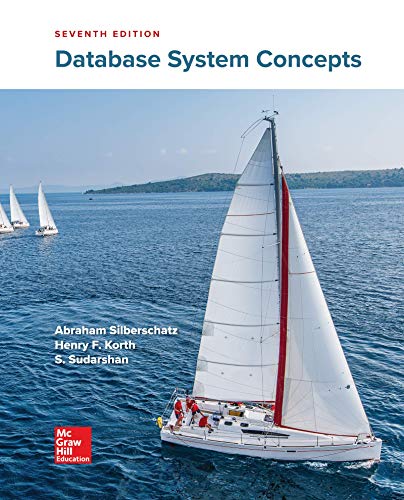
Write a class named Car that has the following private member variables: yearModel: An int that holds the car's year model make: A string that holds the make of the car speed: An int that holds the car's current speed In addition, the class should have the following public member functions: Constructor: Accepts two arguments. The first argument is the car's year model, and the second argument is the car's make. These values should be assigned to the yearModel and make member variables. This constructor should assign 0 to the speed field. Default Constructor: Sets the yearModel member variable to 2022, sets the make member variable to an empty string (""), and sets the speed member variable to 0. setYearModel: Accepts an int argument that is assigned to the yearModel member variable. The function returns no value. setMake: Accepts a string argument that is assigned to the make member variable. The function returns no value. setSpeed: Accepts an int argument that is assigned to the speed member variable. The function returns no value. getYearModel: Returns the value stored in the yearModel member variable. getMake: Returns the value stored in the make member variable. getSpeed: Returns the value stored in the speed member variable. accelerate: Adds 5 to the speed member variable. The function returns no value. brake: Subtracts 5 from the speed member variable. The function returns no value. Note: Write only the class declaration for the Car class. Do not write a main function, and do not write any #include directives.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Lab 2 – Designing a class This lab requires you to think about the steps that take place in a program by writing pseudocode. Read the following program prior to completing the lab. Design a class named Computer that holds the make, model, and amount of memory of a computer. Include methods to set the values for each data field, and include a method that displays all the values for each field. For the programming problem, create the pseudocode that defines the class and enter it below. Enter pseudocode herearrow_forwardIN C++ Design a class that stores a temperature in a temperature member variable and has the appropriate constructor, setter and getter functions. In addition to these, the class should have the following member functions: isEthylFreezing. This function should return the bool value true if the temperature stored in the temperature field is at or below the freezing point of ethyl alcohol. Otherwise, the function should return false. isEthylBoiling. This function should return the bool value true if the temperature stored in the temperature field is at or above the boiling point of ethyl alcohol. Otherwise, the function should return false. • isWaterFreezing. This function should return the bool value true if the temperature stored in the temperature field is at or below the freezing point of water. Otherwise, the function should return false. isWaterBoiling. This function should return the bool value true if the temperature stored in the temperature field is at or above the boiling…arrow_forwardAll member variables should - in most cases - be declared with this access specifier. public private package none of the abovearrow_forward
- There are two types of data members in a class: static and non-static. Give an example of real-world use for a static data member.arrow_forwardEmployee and ProductionWorker ClassesCreate an Employee class that has properties for the following data:Employee nameEmployee numberNext, create a class named ProductionWorker that is derived from the Employee class. The ProductionWorker class should have properties to hold the following data:Shift number (an integer, such as 1, 2, or 3)Hourly pay rateThe workday is divided into two shifts: day and night. The Shift property will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2.Create an application that creates an object of the ProductionWorker class and lets the user enter data for each of the object’s properties. Retrieve the object’s properties and display their values. this.ReportViewer1.Employee(); cannot reference?arrow_forwardThis type of member function may be called from a function that is a member of the same class or a derived class. static private protected O None of thesearrow_forward
- 1. RetailItem Class Write a class named Retailltem that holds data about an item in a retail store. The class should have the following fields: • description. It is a String object that holds a brief description of the item. • unitsOnHand. It is an int variable that holds the number of units currently in inventory. • price. It is a double that holds the item's retail price. Write appropriate mutator methods that store values in these fields and accessor methods that return the values in these fields. Once you have written the class, write a separate program that creates three RetailItem objects and stores the following data in them. Units On Hold Description Designer Jeans Jacket Price 40 34.95 12 59.95 Shirt 20 24.95arrow_forward6. Patient Charges Write a class named Patient that has attributes for the following data: • First name, middle name, and last name • Address, city, state, and ZIP code • Phone number ● The Patient class's init__ method should accept an argument for each attribute. The Name and phone number of emergency contact Patient class should also have accessor and mutator methods for each attribute. Next, write a class named Procedure that represents a medical procedure that has been performed on a patient. The Procedure class should have attributes for the following data: • Name of the procedure • Date of the procedure • Name of the practitioner who performed the procedure • Charges for the procedure 107 The Procedure class's __init__ method should accept an argument for each attribute. The Procedure class should also have accessor and mutator methods for each attribute Next, write a program that creates an instance of the Patient class, initialized with sample data. Then, create three…arrow_forwardWrite a class named Coin . The Coin class should have the followingmember variable:A string named sideUp . The sideUp member variable will holdeither “heads” or “tails” indicating the side of the coin that isfacing up.The Coin class should have the following member functions:A default constructor that randomly determines the side of thecoin that is facing up (“heads” or “tails”) and initializes the sideUpmember variable accordingly.A void member function named toss that simulates the tossingof the coin. When the toss member function is called, itrandomly determines the side of the coin that is facing up(“heads” or “tails”) and sets the sideUp member variableaccordingly.A member function named getSideUp that returns the value ofthe sideUp member variable.Write a program that demonstrates the Coin class. The programshould create an instance of the class and display the side that isinitially facing up. Then, use a loop to toss the coin 20 times. Eachtime the coin is tossed, display the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
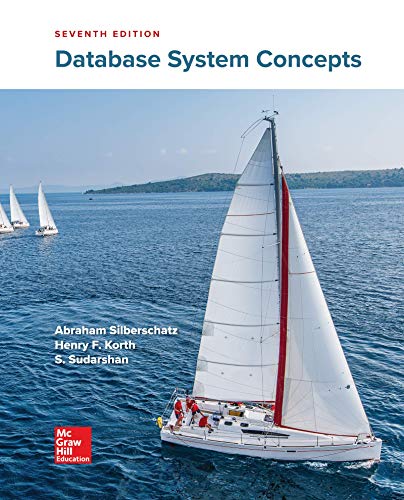
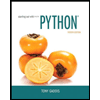
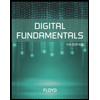
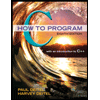
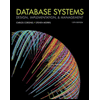
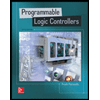