Write a program that creates a table for the user's choice of basic math operations (+, -, *, /, and %). These operations will all be performed in an integer-only way. (Modulo doesn't work with decimals anyway... And you're going to have enough trouble lining up the table without dealing with decimal places on division!) The user should also be allowed to choose the size of the table (it will, of course, be square: 4x4, 5x5, etc.). You may limit the maximum size of the table, for formatting purposes. Make sure your table is neat and lines up nicely (see the examples below). Columns should all be of equal width. This should be the minimum width necessary to hold the largestanswer for the table's operation. (Be careful! This may be a negative value!) Note that, if each column is as small as possible, you can fit more of them on the screen... Try to use functions to break up the program into more manageable -- re-usable -- pieces. (You'll note that most of the table display is identical but for the corner symbol, the largest answer width, and the calculation of the entries themselves... Perhaps you could parameterize the data portions of this and ...er... 'farm-out' the calculation of the entries to a parameterized little helper function..?) As an example, the program interaction might look something like this: $ ./mathtable.out Welcome to the Math Table Program!!! Table Menu 1) Addition table 2) Multiplication table 3) Subtraction table 4) Division table 5) Remainder table 6) Quit Choice: a What size should the addition table be? 50 I'm sorry, 50 is too large of an addition table to print on the screen... What size should the addition table be? 5 Thank you...calculating... + | 1 2 3 4 5 ----+----------------- 1 | 2 3 4 5 6 2 | 3 4 5 6 7 3 | 4 5 6 7 8 4 | 5 6 7 8 9 5 | 6 7 8 9 10 Table Menu 1) Addition table 2) Multiplication table 3) Subtraction table 4) Division table 5) Remainder table 6) Quit Choice: R What size should the remainder table be? 7 Thank you...calculating... % | 1 2 3 4 5 6 7 ----+---------------- 1 | 0 1 1 1 1 1 1 2 | 0 0 2 2 2 2 2 3 | 0 1 0 3 3 3 3 4 | 0 0 1 0 4 4 4 5 | 0 1 2 1 0 5 5 6 | 0 0 0 2 1 0 6 7 | 0 1 1 3 2 1 0 Table Menu 1) Addition table 2) Multiplication table 3) Subtraction table 4) Division table 5) Remainder table 6) Quit Choice: 3 What size should the subtraction table be? 30 I'm sorry, 30 is too large of a subtraction table to print on the screen... What size should the subtraction table be? -3 I'm sorry, -3 would be stupid... What size should the subtraction table be? 3 Thank you...calculating... - | 1 2 3 ----+----------- 1 | 0 -1 -2 2 | 1 0 -1 3 | 2 1 0 Table Menu 1) Addition table 2) Multiplication table 3) Subtraction table 4) Division table 5) Remainder table 6) Quit Choice: q Thank you for using the MTP!! Endeavor to have a auspicious day!
Write a program that creates a table for the user's choice of basic math operations (+, -, *, /, and %). These operations will all be performed in an integer-only way. (Modulo doesn't work with decimals anyway... And you're going to have enough trouble lining up the table without dealing with decimal places on division!)
The user should also be allowed to choose the size of the table (it will, of course, be square: 4x4, 5x5, etc.). You may limit the maximum size of the table, for formatting purposes.
Make sure your table is neat and lines up nicely (see the examples below). Columns should all be of equal width. This should be the minimum width necessary to hold the largestanswer for the table's operation. (Be careful! This may be a negative value!) Note that, if each column is as small as possible, you can fit more of them on the screen...
Try to use functions to break up the program into more manageable -- re-usable -- pieces. (You'll note that most of the table display is identical but for the corner symbol, the largest answer width, and the calculation of the entries themselves... Perhaps you could parameterize the data portions of this and ...er... 'farm-out' the calculation of the entries to a parameterized little helper function..?)
As an example, the program interaction might look something like this:
$ ./mathtable.out
Welcome to the Math Table Program!!!
Table Menu
1) Addition table
2) Multiplication table
3) Subtraction table
4) Division table
5) Remainder table
6) Quit
Choice: a
What size should the addition table be? 50
I'm sorry, 50 is too large of an addition table to print on the screen...
What size should the addition table be? 5
Thank you...calculating...
+ | 1 2 3 4 5
----+-----------------
1 | 2 3 4 5 6
2 | 3 4 5 6 7
3 | 4 5 6 7 8
4 | 5 6 7 8 9
5 | 6 7 8 9 10
Table Menu
1) Addition table
2) Multiplication table
3) Subtraction table
4) Division table
5) Remainder table
6) Quit
Choice: R
What size should the remainder table be? 7
Thank you...calculating...
% | 1 2 3 4 5 6 7
----+----------------
1 | 0 1 1 1 1 1 1
2 | 0 0 2 2 2 2 2
3 | 0 1 0 3 3 3 3
4 | 0 0 1 0 4 4 4
5 | 0 1 2 1 0 5 5
6 | 0 0 0 2 1 0 6
7 | 0 1 1 3 2 1 0
Table Menu
1) Addition table
2) Multiplication table
3) Subtraction table
4) Division table
5) Remainder table
6) Quit
Choice: 3
What size should the subtraction table be? 30
I'm sorry, 30 is too large of a subtraction table to print on the screen...
What size should the subtraction table be? -3
I'm sorry, -3 would be stupid...
What size should the subtraction table be? 3
Thank you...calculating...
- | 1 2 3
----+-----------
1 | 0 -1 -2
2 | 1 0 -1
3 | 2 1 0
Table Menu
1) Addition table
2) Multiplication table
3) Subtraction table
4) Division table
5) Remainder table
6) Quit
Choice: q
Thank you for using the MTP!!
Endeavor to have a auspicious day!
$

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

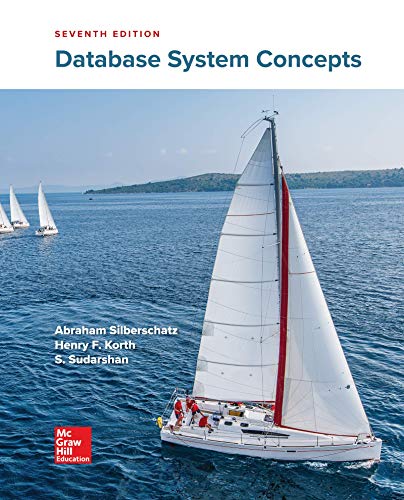
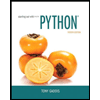
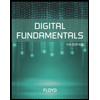
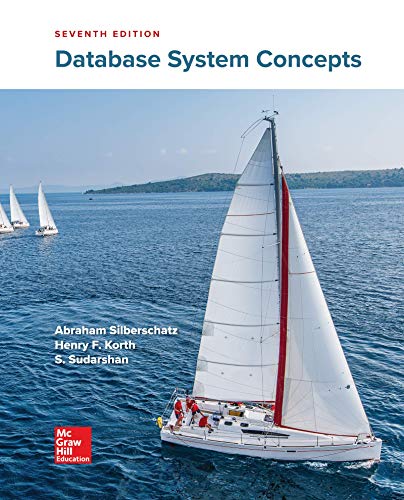
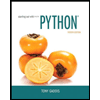
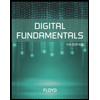
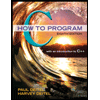
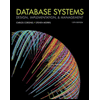
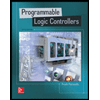