Write a program that simulates the game “hangman”.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.4: A Case Study: Rectangular To Polar Coordinate Conversion
Problem 9E: (Numerical) Write a program that tests the effectiveness of the rand() library function. Start by...
Related questions
Question
Program definition:
Write a program that simulates the game “hangman”.
a. In the game of hangman, have a user enter a word for “another” player to guess.
b. After the word to guess is entered, clear the screen and present a list of blanks or underscores representing unguessed letters of the hidden word.
c. Have the user guess a letter in the word. If the letter is in the word, display the letter instead of the blank or underscore and display the remaining blanks or underscores for the unguessed letters, all in the proper position.
d. If a previously guessed letter is repeated, whether in the word or not, another try is allowed without penalty. If a guess is incorrect, the game state progresses toward being “hung”.
e. There are 8 bad guesses allowed before the game is lost.
f. The game will progress through the states of PLATORM, HEAD, BODY, LEFT_ARM, RIGHT_ARM, LEFT_LEG, RIGHT_LEG, and HUNG. Use an ENUM to represent the game states listed.
g. Use a linked list to represent the list of letters for the word to guess
h. Use a class to represent each letter in the word. The class should have the following private data members:
letter of the word
boolean to represent if a blank (or underscore) or the letter is to be displayed
pointer to the next class in the linked list
i. Code the appropriate constructors, setters, and getters for accessing and manipulating the class.
j. Use a second linked list to represent the letters that have already been guessed. This second linked list may be a structure.
k. Keep asking the user for another guess until the entire word has been guessed or the game state is “HUNG”.
l. After each guess, display the current state of the game, the letters of the word guessed so far as well as blanks or underscores and the list of letters already guessed.
m. The user should be able to play the game until the word “quit” is entered instead of entering another word to guess.
n. Enclose the necessary code in a try() / catch{} to trap guessed letters other than ‘A’-‘Z’ or ‘a’ – ‘z’. If a letter or symbol outside this range is entered, ask the user to enter a correct response.
You do not have to code for phrases, only words.
You do not have to ensure the proper capitalization, i.e., you may require all your letters be lower or upper case.
o. You do not have to use functions beyond what is required for the class, however, the use of functions is always encouraged.
p. Each class should have its own .cpp and header file. Any functions written for the main program should be in a separate header file.
The code to insert, print and search is in the book. You may use and modify the code however you wish to provide a solution to the assignment. You should not use the template code, i.e., overloaded ++, etc. I want you to write the code to manipulate and traverse the linked list. Please give credit to the authors in your comments for any code you use directly.
The code file must contain the following as documentation.
The name of your C++ file
Your name
Some kind of date, either the due date or the date you finished
The type of input
The type of output
A brief description of the algorithm or purpose of the program
For example:
/ * Program name: assignment1.cpp
Author: Pam Smith Date: 8/25/14
Input: requests an input and output file name from the user (inputfile.txt provided).
Output is name of your choice
Output: displays output on the console and writes output to file name provided
Description: This program translates a word or phrase using the ROT13 cipher*/
All procedures and functions should be documented with the following information:
A brief description of the purpose of the function or procedure
Signature : return type, name, parameters (including type)
A list of the parameters and what each represents
A description of the function return type as applicable
Precondition(s) as applicable
Postcondition(s) as applicable
For example:
/*
myfunction outputs the values contained in the input parameters.
myfunction returns no value; it takes an integer parameter as input and a character parameter as input
The integer parameter contains the ordinal number representing a letter in the alphabet
The character parameter contains a letter of the alphabet
No value is returned
Precondition: the integer value must contain a valid number representing the ordinal number of the letter contained in the character parameter; the character parameter must contain a value representing a letter of the alphabet
Postcondition: there are no post conditions
*/
void myfunction (int a, char b) {}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
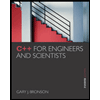
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
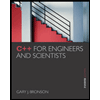
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr