Write a program that takes numbers from the user and places them into an array. After each new entry from the user, the program prints out the current array of numbers. Several rules must be followed: Each new number is checked and added to the array ONLY if it is larger than the number in the previous position in the array. This condition is not checked for the first number. In other words, the new number is rejected and nothing is added to the array if the entry is too-small. The new number is added to the array EXCEPT: when the user enters a number greater than 75, the number 0 is added to the array instead. when the user enters a multiple of 7 (and less than or equal to 75), the number 7 is added to the array instead. The program exits after 10 numbers have been placed into the array. Programming requirements: You must define and call at least one function. I recommend printArray(...) You must use at least one array. Assume the entries are integers Example Test Case: Enter a number: 4 Current numbers: 4 Enter a number: 25 Current numbers: 4 25 Enter a number: 108 Current numbers: 4 25 0 Enter a number: 87 Current numbers: 4 25 0 0 Enter a number: 14 Current numbers: 4 25 0 0 7 Enter a number: 5 Too small! Current numbers: 4 25 0 0 7 Enter a number: 3 Too small! Current numbers: 4 25 0 0 7 Enter a number: 20 Current numbers: 4 25 0 0 7 20 Enter a number: 18 Too small! Current numbers: 4 25 0 0 7 20 Enter a number: 21 Current numbers: 4 25 0 0 7 20 7 Enter a number: 27 Current numbers: 4 25 0 0 7 20 7 27 Enter a number: 35 Current numbers: 4 25 0 0 7 20 7 27 7 Enter a number: 10 Current numbers: 4 25 0 0 7 20 7 27 7 10 All done!
Write a program that takes numbers from the user and places them into an array. After each new entry from the user, the program prints out the current array of numbers.
Several rules must be followed:
- Each new number is checked and added to the array ONLY if it is larger than the number in the previous position in the array. This condition is not checked for the first number.
In other words, the new number is rejected and nothing is added to the array if the entry is too-small. - The new number is added to the array EXCEPT:
- when the user enters a number greater than 75, the number 0 is added to the array instead.
- when the user enters a multiple of 7 (and less than or equal to 75), the number 7 is added to the array instead.
The program exits after 10 numbers have been placed into the array.
- You must define and call at least one function. I recommend printArray(...)
- You must use at least one array. Assume the entries are integers
Example Test Case:
Enter a number: 4
Current numbers: 4
Enter a number: 25
Current numbers: 4 25
Enter a number: 108
Current numbers: 4 25 0
Enter a number: 87
Current numbers: 4 25 0 0
Enter a number: 14
Current numbers: 4 25 0 0 7
Enter a number: 5
Too small!
Current numbers: 4 25 0 0 7
Enter a number: 3
Too small!
Current numbers: 4 25 0 0 7
Enter a number: 20
Current numbers: 4 25 0 0 7 20
Enter a number: 18
Too small!
Current numbers: 4 25 0 0 7 20
Enter a number: 21
Current numbers: 4 25 0 0 7 20 7
Enter a number: 27
Current numbers: 4 25 0 0 7 20 7 27
Enter a number: 35
Current numbers: 4 25 0 0 7 20 7 27 7
Enter a number: 10
Current numbers: 4 25 0 0 7 20 7 27 7 10
All done!

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

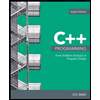
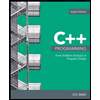