Given the cost for decoration, Hall expenses, and food. Write a c++ program to check if it is within the budget. Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. Create separate classes in separate files. The class Wedding has the following public data members. Data Type Variable Name string brideName string brideGroomName string venue string date int numberOfDays float budget The class WeddingHall has the following public data members. Data Type Variable Name float costPerHour A method in the WeddingHall class Method Member Function float calculateWeddingHallCost(int noOfDays,float costPerHour) To calculate the cost of wedding hall . Note : For Example: If Number of days =1 Cost for WeddingHall = 24*costPerHour The class Decoration has the following public data members. Data Type Variable Name float flowerCost float goldFoilCost float backDropCost A method in the Decoration class Method Member Function float calculateDecorationCost(float flowerCost,float goldFoilCost,float backDropCost) To calculate the cost for decoration. Note : Cost for Decoration = flowerCost+ goldFoilCost+backDropCost The class Food has the following public data members. Data Type Variable Name float breakFastCost float lunchCost float dinnerCost A method in the Food class Method Member Function float calculateFoodCost(float breakFastCost, float lunchCost, float dinnerCost, int noOfDays) To calculate the cost for food. Note : Cost for food = number of days*(breakFastCost+ lunchCost + dinnerCost) Total wedding budget = Weddinghall cost +decoration cost +food cost Reading inputs, displaying outputs, and comparing total with a budget should be in main. [All text in bold are input and the remaining are output] Sample Input and Output 1: Enter the BrideName : Thara K.S Enter the BrideGroomName : Varun K Enter the venue of the wedding : SNV Mahal Enter the Date : 23/10/2016 Enter the Number of days : 1 Enter the cost of wedding hall per hour : 9000 Enter the flowerCost : 2400 Enter the goldFoilCost : 2000 Enter the backDropCost : 30000 Enter the Break Fast Cost : 20000 Enter the Lunch Cost : 35000 Enter the Dinner Cost : 10000 Enter the Budget : 400000 Cost for Wedding Hall :216000 Cost for Decoration :34400 Cost for Food :65000 Total cost :315400 The Estimate is within the Budget! Sample Input and Output 2: Enter the BrideName : Preethika D Enter the BrideGroomName : Vignesh M Enter the venue of the wedding : ShriDevi Mahal Enter the Date : 23/09/2016 Enter the Number of days : 2 Enter the cost of wedding hall per hour : 3000 Enter the flowerCost : 4000 Enter the goldFoilCost : 5000 Enter the backDropCost : 20000 Enter the Break Fast Cost : 35000 Enter the Lunch Cost : 50000 Enter the Dinner Cost : 20000 Enter the Budget : 300000 Cost for Wedding Hall :144000 Cost for Decoration :29000 Cost for Food :210000 Total cost :383000 The Estimate exceeds the Budget!
KINDLY SOLVE SOON THE NEED FOR HOMEWORK. AND MATCH INPUT AND OUTPUT AS IT IS.
----------------------------------------------------------------------
Given the cost for decoration, Hall expenses, and food. Write a c++ program to check if it is within the budget.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. Create separate classes in separate files.
The class Wedding has the following public data members.
Data Type | Variable Name |
string | brideName |
string | brideGroomName |
string | venue |
string | date |
int | numberOfDays |
float | budget |
The class WeddingHall has the following public data members.
Data Type | Variable Name |
float | costPerHour |
A method in the WeddingHall class
Method |
Member Function |
float calculateWeddingHallCost(int noOfDays,float costPerHour) |
To calculate the cost of wedding hall . |
Note :
For Example: If Number of days =1
Cost for WeddingHall = 24*costPerHour
The class Decoration has the following public data members.
Data Type | Variable Name |
float | flowerCost |
float | goldFoilCost |
float | backDropCost |
A method in the Decoration class
Method |
Member Function |
float calculateDecorationCost(float flowerCost,float goldFoilCost,float backDropCost) |
To calculate the cost for decoration. |
Note : Cost for Decoration = flowerCost+ goldFoilCost+backDropCost
The class Food has the following public data members.
Data Type | Variable Name |
float | breakFastCost |
float | lunchCost |
float | dinnerCost |
A method in the Food class
Method |
Member Function |
float calculateFoodCost(float breakFastCost, float lunchCost, float dinnerCost, int noOfDays) |
To calculate the cost for food. |
Note :
Cost for food = number of days*(breakFastCost+ lunchCost + dinnerCost)
Total wedding budget = Weddinghall cost +decoration cost +food cost
Reading inputs, displaying outputs, and comparing total with a budget should be in main.
[All text in bold are input and the remaining are output]
Sample Input and Output 1:
Enter the BrideName :
Thara K.S
Enter the BrideGroomName :
Varun K
Enter the venue of the wedding :
SNV Mahal
Enter the Date :
23/10/2016
Enter the Number of days :
1
Enter the cost of wedding hall per hour :
9000
Enter the flowerCost :
2400
Enter the goldFoilCost :
2000
Enter the backDropCost :
30000
Enter the Break Fast Cost :
20000
Enter the Lunch Cost :
35000
Enter the Dinner Cost :
10000
Enter the Budget :
400000
Cost for Wedding Hall :216000
Cost for Decoration :34400
Cost for Food :65000
Total cost :315400
The Estimate is within the Budget!
Sample Input and Output 2:
Enter the BrideName :
Preethika D
Enter the BrideGroomName :
Vignesh M
Enter the venue of the wedding :
ShriDevi Mahal
Enter the Date :
23/09/2016
Enter the Number of days :
2
Enter the cost of wedding hall per hour :
3000
Enter the flowerCost :
4000
Enter the goldFoilCost :
5000
Enter the backDropCost :
20000
Enter the Break Fast Cost :
35000
Enter the Lunch Cost :
50000
Enter the Dinner Cost :
20000
Enter the Budget :
300000
Cost for Wedding Hall :144000
Cost for Decoration :29000
Cost for Food :210000
Total cost :383000
The Estimate exceeds the Budget!

Step by step
Solved in 4 steps with 2 images

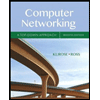
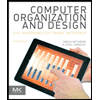
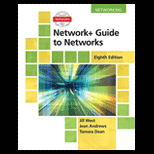
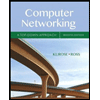
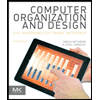
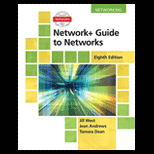
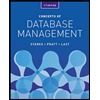
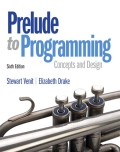
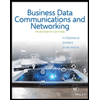