Write a program that asks the user for the name of a file. The program should read all the numbers from the given file and display the total and average of all numbers in the following format (three decimal digits): Total: nnnnn.nnn
import java.io.File
import java.io.FileNotFoundException;
import java.util.Scanner;
public class FileRead {
public static void main(String[] args) throws FileNotFoundException {
Scanner keyboard = new Scanner(System.in);
System.out.println("Please enter the file name or type QUIT to exit:");
String filename = keyboard.nextLine();
if (filename.equalsIgnoreCase("Quit"))
return;
File myFile = new File(filename);
while (!myFile.exists()) {
System.out.println("File: " + filename + " does not exist.");
System.out.println("Please enter the file name again or type QUIT to exit:");
filename = keyboard.nextLine();
if (filename.equalsIgnoreCase("Quit")) {
return;
}
myFile = new File(filename);
}
Scanner inputFile = new Scanner(myFile);
int lines = 0;
while (inputFile.hasNext()) {
String str = inputFile.nextLine();
lines++;
System.out.println(lines + ": " + str);
}
inputFile.close();
}
}
Test Case 1
input1.txtENTER
Total: 11.800\n
Average: 2.950\n
Test Case 2
input2.txtENTER
Total: 17.300\n
Average: 3.460\n
Test Case 3
input3.txtENTER
Total: 1.124\n
Average: 1.124\n
Test Case 4
input4.txtENTER
File input4.txt is empty.\n
Test Case 5
input5.txtENTER
File: input5.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
input1.txtENTER
Total: 11.800\n
Average: 2.950\n
Test Case 6
qUiTENTER
Test Case 7
input5.txtENTER
File: input5.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
QuiTENTER

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

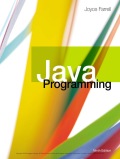
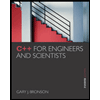
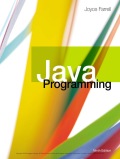
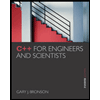