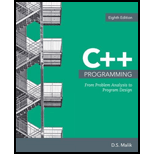
Concept explainers
Using classes, design an online address book to keep track of the names, addresses, phone numbers, and dates of birth of family members, close friends, and certain business associates. Your program should be able to handle a maximum of 500 entries.
Define a class addressType that can store a street address, city, state, and ZIP code. Use the appropriate functions to print and store the address. Also, use constructors to automatically initialize the member variables.
Define a class extPersonType using the class personType (as defined in Example 10-10, Chapter 10), the class dateType (as designed in this chapters
Programming Exercise 2), and the class addressType. Add a member variable to this class to classify the person as a family member, friend, or business associate. Also, add a member variable to store the phone number. Add (or override) the functions to print and store the appropriate information. Use constructors to automatically initialize the member variables.Define the class addressBookType using the previously defined classes. An object of the type addressBookType should be able to process a maximum of 500 entries.
The program should perform the following operations:
Load the data into the address book from a disk.
Sort the address book by last name.
Search for a person by last name.
Print the address, phone number, and date of birth (if it exists) of a given person.
Print the names of the people whose birthdays are in a given month.
Print the names of all the people between two last names.
Depending on the user’s request, print the names of all family members, friends, or business associates.

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
C++ Programming: From Problem Analysis to Program Design
- Java Question - Using the bottom code, create another class named "DigitalBook" as a subclass of the given "Book" class. Then, Make sure that the "DigitalBook" class contains two constructors: (1) one is the default constructor that takes no parameter, and (2) the other is a parameterized constructor that take 6 parameters. Be sure to use the “super” keyword in the constructors of the "DigitalBook" class. In the “main()” method of the “EX12_1” class, create four instances: “b1”, “b2”, d1”, and “d2”. Let “b1” and “b2” be the instances of the “Book” class, and let the “d1” and “d2” be the instance of the “DigitalBook” class. Be sure to use the specified constructor (as shown in attached picture) to initialize these instances. The Final Output is shown in the attached pictures. Please make sure that no errors show up in the Java Compiler when testing the code. Thank you. Add the following code to the "class Book" code (Below the ___ line). public class EX12_1{public static void…arrow_forwardJava Question - Using the bottom code, create another class named "DigitalBook" as a subclass of the given "Book" class. Then, Make sure that the "DigitalBook" class contains two constructors: (1) one is the default constructor that takes no parameter, and (2) the other is a parameterized constructor that take 6 parameters. Be sure to use the “super” keyword in the constructors of the "DigitalBook" class. In the “main()” method of the “EX12_1” class, create four instances: “b1”, “b2”, d1”, and “d2”. Let “b1” and “b2” be the instances of the “Book” class, and let the “d1” and “d2” be the instance of the “DigitalBook” class. Be sure to use the specified constructor (as shown in attached picture) to initialize these instances. The Final Output is shown in the attached pictures. Add the following code to the "class Book" code (Below the ___ line). public class EX12_1{public static void main(String[] args){/////add your code here}}…arrow_forwardThese two pictures are my resource and driver classes. Please, help me with the error in my resource class. I want my resource class to have getName, getSalary, getThePercentageThatTheEmployeeWantToRaise, and calculateTheNewSalary methods. For my driver class, I want the driver class to ask the user to input their name, salary, how much they want to raise, and print out their new salary.arrow_forward
- Why do we need encapsulation? What happens if data is not encapsulated within a class? Is there a way to access data which is encapsulated in a class? Give examples to encapsulate data within class and package. There is an online banking system where a user can view his contact info, account summary, add few beneficiaries account details. The bank officer can also view user's contact info, account summary, ATM card charges, bank charges, and other deductions. Create a class Customer and define its attributes and method names with proper access modifiers i.e. public, private or default.arrow_forwardPrompt We have learned how to use accessor and mutator methods to access private class member data. It is possible to instead make the class members public, which would allow other programs to directly retrieve and modify the class member data without needing to write the accessor and mutator methods. This is usually considered bad practice. Why do you think that is? What do you think the benefits are to writing accessor and mutator methods instead of just leaving the variables public? Below is the definition for a class called Counter. Define a new method for this class called "findDifference". This method should take another Counter object as an argument and return the difference in the counts between the counter being called and the one passed as an argument. The difference should be given as an absolute value (not returned as a negative). See below the class definition