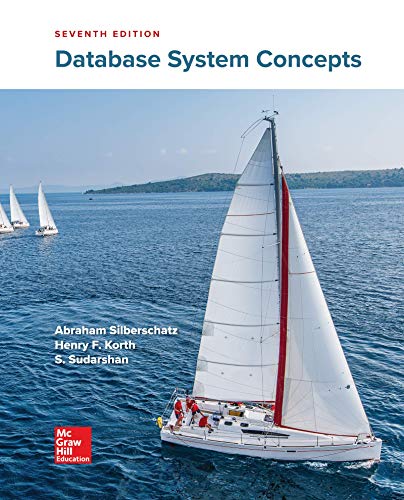
Concept explainers
import java.util.Scanner;
public class LabProgram {
public static void main(String args[]) {
Scanner scnr = new Scanner(System.in);
int credits;
int seed;
GVDie die1, die2;
die1 = new GVDie();
die2 = new GVDie();
// Read random seed to support testing (do not alter)
seed = scnr.nextInt();
die1.setSeed(seed);
// Read starting credits
credits = scnr.nextInt();
int rounds = 0;
while (credits > 0) {
// Step 1: Roll both dice
die1.roll();
die2.roll();
int total = die1.getValue() + die2.getValue();
if (total == 7 || total == 11) {
// Player wins one credit
credits++;
// UPDATE - print the dice total here
System.out.println("Dice total: " + total);
//UPDATE - break the loop and end the round
break;
} else if (total == 2 || total == 3 || total == 12) {
// Player loses one credit
credits--;
// UPDATE - print the dice total here
System.out.println("Dice total: " + total);
//UPDATE - break the loop and end the round
break;
} else {
// Set the goal for future rolls
int goal = total;
// UPDATE - print the dice total here
System.out.println("Dice total: " + total);
// Step 2: Continue rolling until goal or 7 is rolled
while (true) {
die1.roll();
die2.roll();
total = die1.getValue() + die2.getValue();
if (total == 7) {
// Player loses one credit
credits--;
break;
} else if (total == goal) {
// Player wins one credit
credits++;
break;
}
}
// set goal to -1 after the round ends.
goal = -1;
}
}
System.out.print("Credits: " + credits);
}
}
import java.util.*;
public class GVDie implements Comparable <GVDie> {
// Static members are shared across all instances of class GVDie
private static Random rand = new Random();
private int myValue;
// Set initial die value
public GVDie() {
myValue = rand.nextInt(6) + 1;
}
// Roll the die to get 1 - 6
public void roll () {
myValue = rand.nextInt(6) + 1;
}
// Return current die value
public int getValue() {
return myValue;
}
// Set the random number generator seed to support testing
public void setSeed(int seed) {
rand.setSeed(seed);
}
// Allows dice to be compared if necessary
public int compareTo(GVDie d) {
return getValue() - d.getValue();
}
}
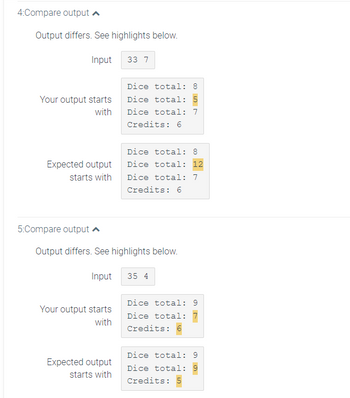
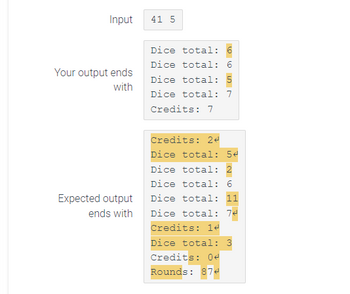

Step by stepSolved in 4 steps with 4 images

- public class SeperateDuplicates { public static void main(String[ args) { System.out.printIn(seperateDuplicatesChars("Hello")); System.out.printin(seperateDuplicatesChars ("Bookkeeper"')); System.out.printin(seperateDuplicatesChars("Yellowwood door"')); System.out.printin(seperateDuplicatesChars("Chicago Cubs")); */ public static String seperateDuplicatesChars(String str) { //To be completed } }arrow_forwardLab 9 C balance the same, y, n, why? class CheckingAct { . . . . private int balance; public void processCheck( int amount ) { int charge; if ( balance < 100000 ) charge = 15; else charge = 0; balance = balance - amount - charge ; // change the local copy of the value in "amount" amount = 0 ; } } public class CheckingTester { public static void main ( String[] args ) { CheckingAct act; int check = 5000; act = new CheckingAct( "123-345-99", "Your Name", 100000 ); System.out.println( "check:" + check ); // prints "5000" // call processCheck with a copy of the value 5000 act.processCheck( check ); System.out.println( "check:" + check ); // prints "5000" --- "check" was not changed } }arrow_forwardStatement that increases numPeople by 5. Ex: If numPeople is initially 10, the output is: There are 15 people.arrow_forward
- Javaarrow_forwardHere is a statement: class temporary { public: void set(string, double, double); void print(); double manipulate(); void get(string&, double&, double&); void setDescription(string); void setFirst(double); void setSecond(double); string getDescription() const; double getFirst()const; double getSecond()const; temporary(string = "", double = 0.0, double = 0.0); private: string description; double first; double second; }; How do I write the definition of the member function set so that the instance variables are set according to the parameters?arrow_forwardpublic class arrayOutput { public static void main (String [] args) { final int NUM_ELEMENTS = 3; int[] userVals = new int [NUM_ELEMENTS]; int i; int sumVal; userVals [0] = 3; userVals [1] = 5; userVals [2] = 8; Type the program's output sumVal = 0; for (i = 0; i < userVals.length; ++i) { sumVal= sumVal + userVals [i]; System.out.println (sumVal); } ? ? ??arrow_forward
