Write a Java program that computes how high the ball will be above the ground after x seconds in the above example? Where x is an input provided by the user. Step 1. Create an algorithm (either flowchart or pseudocode) that you will use to write the program. Place the algorithm in a Word document. Step 2. Code the program in Eclipse to compute the time. Inputs: The program should prompt the user for the height of the building, the initial speed, and the time the ball has traveled (i.e, flight time). The building height should be an integer variable, while the initial speed and flight time are double variables. Create a separate method from main to calculate the height of the ball. Name the separate method calcBallHeight and it needs to have three input arguments: height of building, initial speed, and flight time of the ball. The output of the calcBallHeight method will return a double, ballHeight, that is the height of the ball when the flight time has elapsed. Outputs: The program should have this output (below is an example): The ball will be feet above the ground after seconds of flight time. Where ballHeight, and flightTime must be formatted to two decimal places, e.g. 12.48, using a grintf statement. Add comments at the top of the class with your First and Last name. Step 3. Test your program with a height of 128 feet, an initial speed of 32 ft/sec, and a flight time of 3 seconds just like the example problem. Use the Snip It tool in Windows or a similar tool on the Mac to cut and paste the Eclipse Console output window into the same Word document as the algorithm in Step 1. C6 Project New [Java Application) C:\Program Files\Javaljdk-14.0.2\bin\java.exe (Oct 30, 2020 10:26:16 AM-10:26:29 AM) Enter the height of the building in feet as an integer: 128 Enter the init1 speed of the ball in ft/sec as a double: 32.0 Enter the ght time of the ball as a double: 3.0 The ball be 80.00 feet above the ground after 3.00 seconds of flight time.
Write a Java program that computes how high the ball will be above the ground after x seconds in the above example? Where x is an input provided by the user. Step 1. Create an algorithm (either flowchart or pseudocode) that you will use to write the program. Place the algorithm in a Word document. Step 2. Code the program in Eclipse to compute the time. Inputs: The program should prompt the user for the height of the building, the initial speed, and the time the ball has traveled (i.e, flight time). The building height should be an integer variable, while the initial speed and flight time are double variables. Create a separate method from main to calculate the height of the ball. Name the separate method calcBallHeight and it needs to have three input arguments: height of building, initial speed, and flight time of the ball. The output of the calcBallHeight method will return a double, ballHeight, that is the height of the ball when the flight time has elapsed. Outputs: The program should have this output (below is an example): The ball will be feet above the ground after seconds of flight time. Where ballHeight, and flightTime must be formatted to two decimal places, e.g. 12.48, using a grintf statement. Add comments at the top of the class with your First and Last name. Step 3. Test your program with a height of 128 feet, an initial speed of 32 ft/sec, and a flight time of 3 seconds just like the example problem. Use the Snip It tool in Windows or a similar tool on the Mac to cut and paste the Eclipse Console output window into the same Word document as the algorithm in Step 1. C6 Project New [Java Application) C:\Program Files\Javaljdk-14.0.2\bin\java.exe (Oct 30, 2020 10:26:16 AM-10:26:29 AM) Enter the height of the building in feet as an integer: 128 Enter the init1 speed of the ball in ft/sec as a double: 32.0 Enter the ght time of the ball as a double: 3.0 The ball be 80.00 feet above the ground after 3.00 seconds of flight time.
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 5RQ
Related questions
Question
![Chapter 6 Project
Project Name: Chpt6_Project
Class Name: Chpt6_Project
Projectile Motion
An object is thrown straight up from the top of a building that is h feet tall, with an initial velocity of v
feet per second. The height of the object as a function of time can be modeled by the function
h(t) = -16t² + vt + h, where h(t) is the height of the object (in feet) t seconds after it is thrown, and his
the starting height, i.e. the height of the building
This model assumes the object misses the top of the building on the way back down to the ground
and that wind resistance is minimal.
Example: Assume the ball is thrown straight up from the top of a 128 foot tall building with an initial speed
of 32 feet per second (ft/sec). The height of the ball as a function of time can be modeled by the function
by:
h(t) = -16t² + 32t + 128.
How high above the ground will the ball be after 3 seconds of flight time?
Note: t² equals t times t
h(t) = -16(3)² +32(3)+128
h(t) = -144 +96+ 128= 80 feet
The height of the ball will be 80 feet above the ground after 3 seconds of flight time.
Write a Java program that computes how high the ball will be above the ground after x seconds in the
above example? Where x is an input provided by the user.
Step 1. Create an algorithm (either flowchart or pseudocode) that you will use to write the program. Place
the algorithm in a Word document.
Step 2. Code the program in Eclipse to compute the time.
Inputs: The program should prompt the user for the height of the building, the initial speed, and the time
the ball has traveled (i.e. flight time). The building height should be an integer variable, while the initial
speed and flight time are double variables.
Create a separate method from main to calculate the height of the ball.
Name the separate method calcBallHeight and it needs to have three input arguments: height of
building, initial speed, and flight time of the ball. The output of the calcBallHeight method will
return a double, ballHeight, that is the height of the ball when the flight time has elapsed.