for examples of this method being used. public class Counter {private int count;public Counter() {count =…arrow_forwardCan you help me with this please: Write the definition of a class swimmingPool, to implement the properties of a swimming pool. Your class should have the instance variables to store the length (in feet), width (in feet), depth (in feet), the rate (in gallons per minute) at which the water is filling the pool, and the rate (in gallons per minute) at which the water is draining from the pool. Add appropriate constructors to initialize the instance variables. Also, add member functions to do the following: determine the amount of water needed to fill an empty or partially filled pool, determine the time needed to completely or partially fill or empty the pool, and add or drain water for a specific amount of time, if the water in the pool exceeds the total capacity of the pool, output "Pool overflow" to indicate that the water has breached capacity. The header file for the swimmingPool class has been provided for reference. Write a program to test your swimmingPool class. An example of…arrow_forward
- I have no idea what to do. Modify the FitnessTracker class, created in Chapter 4 Programming Exercise 3AB, so that the default constructor calls the three-parameter constructor. Use TestFitnessTracker2 to test the new version of the class. import java.time.*;public class FitnessTracker2 {String activity;int minutes;LocalDate date;public FitnessTracker2() {}public FitnessTracker2(String activityParam, int minutesParam, LocalDate dateParam) {}public String getActivity() {}public int getMinutes() {}public LocalDate getDate() {}} import java.time.*;public class TestFitnessTracker2{public static void main(String[] args){ FitnessTracker2 exercise = new FitnessTracker2(); System.out.println(exercise.getActivity() + " " + exercise.getMinutes() +" minutes on " + exercise.getDate()); // code to test constructor added for exercise 3bLocalDate date = LocalDate.of(2020, 8, 20);FitnessTracker2 exercise2 = new FitnessTracker2("bicycling", 35, date); System.out.println(exercise2.getActivity() + " " +…arrow_forwardCreate two classes of your choice (a parent and a child) and access their properties as shownabovearrow_forwardPlease, I want to modify the code so that the user can add the employee's name, number and specialization, and use for loob He must add more than one employee. When he finishes adding the employee’s data, he asks the user: Do you want 1-Print the data 2-Add a new employee 3-Exit class Doctor:"""Represents a Doctor""" #initializer with specialization default to "general"def __init__(self, Id, name, specialization="general"):#attributesself.Id = Idself.name = nameself.specialization = specialization.lower() #initializing salary to 25000, basic salaryself.salary = 25000 #incrementing salary based on specialization#if specialization is pediatric, increasing salary by 10%if specialization == "pediatric":self.salary += self.salary*10/100 #if specialization is dental, increasing salary by 15%elif specialization == "dental":self.salary += self.salary*15/100 #str() functiondef __str__(self):return (f"Id: {self.Id} \n"f"Name: {self.name} \n"f"Specialization: {self.specialization} \n"f"Salary:…arrow_forward
- Hi, I am making a elevator simulation and I need help in making the passenger classes using polymorphism, I am not sure what to implement in the comment that says logic. Any help is appreciated. There are 4 types of passengers in the system:Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements.VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators.Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators.Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. This is what i have so far public abstract class Passenger { protected String type; protected int…arrow_forwardTake the tax program from the last homework assignment and implement it using classes and objects instead. To do so, create a class definition for a class called Customer with attributes income and tax. It should also have a set method for income, and a calcTax() method to assign tax and return it. As a reminder, the tax is calculated as follows: Income Tax Due $0 - $50,000 5% $50,000-$100,000 $2,500 + 10% of (income > $50,000) > $100,000 $7,500 + 15% of (income > $100,000) Create a Customer object in main, have the user enter the income and assign this to the income variable of the Customer object, and then call the calcTax() method for the Customer object and print the tax due.arrow_forwardHi can you assist me on the problem below for a class and a test class that conforms to the following specifications: 1. The name of the class is RightTriangle 2. The class has member variables base and height 3. The class has a constructor that initializes base and height 4. The class has accessors and mutators for base and height 5. The class has a toString method that produces String representation of a RightTriangle like the following example for a triangle with base 12 and height 10. Base : 12 Height 10 6. The class has a method called findArea to compute the area of the right triangle. Here is a suggested implementation. public double findArea() { return 0.5* this.base*this.height; } 7. The class using proper naming conventions Write a test class called RightTriangleTest that conforms to the following: 1. Create an object with base = 10 and height = 20, for example myTriangle. 2. To print the toString for myTriangle. 3. To print the area of myTriangle. System.out.println(“The…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
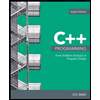