Write a function that will combine two arrays of different lengths into another array. Express the function's running time in terms of actual running time and Big-Oh notation. For reference, this is the function declaration: void combine(int* comb_arr, int* arr1, int n, int* arr2, int m) where comb_arr- the array used to combine arr1 and arr2 arr1 - the first array n - the length of the first array arr2 - the second array m - the length of the second array INITAL CODE TO BE COMPLETED #include using namespace std; void combine(int*, int*, int, int*, int); int main(void) { // Hey there, start typing your C++ code here... int size1, size2; cin >> size1; int arr1[size1]; for (int i = 0; i < size1; i++) { cin >> arr1[i]; } cin >> size2; int arr2[size2]; for (int i = 0; i < size2; i++) { cin >> arr2[i]; } int comb_size = ___; int comb_arr[comb_size]; combine(comb_arr, arr1, size1, arr2, size2); for (int i = 0; i < comb_size; i++) { printf("%d ", *(comb_arr+i)); } return 0; } // EXACT RUNNING TIME = ______ // BIG OH RUNNING TIME = ______ void combine(int* combine, int* arr1, int n, int* arr2, int m) { }
Write a function that will combine two arrays of different lengths into another array. Express the function's running time in terms of actual running time and Big-Oh notation.
For reference, this is the function declaration:
void combine(int* comb_arr, int* arr1, int n, int* arr2, int m)
where
- comb_arr- the array used to combine arr1 and arr2
- arr1 - the first array
- n - the length of the first array
- arr2 - the second array
- m - the length of the second array
INITAL CODE TO BE COMPLETED
#include <iostream>
using namespace std;
void combine(int*, int*, int, int*, int);
int main(void) {
// Hey there, start typing your C++ code here...
int size1, size2;
cin >> size1;
int arr1[size1];
for (int i = 0; i < size1; i++) {
cin >> arr1[i];
}
cin >> size2;
int arr2[size2];
for (int i = 0; i < size2; i++) {
cin >> arr2[i];
}
int comb_size = ___;
int comb_arr[comb_size];
combine(comb_arr, arr1, size1, arr2, size2);
for (int i = 0; i < comb_size; i++) {
printf("%d ", *(comb_arr+i));
}
return 0;
}
// EXACT RUNNING TIME = ______
// BIG OH RUNNING TIME = ______
void combine(int* combine, int* arr1, int n, int* arr2, int m) {
}

Step by step
Solved in 3 steps with 1 images

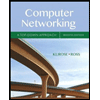
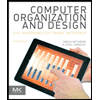
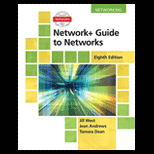
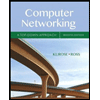
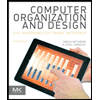
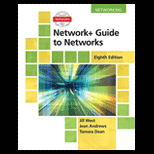
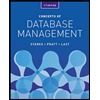
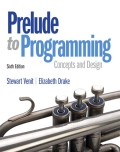
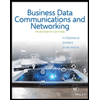