In this assignment, you will write a program that readsa list of people from a file, create a HashSet of the people, and print the people in the HashSet.The input filename ispassed in as a command line argument.•The program must implement a main class, and a Person class.•A template for the Person class is provided.A Person has a name and an address, both Strings.•If the input file name is not provided, your program should an error message and exit, e.g., Usage: java FuAssignment10/FuMain inputfile•If the specified input file does not exist or cannot be opened, your program should an error message and exit, e.g.,File abc.txt does not existor cannot be opened!•The input file lists one person per line. Each line contains aname and an address, separated by a comma, “,”.For example, the following are in a sample file students.txt:James Bond, 111 Main StTayer Smoke, 2334 Murry LnDavid Jones, 302 Toast AveAbby Wasch, 84 Sunny PlJames Bond, 111 Main St•Yourprogram should read each line, parseit, createa Person, and add the Person to a HashSet. •After all the lines are processed, your program should print the Persons in the HashSet.A sample run with the previous input file, students.txt, looks like this:Name: David JonesAddress: 302 Toast AveName: James BondAddress: 111 Main StName: Abby WaschAddress: 84 Sunny PlName: Tayler SmokeAddress: 2334 Murry LnNote:•HashSet does not allow duplicate elements. •The order of thePersons in theoutput is not specified. II.Duplicate Elements in HashSet•A HashSet does notallow duplicate elements. It uses the equals() method and the hashCode() methodof the elements to find duplicate elements.An elementis considered as a duplicate of another elementif they have the same hashCode() and the equals() returns true.•In the Person class, you must override the equals() method, which is used to compare 2 Personobjects. •Two Persons are considered equalif they have the same name and the same address. That is, the equals() method should return true if the two Person objects have the same name and address.•Two elements must also have the same hash code to be considered as duplicate, even if they areequal. That is, two Person objects with different hash codesare considered unique, even if they have the same name and address.•You also need to overwrite the hashCode() method of the Person class. The hashCode() method is used to calculate the hash code of the Personobject. •The defaulthashCode() of an object is its physical location, which is unique.
In this assignment, you will write a program that readsa list of people from a file, create a HashSet of the people, and print the people in the HashSet.The input filename ispassed in as a command line argument.•The program must implement a main class, and a Person class.•A template for the Person class is provided.A Person has a name and an address, both Strings.•If the input file name is not provided, your program should an error message and exit, e.g., Usage: java FuAssignment10/FuMain inputfile•If the specified input file does not exist or cannot be opened, your program should an error message and exit, e.g.,File abc.txt does not existor cannot be opened!•The input file lists one person per line. Each line contains aname and an address, separated by a comma, “,”.For example, the following are in a sample file students.txt:James Bond, 111 Main StTayer Smoke, 2334 Murry LnDavid Jones, 302 Toast AveAbby Wasch, 84 Sunny PlJames Bond, 111 Main St•Yourprogram should read each line, parseit, createa Person, and add the Person to a HashSet. •After all the lines are processed, your program should print the Persons in the HashSet.A sample run with the previous input file, students.txt, looks like this:Name: David JonesAddress: 302 Toast AveName: James BondAddress: 111 Main StName: Abby WaschAddress: 84 Sunny PlName: Tayler SmokeAddress: 2334 Murry LnNote:•HashSet does not allow duplicate elements. •The order of thePersons in theoutput is not specified. II.Duplicate Elements in HashSet•A HashSet does notallow duplicate elements. It uses the equals() method and the hashCode() methodof the elements to find duplicate elements.An elementis considered as a duplicate of another elementif they have the same hashCode() and the equals() returns true.•In the Person class, you must override the equals() method, which is used to compare 2 Personobjects. •Two Persons are considered equalif they have the same name and the same address. That is, the equals() method should return true if the two Person objects have the same name and address.•Two elements must also have the same hash code to be considered as duplicate, even if they areequal. That is, two Person objects with different hash codesare considered unique, even if they have the same name and address.•You also need to overwrite the hashCode() method of the Person class. The hashCode() method is used to calculate the hash code of the Personobject. •The defaulthashCode() of an object is its physical location, which is unique.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

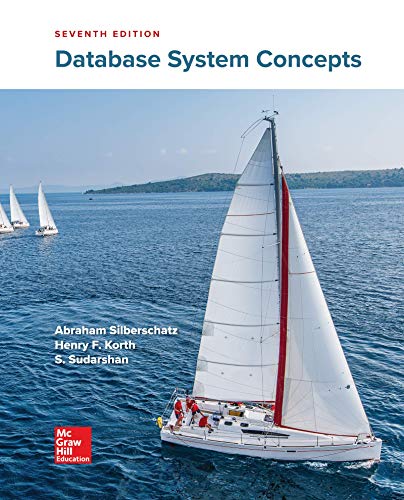
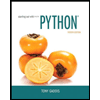
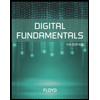
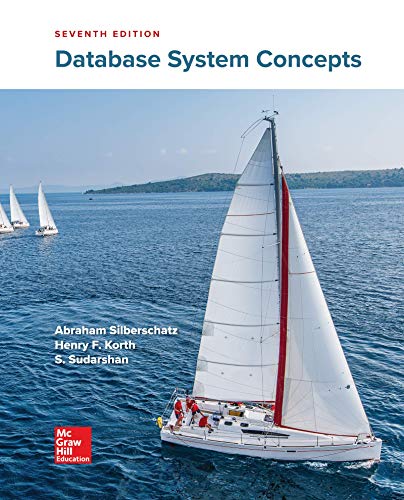
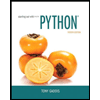
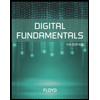
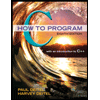
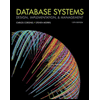
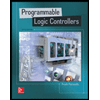