Write a program in C++ to help a teacher to automate her grading system. Your program will have the following: 1 - A struct named studentInfo with the following members: • firstName, variable of type string • lastName, variable of type string • ID, variable of type string 2 - A struct named courseGrade with the following members: • total, variable of type int • Final, variable of type int • sum, variable of type int 3- A struct named student with the following members: • info, variable of type studentInfo • grade, variable of type courseGrade • status, variable of type bool
Write a
1 - A struct named studentInfo with the following members:
• firstName, variable of type string
• lastName, variable of type string
• ID, variable of type string
2 - A struct named courseGrade with the following members:
• total, variable of type int
• Final, variable of type int
• sum, variable of type int
3- A struct named student with the following members:
• info, variable of type studentInfo
• grade, variable of type courseGrade
• status, variable of type bool
4 - A function inputData to prompt the user to enter the information of the student. The function accepts one parameter of type student.
5 - A function computeSum to compute and set the total of all grades (total+final). The function accepts one parameter of type courseGrade.
6 - A function setStatus to set the status of the course. If the sum>=50 then, the student passed the course else, the student failed. The function accepts one parameter of type student.
7- A function print to print all information of the class student. The function accepts one parameter of type array of student.
8 - Your main should perform the following:
• Declare an array std of type student. Use size 3.
• Fill the information of the std array by calling inputData Function
• Call computeSum Function for all elements of the std array
• Call setStatus Function for all elements of the std array
• Call print Function for all elements of the std array
Constraints
None
Input Format
The input will be as following:
First name, last name, ID, total of 60 and final grade
Output Format
As in the sample
Sample #1
Input
Omar Khaled 12345 40 20
Sara Ali 12346 55 35
Rashed Kamal 12347 20 15
Output
Omar Khaled 12345 60 Pass
Sara Ali 12346 90 Pass
Rashed Kamal 12347 35 Fail

Step by step
Solved in 3 steps

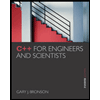
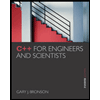