- public class KnowledgeCheckTrek { public static final String TNG = "The Next Generation"; public static final String DS9 = "Deep Space Nine"; public static final String VOYAGER = "Voyager"; public static String trek(String character) { if (character != null && (character.equalsIgnoreCase("Picard") || character.equalsIgnoreCase("Data"))) { // IF ONE return TNG; } else if (character != null && character.contains("7")) { // IF TWO return VOYAGER; } else if ((character.contains("Quark") || character.contains("Odo"))) { // IF THREE return DS9; } return null; } public static void main(String[] args) { System.out.println(trek("Captain Picard")); System.out.println(trek("7 of Nine")); System.out.println(trek("Odo")); System.out.println(trek("Quark")); System.out.println(trek("Data").equalsIgnoreCase(TNG));…arrow_forwardclass temporary { public: void set(string, double, double); void print(); double manipulate(); void get(string&, double&, double&); void setDescription(string); void setFirst(double); void setSecond(double); string getDescription() const; double getFirst()const; double getSecond()const; temporary(string = "", double = 0.0, double = 0.0); private: string description; double first; double second; }; I need help writing the definition of the member function set so the instance varialbes are set according to the parameters. I also need help in writing the definition of the member function manipulation that returns a decimal with: the value of the description as "rectangle", returns first * second; if the value of description is "circle", it returns the area of the circle with radius first; if the value of the description is "cylinder", it returns the volume of the cylinder with radius first and height second; otherwise, it returns with the value -1.arrow_forwardusing System; class main { publicstaticvoid Main(string[] args) { int number = 1; while (number <= 88) { int i; Random r = new Random(); int[] randNo=newint[3]; for(i=0;i<3;i++) { randNo[i]= r.Next(1,88); Console.WriteLine("Random Number between 1 and 88 is "+randNo[i]); } } } } This code is supposed to count from 1-88 and then select three random numbers from the list but instead it generates an infinite loop of random numers between 1-88. how can it be fixed?arrow_forward
- import java.util.Scanner;/** This program computes the time required to double an investment with an annual contribution.*/public class DoubleInvestment{ public static void main(String[] args) { final double RATE = 5; final double INITIAL_BALANCE = 10000; final double TARGET = 2 * INITIAL_BALANCE; Scanner in = new Scanner(System.in); System.out.print("Annual contribution: "); double contribution = in.nextDouble(); double balance = INITIAL_BALANCE; int year = 0; // TODO: Add annual contribution, but not in year 0 do { year++; double interest = balance*(RATE/100); balance = (balance+contribution+interest); } while(balance< TARGET); System.out.println("Year: " + year); System.out.printf("Balance: %.2f%n", balance); }}arrow_forwardComplete the isExact Reverse() method in Reverse.java as follows: The method takes two Strings x and y as parameters and returns a boolean. The method determines if the String x is the exact reverse of the String y. If x is the exact reverse of y, the method returns true, otherwise, the method returns false. Other than uncommenting the code, do not modify the main method in Reverse.java. Sample runs provided below. Argument String x "ba" "desserts" "apple" "regal" "war" "pal" Argument String y "stressed" "apple" "lager" "raw" "slap" Return Value false true false true true falsearrow_forwardint stop = 6; int num =6; int count=0; for(int i = stop; i >0; i-=2) { num += i; count++; } System.out.println("num = "+ num); System.out.println("count = "+ count); } }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
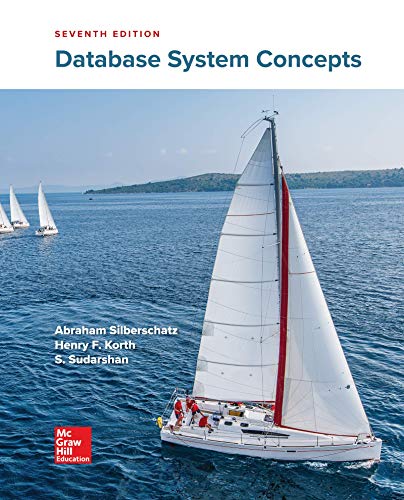
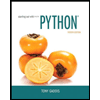
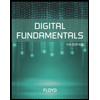
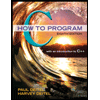
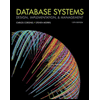
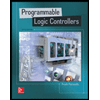