write a program that simulates the “Rock-paper-Scissors” game. In this game the user and the computer each pick “rock”, “paper” or “scissors”. The winner of each round is decided by the following rule: Scissors wins over Paper (it can cut the paper) but loses to Rock (itcan get crushed by the rock)Paper wins over Rock (it can wrap the rock) but loses to Scissors (it can be cut by scissors)Rock wins over Scissors( it can crush the scissors) but loses to Paper (it can be wrapped by paper)You repeat the rounds until userpicks “(q)uit” to quit the game. Specifically, the program 1.Welcomes the user and prints the rules of the game. 2.While the user pick is not “q”, repeats steps a-e: a.Generates computer’s pick using random function. b.Decides the winner based on the above rules. c.Prints the user and computer pick and the winner for this round. d.Keeps track of i.number of rounds ii.number of ties, iii.number of user wins iv.number of computer wins. e.Asks the user for the next pick 3.Prints all the counts and exits. To help you plan your code, a high-level program outline is included below. You can base your code on this pseudocode. At the end, two sample runs are shown. Once you implement these functions, test them individually from the shell. E.g. getResult(‘r’, ‘s’) returns ‘user’ as the winner, and getResult(‘s’,’p’) returns ‘computer’ as the winner. Save your program to a file with a name of the format first_last_RPS.py. Submit this file. High-level Program Outline: 1.printWelcome(): Function to print the welcome message and the rules of the game. Accepts no parameters, doesn’t return anything. Print the welcome message. Print the rules of the game. 2.getUserPick(): Function to prompt the user for the pick (including quit). Accepts no parameters, returns user’s pick. Prompt the user to pick a choice and get input from the user. Return the user input. 3.getResult(user, computer): Function that compares the user and computer choice and returns who the winner is (“tie”, “user” or “computer”). 4.pickRPS(): Function that simulates the computer picking its choice. Accepts no parameters, and returns computer’s pick as “rock”, “paper” or “scissor”. Usefunctions from the random module (random.randint or random.choice) BE SURE TO IMPORT THE RANDOM MODULE AT THE TOP OF THE FILE. Use the built-in help to get more information on these functions: help(random.randint) or help(random.choice). 5.main(): The main function that ties all these functions together as shown below: Call printWelcome Initialize all counts to zero (countUserWins, countComputerWins, countTies, countTotal) Call get UserPickto get user’s pick, save the return value to a variable user. While user’s pick is not equal to “q” :Call pickRPS() to get computer pick, save the return value to a variable called computer Call getResultpassing user’s pickand computer’s pick, save the result to a variable called winner. Print and announce the winner. Increment appropriate count based on the result Call getUserPick again Print final countsPrint “Bye” Useful Functions: 1.“randint” and “choice” from the random module .2.“startswith” function from the str class.
write a program that simulates the “Rock-paper-Scissors” game. In this game the user and the computer each pick “rock”, “paper” or “scissors”. The winner of each round is decided by the following rule: Scissors wins over Paper (it can cut the paper) but loses to Rock (itcan get crushed by the rock)Paper wins over Rock (it can wrap the rock) but loses to Scissors (it can be cut by scissors)Rock wins over Scissors( it can crush the scissors) but loses to Paper (it can be wrapped by paper)You repeat the rounds until userpicks “(q)uit” to quit the game. Specifically, the program 1.Welcomes the user and prints the rules of the game. 2.While the user pick is not “q”, repeats steps a-e: a.Generates computer’s pick using random function. b.Decides the winner based on the above rules. c.Prints the user and computer pick and the winner for this round. d.Keeps track of i.number of rounds ii.number of ties, iii.number of user wins iv.number of computer wins. e.Asks the user for the next pick 3.Prints all the counts and exits. To help you plan your code, a high-level program outline is included below. You can base your code on this pseudocode. At the end, two sample runs are shown. Once you implement these functions, test them individually from the shell. E.g. getResult(‘r’, ‘s’) returns ‘user’ as the winner, and getResult(‘s’,’p’) returns ‘computer’ as the winner. Save your program to a file with a name of the format first_last_RPS.py. Submit this file. High-level Program Outline: 1.printWelcome(): Function to print the welcome message and the rules of the game. Accepts no parameters, doesn’t return anything. Print the welcome message. Print the rules of the game. 2.getUserPick(): Function to prompt the user for the pick (including quit). Accepts no parameters, returns user’s pick. Prompt the user to pick a choice and get input from the user. Return the user input. 3.getResult(user, computer): Function that compares the user and computer choice and returns who the winner is (“tie”, “user” or “computer”). 4.pickRPS(): Function that simulates the computer picking its choice. Accepts no parameters, and returns computer’s pick as “rock”, “paper” or “scissor”. Usefunctions from the random module (random.randint or random.choice) BE SURE TO IMPORT THE RANDOM MODULE AT THE TOP OF THE FILE. Use the built-in help to get more information on these functions: help(random.randint) or help(random.choice). 5.main(): The main function that ties all these functions together as shown below: Call printWelcome Initialize all counts to zero (countUserWins, countComputerWins, countTies, countTotal) Call get UserPickto get user’s pick, save the return value to a variable user. While user’s pick is not equal to “q” :Call pickRPS() to get computer pick, save the return value to a variable called computer Call getResultpassing user’s pickand computer’s pick, save the result to a variable called winner. Print and announce the winner. Increment appropriate count based on the result Call getUserPick again Print final countsPrint “Bye” Useful Functions: 1.“randint” and “choice” from the random module .2.“startswith” function from the str class.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter5: Control Structures Ii (repetition)
Section: Chapter Questions
Problem 27PE
Related questions
Question
provide a simple python script following the guidelines below with an explanation of how it functions, please.
