Write a complete C++ program that randomly generates 20 integers between the rangeof 1 to 50 and store the result in an array. You are only required to implement fiveof the following six functions. You may complete a sixth for extra credit. Use thesefunctions to test the array. The arguments for each of these functions will be thearray itself and its length (20).1. void print(int array[], int length);This function will simply print the array on a single line with a space in betweeneach entry.2. int findMax(int array[], int length);This function will return the largest number in the array.3. int findMin(int array[], int length);This function will return the smallest number in the array.4. double findAverage(int array[], int length);This function will return the average of the numbers in the array.5. int findMinGap(int array[], int length);This function will return the smallest gap between adjacent entries of the array. Agap between two numbers is the absolute value of their difference. For example, ifan array contains the elements {10, 14, -5, -3, 0, 5, 7}, the minimum gap is 2(between -5 and -3).6. int findGapSum(int array[], int length);This function will return the sum of gaps between adjacent entries of the array.Your main function should look something like this:int main() {int length = 20;int array[length];// code here to initialize the values of the array// note when passing an array to a function, you do not include the []print(array, length);cout << "max: " << findMax(array, length) << endl;cout << "min: " << findMin(array, length) << endl;cout << "avg: " << findAverage(array, length) << endl;cout << "min gap: " << findMinGap(array, length) << endl;cout << "gap sum: " << findGapSum(array, length) << endl;return 0;}And your output should look something like this:14 4 36 43 46 16 43 26 26 5 47 47 47 13 21 4 24 13 20 3max: 47min: 3avg: 25.4min gap: 0gap sum: 323
Write a complete C++ program that randomly generates 20 integers between the range
of 1 to 50 and store the result in an array. You are only required to implement five
of the following six functions. You may complete a sixth for extra credit. Use these
functions to test the array. The arguments for each of these functions will be the
array itself and its length (20).
1. void print(int array[], int length);
This function will simply print the array on a single line with a space in between
each entry.
2. int findMax(int array[], int length);
This function will return the largest number in the array.
3. int findMin(int array[], int length);
This function will return the smallest number in the array.
4. double findAverage(int array[], int length);
This function will return the average of the numbers in the array.
5. int findMinGap(int array[], int length);
This function will return the smallest gap between adjacent entries of the array. A
gap between two numbers is the absolute value of their difference. For example, if
an array contains the elements {10, 14, -5, -3, 0, 5, 7}, the minimum gap is 2
(between -5 and -3).
6. int findGapSum(int array[], int length);
This function will return the sum of gaps between adjacent entries of the array.
Your main function should look something like this:
int main() {
int length = 20;
int array[length];
// code here to initialize the values of the array
// note when passing an array to a function, you do not include the []
print(array, length);
cout << "max: " << findMax(array, length) << endl;
cout << "min: " << findMin(array, length) << endl;
cout << "avg: " << findAverage(array, length) << endl;
cout << "min gap: " << findMinGap(array, length) << endl;
cout << "gap sum: " << findGapSum(array, length) << endl;
return 0;
}
And your output should look something like this:
14 4 36 43 46 16 43 26 26 5 47 47 47 13 21 4 24 13 20 3
max: 47
min: 3
avg: 25.4
min gap: 0
gap sum: 323

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

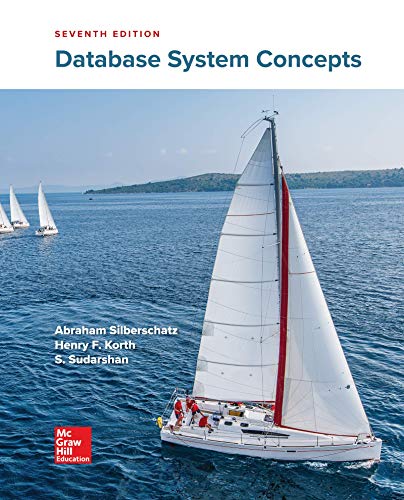
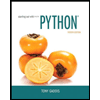
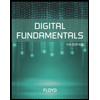
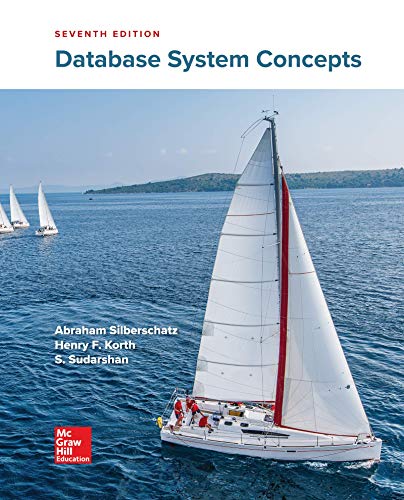
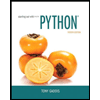
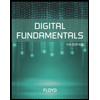
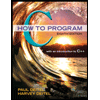
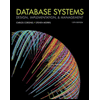
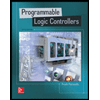