Write a class RangeInput that allows users to enter a value within a range of values that is provided in the constructor. An example would be a temperature control switch in a car that allows inputs between 60 and 80 degrees Fahrenheit. The input control has “up” and “down” buttons. Provide up and down methods to change the current value. The initial value is the midpoint between the limits. As with the preceding exercises, use Math.min and Math.max to limit the value. Write a sample program that simulates clicks on controls for the passenger and driver seats. Please ensure that it works with the test class provided
Write a class RangeInput that allows users to enter a value within a range of values that is provided in the constructor. An example would be a temperature control switch in a car that allows inputs between 60 and 80 degrees Fahrenheit. The input control has “up” and “down” buttons. Provide up and down methods to change the current value. The initial value is the midpoint between the limits. As with the preceding exercises, use Math.min and Math.max to limit the value. Write a sample program that simulates clicks on controls for the passenger and driver seats.
Please ensure that it works with the test class provided.
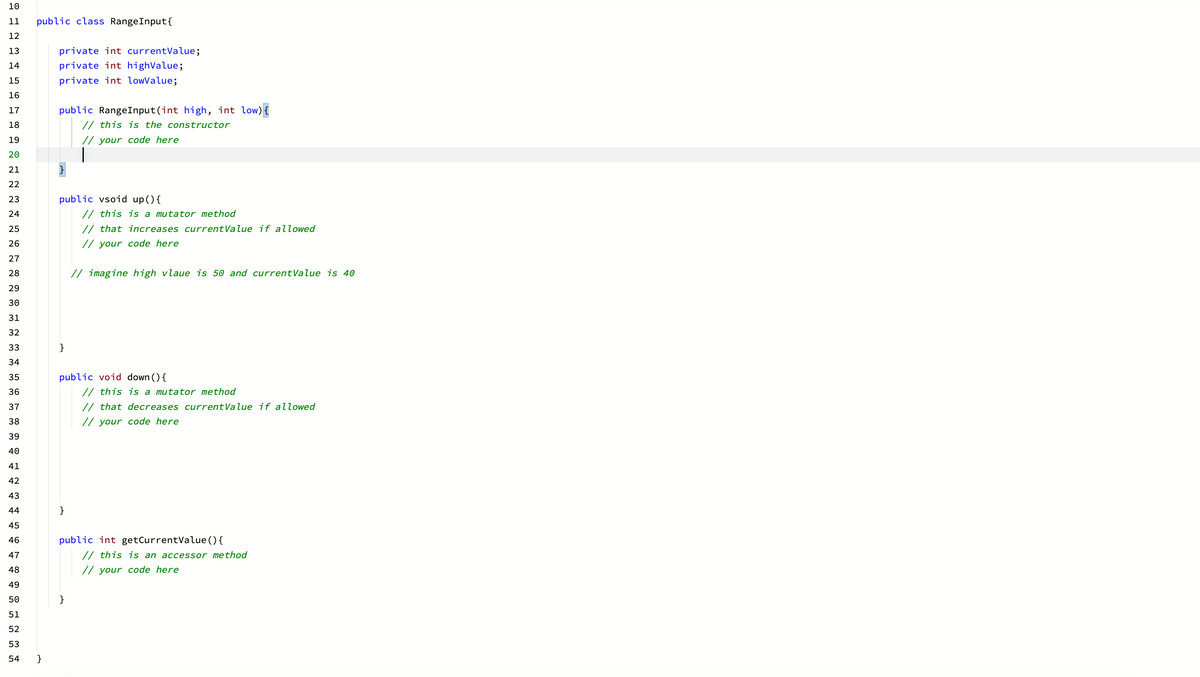
![public class RangeInputTester{
public static void main(String[] args){
RangeInput myInput = new RangeInput (30,20);
System.out.println("current value: " + myInput.getCurrentValue ());
for (int i=0;i<4;i++){
myInput.up();
}
System.out.println("new value: "
+ myInput.getCurrentValue ());
for (int i=0;i<50;i++){
myInput.down();
}
System.out.println("last value:
" + myInput.getCurrentValue ());
}
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F123fe9bd-f5bf-404f-97ce-90ae622db155%2Fcc428816-53cc-474e-9efd-52b204a85f30%2Fcw870tb_processed.png&w=3840&q=75)

Given
The answer is given below.
CODE:-
import java.util.Scanner;
class RangeInput
{
static int lower,upper,current;
public RangeInput(int lower,int upper)
{
lower = lower;
upper = upper;
current = (upper-lower) / 2;
}
public static void input()
{
Scanner sc = new Scanner(System.in);
System.out.println("Enter temperature between %d and %d:",lower,upper);
int temp = sc.nextInt();
if(temp >= lower && temp <=upper)
current = temp;
else
System.out.println("Invalid temperature");
}
public static void up()
{
if(current < Math.max(upper,lower))
current++;
else
System.out.println("Reached Maximum");
}
public static void down()
{
if(current > Math.min(upper,lower))
current--;
else
System.out.println("Reached Minimum");
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

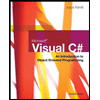
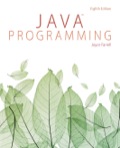
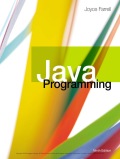
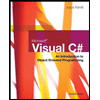
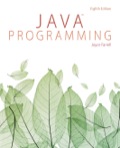
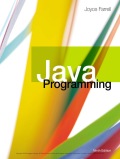