Write a program that will read a string and display the string in reverse order. Use the code snippet given below to solve the problem. Hint. Push each character of a string onto the stack and then pop and display each character from the stack. import os class Stack: def __init__(self, size): self.stack = [0] * size self.top = 0 self.size = size def push(self,item): if (self.top < self.size): self.stack[self.top] = item self.top += 1 else: print("Stack Overflow") def pop(self): if (self.top > 0): self.top -= 1 return self.stack[self.top] else: print("Stack Underflow") def is_empty(self): return (self.top == 0) def is_full(self): return (self.top == self.size) def makenull(self): self.top = 0 def peek(self): if (self.top > 0): return self.stack[self.top - 1] else: print("Stack Underflow") string = input("Enter a string: ") # write code here to display the string in reverse order Sample Run: Enter a string: Hello olleH
Write a program that will read a string and display the string in reverse order. Use the code
snippet given below to solve the problem.
Hint. Push each character of a string onto the stack and then pop and display each character from the stack.
import os
class Stack:
def __init__(self, size):
self.stack = [0] * size
self.top = 0
self.size = size
def push(self,item):
if (self.top < self.size):
self.stack[self.top] = item
self.top += 1
else:
print("Stack Overflow")
def pop(self):
if (self.top > 0):
self.top -= 1
return self.stack[self.top]
else:
print("Stack Underflow")
def is_empty(self):
return (self.top == 0)
def is_full(self):
return (self.top == self.size)
def makenull(self):
self.top = 0
def peek(self):
if (self.top > 0):
return self.stack[self.top - 1]
else:
print("Stack Underflow")
string = input("Enter a string: ")
# write code here to display the string in reverse order
Sample Run:
Enter a string: Hello
olleH
![CpE 204L Hands-on Lab Activity 2
Write a program that will read a string and display the string in reverse order. Use the code
snippet given below to solve the problem.
Hint. Push each character of a string onto the stack and then pop and display each character
from the stack.
import os
class Stack:
def _init_(self, size):
self.stack = [0] * size
self.top = 0
self.size = size
def push(self,item):
if (self.top < self.size):
self.stack[self.top] = item
self.top += 1
else:
print("Stack Overflow")
def pop(self):
if (self.top > 0):
self.top -= 1
return self.stack[self.top]
else:
print("Stack Underflow")
def is_empty(self):
return (self.top == 0)
def is_full(self):
return (self.top == self.size)
def makenull(self):
self.top = 0](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F05573c82-79e3-48f4-87a0-eb905b8f01d0%2Fa1bb8a0d-f610-4747-ae12-6a77667b86fd%2Fkzyvs7_processed.png&w=3840&q=75)
![def peek(self):
if (self.top > 0):
return self.stack[self.top - 1]
else:
print("Stack Underflow")
string = input("Enter a string: ")
# write code here to display the string in reverse order
Sample Run:
Enter a string: Hello
olleH](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F05573c82-79e3-48f4-87a0-eb905b8f01d0%2Fa1bb8a0d-f610-4747-ae12-6a77667b86fd%2Fojicf24_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps with 1 images

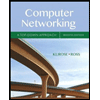
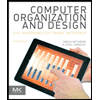
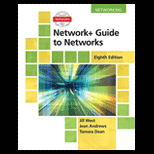
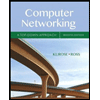
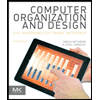
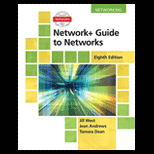
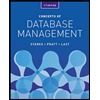
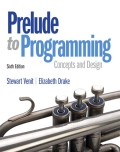
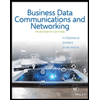