Outputs: The program should have this output (below is an example):
The ball will be <ballHeight> feet above the ground after <flightTime> seconds of flight time.
Where ballHeight and flightTime must be formatted to two decimal places, e.g. 12.48, using a printf
statement.
Add comments at the top of the class with your First and Last name.
Step 3. Test your program with a height of 128 feet, an initial speed of 32 ft/sec, and a flight time of 3
seconds just like the example problem. Use the Snip It tool in Windows or a similar tool on the Mac to cut
and paste the Eclipse Console output window into the same Word document as the algorithm in Step 1.
<terminated > C6_Project_New [Java Application] C:\Program Files\Java\jdk-14.0.2\bin\javaw.exe (Oct 30, 2020 10:26:16 AM - 10:26:29 AM)
Enter the height of the building in feet as an integer: 128
Enter the initial speed of the ball in ft/sec as a double: 32.0
Enter
The balight time of the ball as a double: 3.0
The ball be 80.00 feet above the ground after 3.00 seconds of flight time.
Step 4. Now vary the flight time of the ball in 5 second increments to see how high it will go up before it
starts to come down. Show that output as well. You will have to try different flight times to get close to
the maximum height. Keep in mind the height of the ball will increase until it reaches the top of the curve
then it will start coming down. What is the maximum height the ball will travel?](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2f3cf72c-601d-4153-9d70-95173bcd1276%2F9b0b8da6-83ac-4ee1-a88b-26e2b3f80a0c%2Fntwljrk_processed.png&w=3840&q=75)
Transcribed Image Text:Chapter 6 Project
Project Name: Chpt6_Project
Class Name: Chpt6_Project
Projectile Motion
An object is thrown straight up from the top of a building that is h feet tall, with an initial velocity of v
feet per second. The height of the object as a function of time can be modeled by the function
h(t) = -16t² + vt + h, where h(t) is the height of the object (in feet) t seconds after it is thrown, and his
the starting height, i.e. the height of the building
This model assumes the object misses the top of the building on the way back down to the ground
and that wind resistance is minimal.
Example: Assume the ball is thrown straight up from the top of a 128 foot tall building with an initial speed
of 32 feet per second (ft/sec). The height of the ball as a function of time can be modeled by the function
by:
h(t) = -16t² + 32t + 128.
How high above the ground will the ball be after 3 seconds of flight time?
Note: t² equals t times t
h(t) = -16(3)² +32(3)+128
h(t) = -144 +96+ 128= 80 feet
The height of the ball will be 80 feet above the ground after 3 seconds of flight time.
Write a Java program that computes how high the ball will be above the ground after x seconds in the
above example? Where x is an input provided by the user.
Step 1. Create an algorithm (either flowchart or pseudocode) that you will use to write the program. Place
the algorithm in a Word document.
Step 2. Code the program in Eclipse to compute the time.
Inputs: The program should prompt the user for the height of the building, the initial speed, and the time
the ball has traveled (i.e. flight time). The building height should be an integer variable, while the initial
speed and flight time are double variables.
Create a separate method from main to calculate the height of the ball.
Name the separate method calcBallHeight and it needs to have three input arguments: height of
building, initial speed, and flight time of the ball. The output of the calcBallHeight method will
return a double, ballHeight, that is the height of the ball when the flight time has elapsed.
Outputs: The program should have this output (below is an example):
The ball will be <ballHeight> feet above the ground after <flightTime> seconds of flight time.
Where ballHeight and flightTime must be formatted to two decimal places, e.g. 12.48, using a printf
statement.
Add comments at the top of the class with your First and Last name.
Step 3. Test your program with a height of 128 feet, an initial speed of 32 ft/sec, and a flight time of 3
seconds just like the example problem. Use the Snip It tool in Windows or a similar tool on the Mac to cut
and paste the Eclipse Console output window into the same Word document as the algorithm in Step 1.
<terminated > C6_Project_New [Java Application] C:\Program Files\Java\jdk-14.0.2\bin\javaw.exe (Oct 30, 2020 10:26:16 AM - 10:26:29 AM)
Enter the height of the building in feet as an integer: 128
Enter the initial speed of the ball in ft/sec as a double: 32.0
Enter
The balight time of the ball as a double: 3.0
The ball be 80.00 feet above the ground after 3.00 seconds of flight time.
Step 4. Now vary the flight time of the ball in 5 second increments to see how high it will go up before it
starts to come down. Show that output as well. You will have to try different flight times to get close to
the maximum height. Keep in mind the height of the ball will increase until it reaches the top of the curve
then it will start coming down. What is the maximum height the ball will travel?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
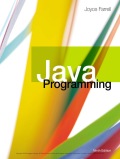
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
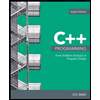
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
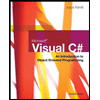
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
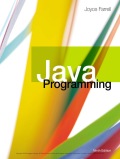
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
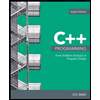
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
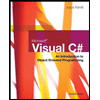
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,