,R3;
//Define function to return the combined resistance in parallel
double calculateCombResParallel() {
return (1/((1/R1)+(1/R2)+(1/R3)));
}
//Define function to return the combined resistance in series
double calculateCombResSerial() {
return (R1+R2+R3);
}
};
int main()
{
int opt;
char r;
cin.get();
do {
system ("cls")
cout << "please select an option" <<endl <<endl; //selection of options
cout << "(A) Series" << endl;
cout << "(B) Parallel" << endl;
cout << "Select Option: ";
cin >> opt;
system ("cls");
switch(opt)
//Create object of Circuit class
{
Circuit c1;
//Get the values of three resistors
cout<<"Enter the value of resistor 1 in ohms: ";
cin>>c1.R1;
cout<<"Enter the value of resistor 2 in ohms: ";
cin>>c1.R2;
cout<<"Enter the value of resistor 3 in ohms: ";
cin>>c1.R3;
// Calculate and display the combined resistance or serial circuit
cout<<"\nresistance in series: "<< c1.calculateCombResSerial() << endl;
break;
Circuit c2;
//Get the values of three resistors
cout<<"Enter the value of resistor 1 in ohms: ";
cin>>c1.R1;
cout<<"Enter the value of resistor 2 in ohms: ";
cin>>c1.R2;
cout<<"Enter the value of resistor 3 in ohms: ";
cin>>c1.R3;
// Calculate and display the combined resistance or serial circuit
cout<<"\nresistance in parallel: "<< c1.calculateCombResParallel() << endl;
break;
}
}
while (opt != 0);
return 0;
}
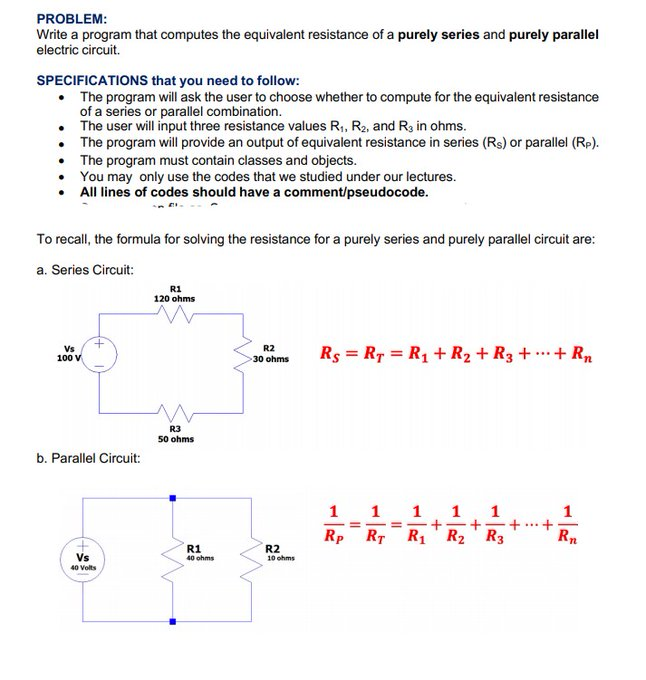
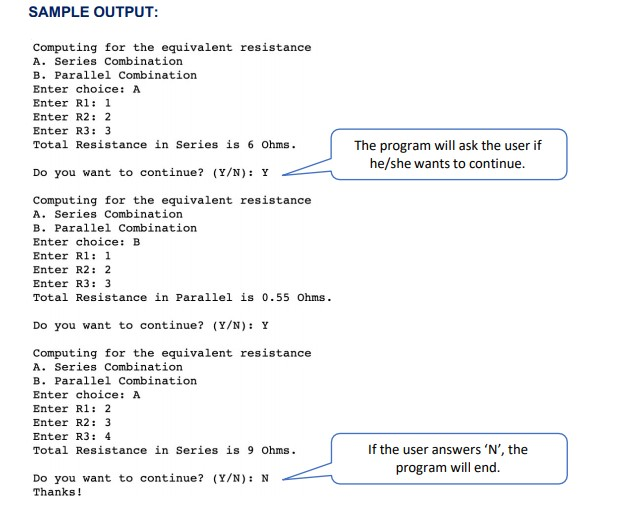

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

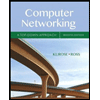
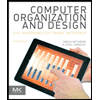
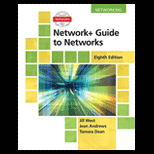
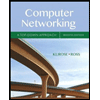
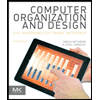
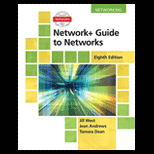
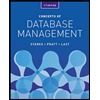
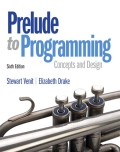
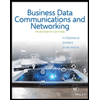