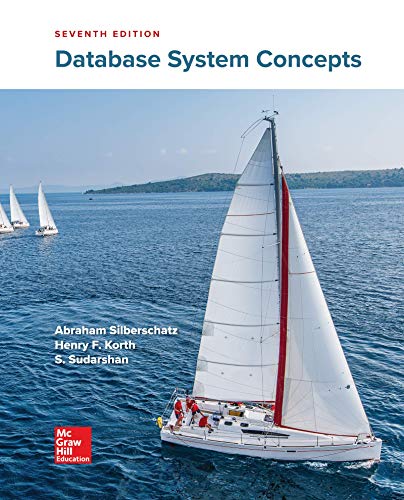
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Write a program (in main.cpp) that:
- Prompts the user for a filename containing node data.
- Outputs the minimal spanning tree for a given graph.
You will need to implement the createSpanningGraph method in minimalSpanTreeType.h to create the graph and the weight matrix.
Note: Files Ch20_Ex21Data.txt and Ch20_Ex4Data.txt contain node data that you may test your program with.
minimalSpanTreeType.h :
#ifndef H_msTree
#define H_msTree
#include <iostream>
#include <fstream>
#include <iomanip>
#include <cfloat>
#include "graphType.h"
using namespace std;
class msTreeType: public graphType
{
public:
void createSpanningGraph();
//Function to create the graph and the weight matrix.
//Postcondition: The graph using adjacency lists and
// its weight matrix is created.
void minimalSpanning(int sVertex);
//Function to create a minimal spanning tree with
//root as sVertex.
// Postcondition: A minimal spanning tree is created.
// The weight of the edges is also
// saved in the array edgeWeights.
void printTreeAndWeight();
//Function to output the edges of the minimal
//spanning tree and the weight of the minimal
//spanning tree.
//Postcondition: The edges of a minimal spanning tree
// and their weights are printed.
msTreeType(int size = 0);
//Constructor
//Postcondition: gSize = 0; maxSize = size;
// graph is an array of pointers to linked
// lists.
// weights is a two-dimensional array to
// store the weights of the edges.
// edges is an array to store the edges
// of a minimal spanning tree.
// egdeWeight is an array to store the
// weights of the edges of a minimal
// spanning tree.
~msTreeType();
//Destructor
//The storage occupied by the vertices and the arrays
//weights, edges, and edgeWeights is deallocated.
protected:
int source;
double **weights;
int *edges;
double *edgeWeights;
};
void msTreeType::createSpanningGraph()
{
// TODO: Implement createSpanningGraph
} //createWeightedGraph
void msTreeType::minimalSpanning(int sVertex)
{
int startVertex, endVertex;
double minWeight;
source = sVertex;
bool *mstv;
mstv = new bool[gSize];
for (int j = 0; j < gSize; j++)
{
mstv[j] = false;
edges[j] = source;
edgeWeights[j] = weights[source][j];
}
mstv[source] = true;
edgeWeights[source] = 0;
for (int i = 0; i < gSize - 1; i++)
{
minWeight = DBL_MAX;
for (int j = 0; j < gSize; j++)
if (mstv[j])
for (int k = 0; k < gSize; k++)
if (!mstv[k] && weights[j][k] < minWeight)
{
endVertex = k;
startVertex = j;
minWeight = weights[j][k];
}
mstv[endVertex] = true;
edges[endVertex] = startVertex;
edgeWeights[endVertex] = minWeight;
} //end for
} //end minimalSpanning
void msTreeType::printTreeAndWeight()
{
double treeWeight = 0;
cout << "Source Vertex: " << source << endl;
cout << "Edges Weight" << endl;
for (int j = 0; j < gSize; j++)
{
if (edges[j] != j)
{
treeWeight = treeWeight + edgeWeights[j];
cout << "("<<edges[j] << ", " << j << ") "
<< edgeWeights[j] << endl;
}
}
cout << endl;
cout << "Minimal Spanning Tree Weight: "
<< treeWeight << endl;
} //end printTreeAndWeight
//Constructor
msTreeType::msTreeType(int size)
:graphType(size)
{
weights = new double*[size];
for (int i = 0; i < size; i++)
weights[i] = new double[size];
edges = new int[size];
edgeWeights = new double[size];
}
//Destructor
msTreeType::~msTreeType()
{
for (int i = 0; i < gSize; i++)
delete [] weights[i];
delete [] weights;
delete [] edges;
delete edgeWeights;
}
#endif
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Given the following function and a Linked List : head->a->b->c->d->e->f->g->null, where a,...g are void fun1(Node head) { if(head == null) return; System.out.printf("%d ", head.data); fun1(head.link.link); } What does the call fun1(head) do? Binary search tree properties.arrow_forwardThe linked list provided below:2 > 3 > 1 > 7 > 5 > 18 > NULLHere the > symbol means a pointer.Write a complete Python program which deletes the third last node of thelist and returns to you the list as below2 > 3 > 1 > 5 > 18 > NULLThe third last node (with a value 7) has now been dislodged from the list.Here are the few things to consider :(a) We do NOT know the length of the list.(b) Your solution should be in O(n) time and O(1) space having madejust a single pass through the list.arrow_forwardthe number of edges in a complete 2 of 2 undirected graph of 80 vertices is.? Select one: a. exactly equals 3160 b. None c. less than or equals 3160 d. less than or equals 6320 e. exactly equals 6320 Assume that you have a doubly link list. Pointer x is pointing to the last node in the link list and pointer y is pointing to before the last node. which of the following will remove the node pointed by x from the list? Select one: O a. delete x; b. y->prev->next = y->next; X->prev= y->prev; delete x; O C. X->prev->next = x->next; y->prev= x->prev; delete x; O d. x->next = y; y->prev = x->prev; delete x;arrow_forward
