Write a program by C# programming language that has the followings: static void Main(string[] args) { try { // Declare two integer variables named as "row" and "col" // -------------------------------------- // Get the values of the "row" and "col" from the user // -------------------------------------- // if the value of the "row" is less than 1, catch part will be run // -------------------------------------- // if the value of the "col" is less than 1, catch part will be run // -------------------------------------- // Declare the two dimensional integer array "matrix" // which has the size (row x col) // -------------------------------------- // Create the StreamWriter object named as "sw". // "sw" will reference "myFile.txt" // -------------------------------------- // Call "CreateRandomNumbers" method // to assign random values to each element of "matrix" array // -------------------------------------- // Call "WriteToFile" method. // -------------------------------------- // Create the StreamReader object named as "sr". // "sr" will read all content of "myFile.txt" // and the content will be displayed at Console window. } catch (Exception) { // ……………………………………………………………….. } } public static void CreateRandomNumbers(int[,] m) { // Create random numbers for each element of the "m" array. // Random values will be between 10 and 100 } public static void WriteToFile(StreamWriter w, int[,] m) { // Write the elements of the "m" array in the matrix format // to the file "myFile.txt". // -------------------------------------- // Call the "AverageOfAllElements" method and // write the returned value after the text // "The average of all elements of the matrix:" in the file "myFile.txt". // -------------------------------------- // Put the header "LIST OF ODD NUMBERS IN EACH ROW:" in the file "myFile.txt". // Call the "WriteOddNumbersOfEachRow" method. // -------------------------------------- // Put the header "LIST OF EVEN NUMBERS IN EACH COLUMN" in the file "myFile.txt" // Call the "WriteEvenNumbersOfEachColumn" method. } public static double AverageOfAllElements(int[,] m) { // ……………………………………………………………….. } public static void WriteOddNumbersOfEachRow(StreamWriter w, int[,] m) { // Write the odd numbers in each row of the "m" array // to the "myFile.txt" as in the output example. } public static void WriteEvenNumbersOfEachColumn(StreamWriter w, int[,] m) { // Write the even numbers in each column of the "m" array // to the "myFile.txt" as in the output example. }
Write a program by C# programming language that has the followings:
static void Main(string[] args)
{
try
{
// Declare two integer variables named as "row" and "col"
// --------------------------------------
// Get the values of the "row" and "col" from the user
// --------------------------------------
// if the value of the "row" is less than 1, catch part will be run
// --------------------------------------
// if the value of the "col" is less than 1, catch part will be run
// --------------------------------------
// Declare the two dimensional integer array "matrix"
// which has the size (row x col)
// --------------------------------------
// Create the StreamWriter object named as "sw".
// "sw" will reference "myFile.txt"
// --------------------------------------
// Call "CreateRandomNumbers" method
// to assign random values to each element of "matrix" array
// --------------------------------------
// Call "WriteToFile" method.
// --------------------------------------
// Create the StreamReader object named as "sr".
// "sr" will read all content of "myFile.txt"
// and the content will be displayed at Console window.
}
catch (Exception)
{
// ………………………………………………………………..
}
}
public static void CreateRandomNumbers(int[,] m)
{
// Create random numbers for each element of the "m" array.
// Random values will be between 10 and 100
}
public static void WriteToFile(StreamWriter w, int[,] m)
{
// Write the elements of the "m" array in the matrix format
// to the file "myFile.txt".
// --------------------------------------
// Call the "AverageOfAllElements" method and
// write the returned value after the text
// "The average of all elements of the matrix:" in the file "myFile.txt".
// --------------------------------------
// Put the header "LIST OF ODD NUMBERS IN EACH ROW:" in the file "myFile.txt".
// Call the "WriteOddNumbersOfEachRow" method.
// --------------------------------------
// Put the header "LIST OF EVEN NUMBERS IN EACH COLUMN" in the file "myFile.txt"
// Call the "WriteEvenNumbersOfEachColumn" method.
}
public static double AverageOfAllElements(int[,] m)
{
// ………………………………………………………………..
}
public static void WriteOddNumbersOfEachRow(StreamWriter w, int[,] m)
{
// Write the odd numbers in each row of the "m" array
// to the "myFile.txt" as in the output example.
}
public static void WriteEvenNumbersOfEachColumn(StreamWriter w, int[,] m)
{
// Write the even numbers in each column of the "m" array
// to the "myFile.txt" as in the output example.
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

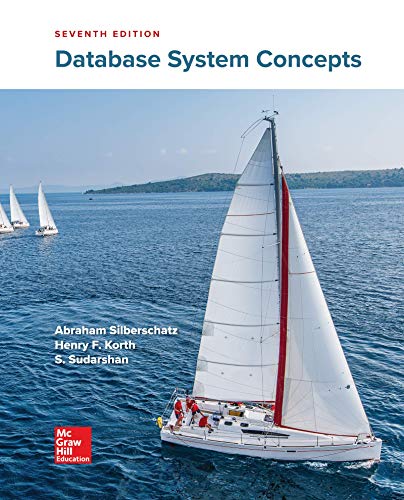
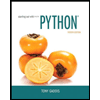
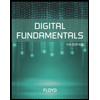
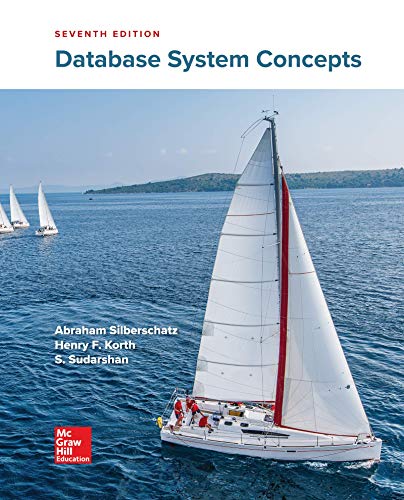
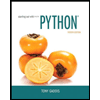
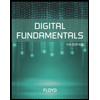
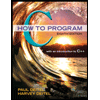
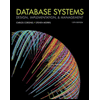
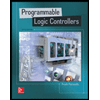