I've attached to pictures of the desired outcome.
write a program that simulates the “Rock-paper-Scissors” game. In this game the user and the computer each pick “rock”, “paper” or “scissors”. The winner of each round is decided by the following rule: Scissors wins over Paper (it can cut the paper) but loses to Rock (itcan get crushed by the rock)Paper wins over Rock (it can wrap the rock) but loses to Scissors (it can be cut by scissors)Rock wins over Scissors( it can crush the scissors) but loses to Paper (it can be wrapped by paper)You repeat the rounds until userpicks “(q)uit” to quit the game. Specifically, the program
1.Welcomes the user and prints the rules of the game.
2.While the user pick is not “q”, repeats steps a-e:
a.Generates computer’s pick using random function.
b.Decides the winner based on the above rules.
c.Prints the user and computer pick and the winner for this round.
d.Keeps track of
i.number of rounds
ii.number of ties,
iii.number of user wins
iv.number of computer wins.
e.Asks the user for the next pick
3.Prints all the counts and exits.
To help you plan your code, a high-level program outline is included below. You can base your code on this pseudocode. At the end, two sample runs are shown. Once you implement these functions, test them individually from the shell. E.g. getResult(‘r’, ‘s’) returns ‘user’ as the winner, and getResult(‘s’,’p’) returns ‘computer’ as the winner. Save your program to a file with a name of the format first_last_RPS.py. Submit this file.
High-level Program Outline:
1.printWelcome(): Function to print the welcome message and the rules of the game. Accepts no parameters, doesn’t return anything.
Print the welcome message.
Print the rules of the game.
2.getUserPick(): Function to prompt the user for the pick (including quit). Accepts no parameters, returns user’s pick.
Prompt the user to pick a choice and get input from the user.
Return the user input.
3.getResult(user, computer): Function that compares the user and computer choice and returns who the winner is (“tie”, “user” or “computer”).
4.pickRPS(): Function that simulates the computer picking its choice. Accepts no parameters, and returns computer’s pick as “rock”, “paper” or “scissor”. Usefunctions from the random module (random.randint or random.choice) BE SURE TO IMPORT THE RANDOM MODULE AT THE TOP OF THE FILE. Use the built-in help to get more information on these functions: help(random.randint) or help(random.choice).
5.main(): The main function that ties all these functions together as shown below:
Call printWelcome
Initialize all counts to zero (countUserWins, countComputerWins, countTies, countTotal)
Call get UserPickto get user’s pick, save the return value to a variable user. While user’s pick is not equal to “q”
:Call pickRPS() to get computer pick, save the return value to a variable called computer
Call getResultpassing user’s pickand computer’s pick, save the result to a variable called winner.
Print and announce the winner.
Increment appropriate count based on the result
Call getUserPick again
Print final countsPrint “Bye”
Useful Functions:
1.“randint” and “choice” from the random module
.2.“startswith” function from the str class.
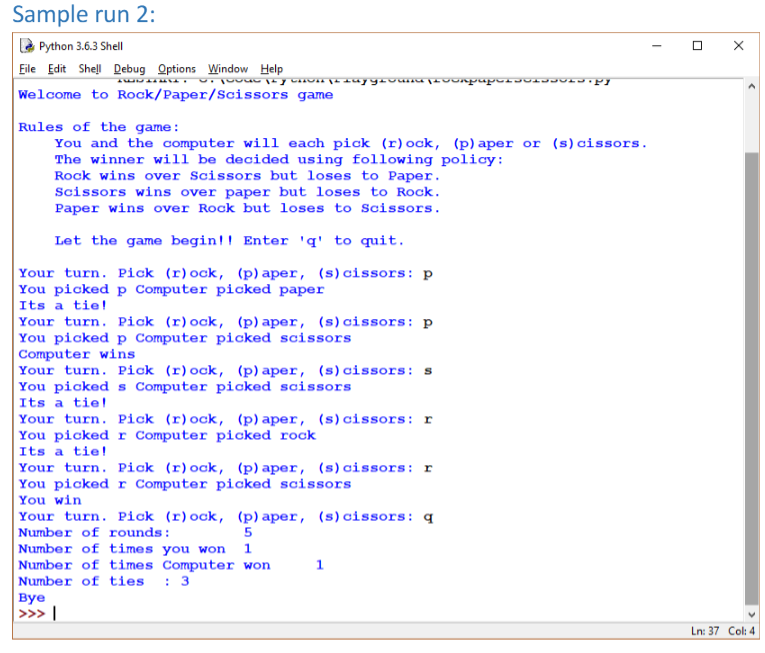
Transcribed Image Text:Sample run 2:
E Python 3.6.3 Shell
Eile Edit Shell Debug Options Window Help
Welcome to Rock/Paper/Scissors game
Rules of the game:
You and the computer will each pick (r) ock, (p)aper or (s)cissors.