- In this problem you will implement a function called triangle_countwhich will take as input a graph object G, representing an undirectedgraph G, and will return the number of triangles in G. Do not use anyimports for this problem. To simplify the problem you may assume thateach edge is stored twice in G. That is if an edge goes from u to v then vwill be in u’s collection and u will be in v’s collection. If you would prefer,you may assume that an edge is only stored once. In either case G willneed to be regarded as undirected.arrow_forwardThe implementations of the methods addAll, removeAll, retainAll, retainAll, containsAll(), and toArray(T[]) are omitted in the MyList interface. Implement these methods. Use the template at https://liveexample.pearsoncmg.com/test/Exercise24_01_13e.txt to implement these methods this the code i have for the write your own code part import java.util.Iterator; interface MyList<E> extends java.util.Collection<E> { void add(int index, E e); boolean contains(Object e); E get(int index); int indexOf(Object e); int lastIndexOf(E e); E remove(int index); E set(int index, E e); void clear(); boolean isEmpty(); Iterator<E> iterator(); boolean containsAll(java.util.Collection<?> c); boolean addAll(java.util.Collection<? extends E> c); boolean removeAll(java.util.Collection<?> c); boolean retainAll(java.util.Collection<?> c); Object[] toArray(); <T> T[] toArray(T[] a); int size();} and its…arrow_forwardCreate a nested class called DoubleNode that allows you to create doubly-linked lists with each node containing a reference to the item before it and the item after it (or null if neither of those items exist). Then implement static methods for the following operations: insert before a given node, insert after a given node, remove a given node, remove from a given node, insert at the beginning, insert at the end, remove from a given node, and remove a given node.arrow_forward
- Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the PrintNodeData() method// to print the entire linked list //…arrow_forwardGiven the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user- input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 7/16/18 5arrow_forwardPlease help with the program below. Need to write a program called dfs-stack.py in python that uses the algorithm below without an agency list but instead uses an adjacency matrix. The program should prompt the user for the number of vertices V, in the graph.Please read the directions below I will post a picture of the instructions and the algorithm.arrow_forward
- Given the MileageTrackerNode class, complete main() in the MileageTrackerLinkedList class to insert nodes into a linked list (using the insertAfter() method). The first user-input value is the number of nodes in the linked list. Use the printNodeData() method to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 public class MileageTrackerNode { private double miles; // Node data private String date; // Node data private MileageTrackerNode nextNodeRef; // Reference to the next node public MileageTrackerNode() { miles = 0.0; date = ""; nextNodeRef = null; } // Constructor public MileageTrackerNode(double milesInit, String dateInit) { this.miles =…arrow_forwardI'm confused about this question. Any help is appreciated!arrow_forwardIn your own words, please explain why "expandability" is an important factor to think about while selecting neighbor devices.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
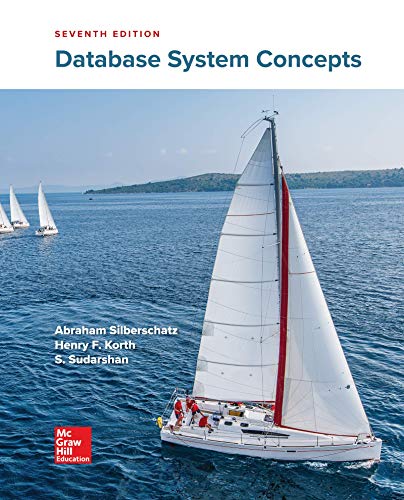
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
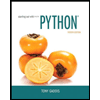
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
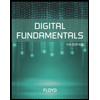
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
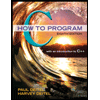
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
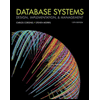
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
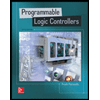
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education