Write a program called gcd.java that repeatedly asks the user to input two integer numbers and print out those numbers' greatest common divisor. Computing and printing the GCD should be done in a function named calculate_gcd that takes the two numbers received from the user as input parameters. Your program should ask the user if they want to end; otherwise, continue asking for the two numbers and printing the results. Here is a part of the program below. Complete the code where the comment states to. The output is also included.
Write a program called gcd.java that repeatedly asks the user to input two integer numbers and print out those numbers' greatest common divisor. Computing and printing the GCD should be done in a function named calculate_gcd that takes the two numbers received from the user as input parameters. Your program should ask the user if they want to end; otherwise, continue asking for the two numbers and printing the results. Here is a part of the program below. Complete the code where the comment states to. The output is also included.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a
Here is a part of the program below. Complete the code where the comment states to. The output is also included.
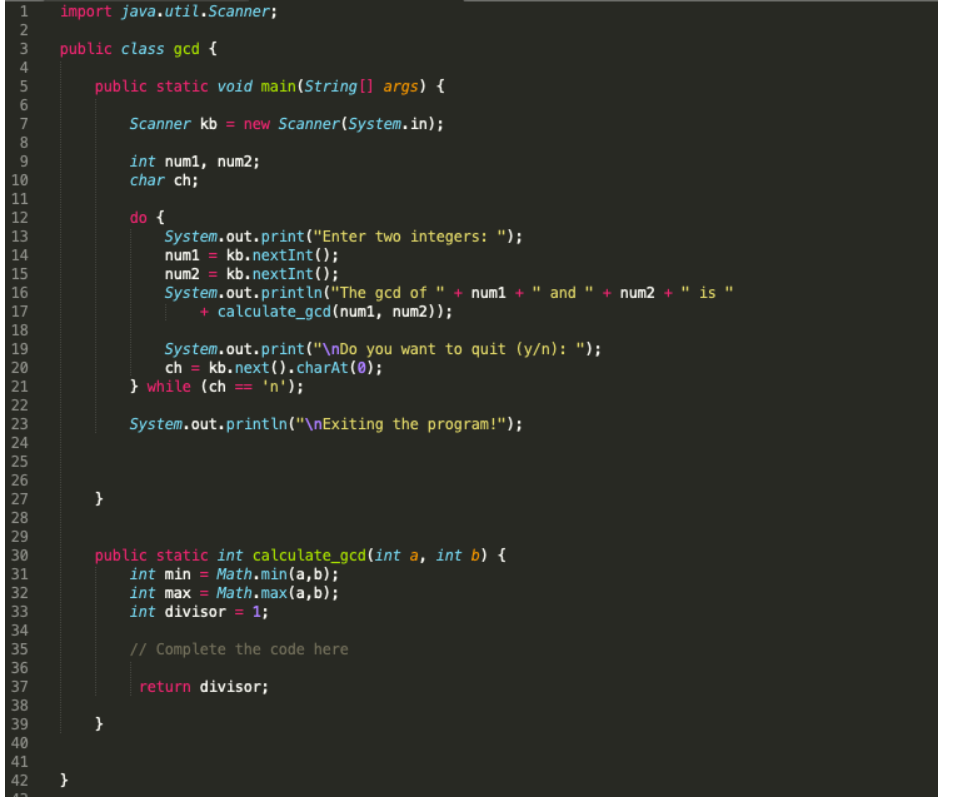
Transcribed Image Text:import java.util.Scanner;
public class gcd {
public static void main(String[ args) {
Scanner kb = new Scanner(System.in);
int num1, num2;
char ch;
10
11
| 12
do {
System.out.print("Enter two integers: ");
num1 = kb.nextInt();
num2 = kb.nextInt();
System.out.println("The gcd of " + num1 + " and " + num2 + " is "
+ calculate_gcd(num1, num2));
13
| 14
15
%3D
16
| 17
18
System.out.print("\nDo you want to quit (y/n): ");
ch = kb.next().charAt(0);
} while (ch
19
| 20
21
'n');
22
System.out.print ln("\nExiting the program!");
23
24
|25
26
|27
}
28
| 29
30
31
32
33
34
35
36
37
38
public static int calculate_gcd(int a, int b) {
int min = Math.min(a,b);
int max = Math. max(a,b);
int divisor = 1;
// Complete the code here
return divisor;
39
}
| 40
42 }
H23 4567 89
344 4
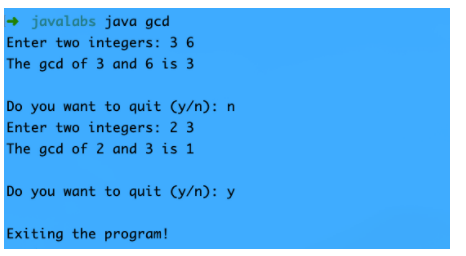
Transcribed Image Text:javalabs java gcd
Enter two integers: 3 6
The gcd of 3 and 6 is 3
Do you want to quit (y/n): n
Enter two integers: 2 3
The gcd of 2 and 3 is 1
Do you want to quit (y/n): y
Exiting the program!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
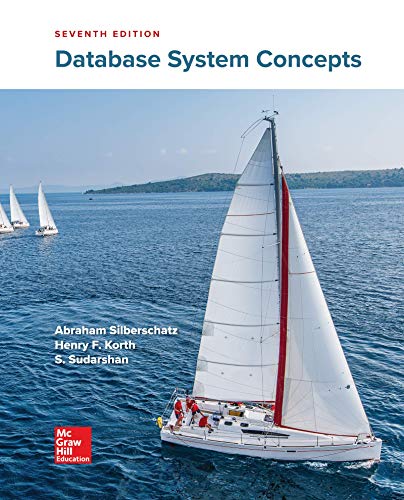
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
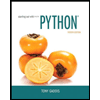
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
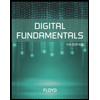
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
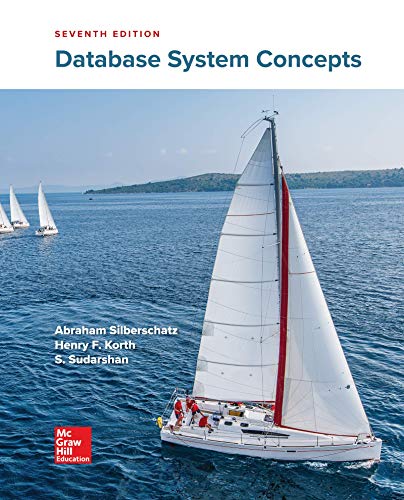
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
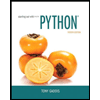
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
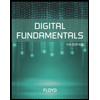
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
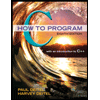
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
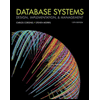
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
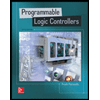
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education