The winner will be decided using following policy:
Rock wins over Scissors but loses to Paper.
Scissors wins over paper but loses to Rock.
Paper wins over Rock but loses to Scissors.
Let the game begin!! Enter 'q' to quit.
Your turn. Pick (r)ock, (p) aper, (s)cissors: p
You picked p Computer picked paper
Its a tie!
Your turn. Pick (r)ock, (p) aper, (s)cissors: p
You picked p Computer picked scissors
Computer wins
Your turn. Pick (r)ock, (p)aper, (s) cissors: s
You picked s Computer picked scissors
Its a tiel
Your turn. Pick (r)ock, (p) aper, (s)cissors: r
You picked r Computer picked rock
Its a tie !
Your turn. Pick (r)ock, (p) aper, (s)cissors: r
You picked r Computer picked scissors
You win
Your turn. Pick (r)ock, (p) aper, (s)cissors: q
Number of rounds:
Number of times you won 1
Number of times Computer won
Number of ties : 3
вуе
| >>» |
Ln: 37 Col: 4
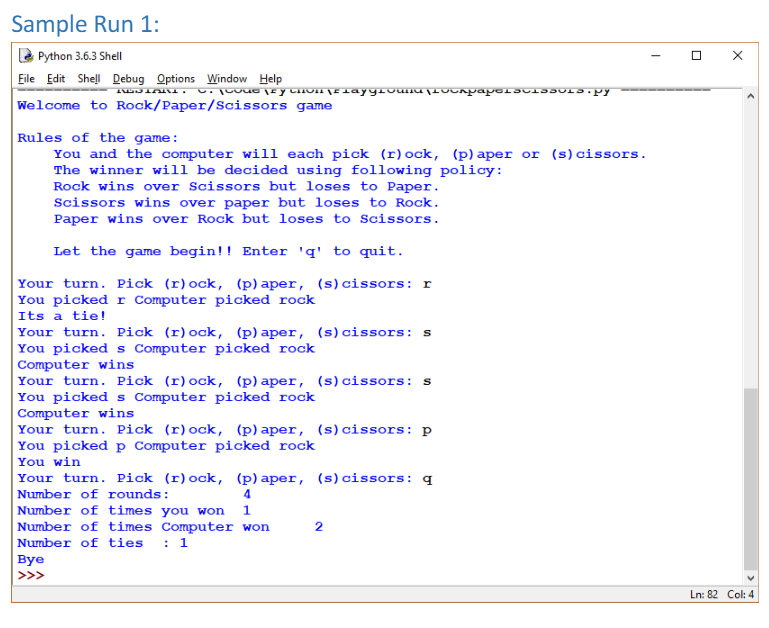
Transcribed Image Text:Sample Run 1:
E Python 3.6.3 Shell
Eile Edit Shel Debug Options Window Help
Welcome to Rock/Paper/Scissors game
Rules of the game:
You and the computer will each pick (r) ock, (p)aper or (s)cissors.
The winner will be decided using following policy:
Rock wins over Scissors but loses to Paper.
Scissors wins over paper but loses to Rock.
Paper wins over Rock but loses to Scissors.
Let the game begin!! Enter 'q' to quit.
Your turn. Pick (r)ock, (p) aper, (s)cissors: r
You picked r Computer picked rock
Its a tie!
Your turn. Pick (r)ock, (p) aper, (s)cissors: s
You picked s Computer picked rock
Computer wins
Your turn. Pick (r)ock, (p) aper, (s)cissors: s
You picked s Computer picked rock
Computer wins
Your turn. Pick (r)ock, (p) aper, (s)cissors: p
You picked p Computer picked rock
You win
Your turn. Pick (r)ock, (p) aper, (s) cissors: q
Number of rounds:
Number of times you won 1
Number of times Computer won
: 1
2
Number of ties
Вye
>>>
Ln: 82 Col: 4
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
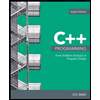
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
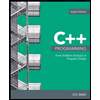
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning