Create a Java program that uses a stack to return a list containing all permutations of a given string, and then checks to see which ones are English words. It should read words from the input and create and check the permutations for each word. For example, there are six permutations of the letters in the string "tra": tra, rta, rat, tar, atr, art implementation should include a GetPermutations class (this is the client class, driver class, demo class or console class) and a PermutationsCalculator. the program should determine which of the permutations is an English word by looking them up in a dictionary text file (just a fe words).
Create a Java program that uses a stack to return a list containing all permutations of a given string, and then checks to see which ones are English words. It should read words from the input and create and check the permutations for each word. For example, there are six permutations of the letters in the string "tra": tra, rta, rat, tar, atr, art implementation should include a GetPermutations class (this is the client class, driver class, demo class or console class) and a PermutationsCalculator. the program should determine which of the permutations is an English word by looking them up in a dictionary text file (just a fe words).
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
- My attempts at this kept giving me exception errors, I would like to compare and see what it should actually look like. I really need help with is, please
- Create a Java
program that uses a stack to return a list containing all permutations of a given string, and then checks to see which ones are English words. It should read words from the input and create and check the permutations for each word. For example, there are six permutations of the letters in the string "tra": tra, rta, rat, tar, atr, art - implementation should include a GetPermutations class (this is the client class, driver class, demo class or console class) and a PermutationsCalculator.
- the program should determine which of the permutations is an English word by looking them up in a dictionary text file (just a fe words).
-
PermutationsCalculator class Fields:
- a stack for calculating the permutations;
- an ArrayList to hold all permutations
- an ArrayList to hold unique permutations.
- an ArrayList to hold the unique English words from the permuatations
-
PermutationsCalculator class methods:
- ArrayList allPermutations(String word) will find all the permutations of the parm, store them in the all permutations ArrayList and return that ArrayList.
- ArrayList uniquePermutations(String word) will find all the unique permutations, store them in the unique permutations ArrayList and return that ArrayList.
- ArrayList uniqueWords(String word) will find the unique English words in the all permutations ArrayList, store them in the unique words ArrayList, and return that ArrayList.
- int numDuplicates() will return the number of duplicate permutations found.
-
GetPermutations class:
- Create an output file named "perms.txt".
- Create an output file named "analytics.txt".
- Prompt for the name of a dictionary text file (just a few words), then call a Dictionary constructor to read the words and create the dictionary.
- Use a loop to read words from the keyboard until the user wants to stop.
- For each word entered by the user:
- Create a PermutationsCalculator and find all permutations, the unique permutations, and the unique English words.
- Print the original word, all permutations of the word, all unique permutations of the word and all the unique English words to the output file.
- Calculate and print to the analytics file the following for each word: the word itself, the total number of permutations, the number of duplicate permutations, the percentage of permutations which were duplicates, the number of English words and the percentage of permutations which were English words.
- Calculate the following overall analytics and print them to the analytics file: the total number of words input by the user, the average length of the words input by the user, the total number of permutations generated, the total number of English words found, and the percentage of permutations which were English words.
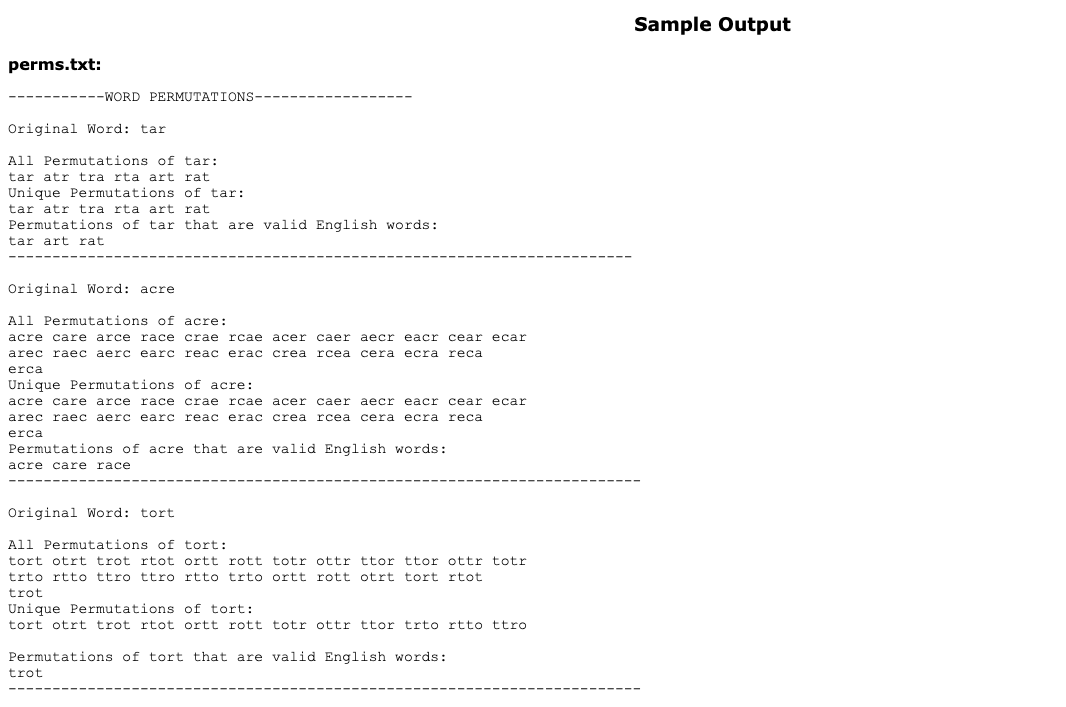
Transcribed Image Text:Sample Output
perms.txt:
---WORD PERMUTATIONS
Original Word: tar
All Permutations of tar:
tar atr tra rta art rat
Unique Permutations of tar:
tar atr tra rta art rat
Permutations of tar that are valid English words:
tar art rat
Original Word: acre
All Permutations of acre:
acre care arce
race crae
rcae acer caer aecr eacr cear ecar
arec raec aerc earc reac erac crea
rcea
сera ecra
reca
erca
Unique Permutations of acre:
acre care arce
race crae rcae
acer caer aecr eacr cear ecar
arec raаес аеr с еarc reac eraа с crea
rcea cera ecra reca
erca
Permutations of acre that are valid English words:
acre care race
Original Word: tort
All Permutations of tort:
tort otrt trot rtot ortt rott totr ottr ttor ttor ottr totr
trto rtto ttro ttro rtto trto ortt rott otrt tort rtot
trot
Unique Permutations of tort:
tort otrt trot rtot ortt rott totr ottr ttor trto rtto ttro
Permutations of tort that are valid English words:
trot
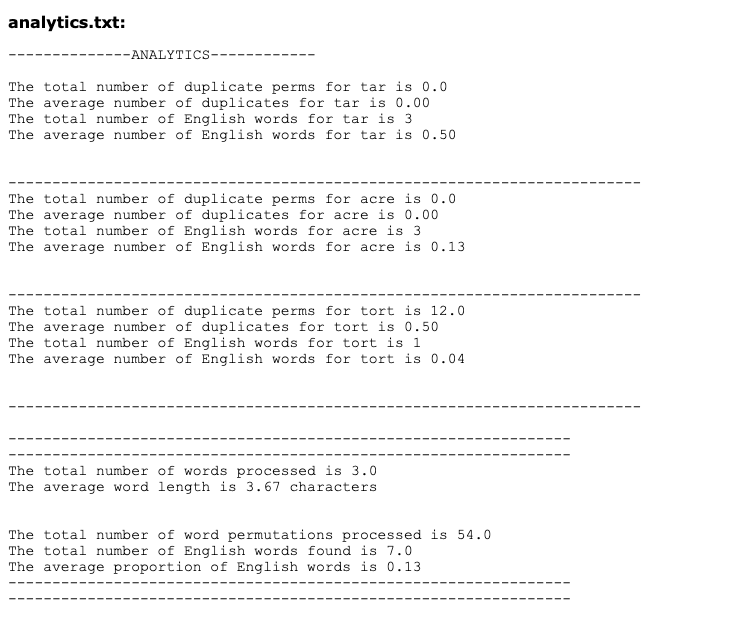
Transcribed Image Text:analytics.txt:
--ANALYTICS--
The total number of duplicate perms for tar is 0.0
The average number of duplicates for tar is 0.00
The total number of English words for tar is 3
The average number of English words for tar is 0.50
The total number of duplicate perms for acre is 0.0
The average number of duplicates for acre is 0.00
The total number of English words for acre is 3
The average number of English words for acre is 0.13
The total number of duplicate perms for tort is 12.0
The average number of duplicates for tort is 0.50
The total number of English words for tort is 1
The average number of English words for tort is 0.04
The total number of words processed is 3.0
The average word length is 3.67 characters
The total number of word permutations processed is 54.0
The total number of English words found is 7.0
The average proportion of English words is 0.13
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
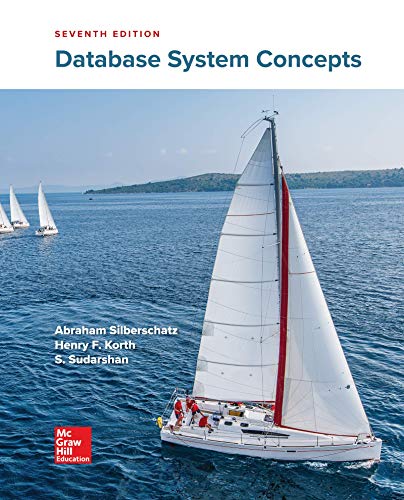
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
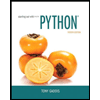
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
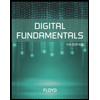
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
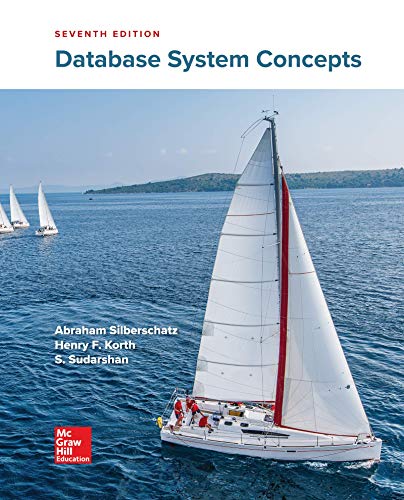
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
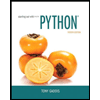
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
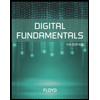
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
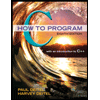
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
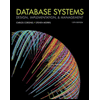
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
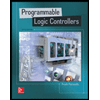
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education