write a program to encrypt outgoing messages and decrypt incoming messages using a Vigenere Cypher.
write a program to encrypt outgoing messages and decrypt incoming messages using a Vigenere Cypher. Parse a string of words from a file into tokens using the strtok_s command with pointers. In your “client” code, you will need to declare a character array that will hold 1000 characters. Using the character array, your client should call the function strtok_s() to tokenize the character array into separate words.
There should be two classes: Vigenere and Message.
Vigenere Class: Data Member: string key
Functions: Vigenere() ßconstructor
void setKey(string k)
string getKey()
string toUpperCase(string k)
string encrypt(string word)
string decrypt(string word)
The class should have a one-argument constructor. The encryption key must be in all capital letters.
Use the following code for the encrypt and decrypt functions in your Vigenere class.
string Vigenere::encrypt(string word){
string output;
for (int i = 0, j = 0; i < word.length(); ++i) {
char c = word[i];
if (c >= 'a' && c <= 'z')
c += 'A' - 'a';
else if (c < 'A' || c > 'Z')
continue;
output += (c + key[j] - 2 * 'A') % 26 + 'A';
j = (j + 1) % key.length();}
return output;}
string Vigenere::decrypt(string word){
string output;
for (int i = 0, j = 0; i < word.length(); ++i) {
char c = word[i];
if (c >= 'a' && c <= 'z')
c += 'A' - 'a';
else if (c < 'A' || c > 'Z')
continue;
output += (c - key[j] + 26) % 26 + 'A';
j = (j + 1) % key.length(); }
return output;}
Message Class: Data members:
Vigenere v
Functions: Message(string k)ß
void encryptWord(char* token)
void decryptWord(char* token)
void makeFile(string fileName)
void showWords()
The Message class should contain a vector of strings. The class should contain functions to encrypt a word, decrypt a word, create a file containing the vector of encrypted/decrypted words, and print.
Main()
In main(), call a function to create a menu that displays options to Encrypt a file, Decrypt a file, and Quit. The function should be called displayMenu and should return an integer for the option selected. The menu should be re-displayed after each selection until the user enters “3” for Quit.
In main(), you will need to create an object of the Message class. You will use this object to call the functions in the Message class that will encrypt, decrypt, save, and print the words retrieved from the document.
Before you create this Message object, you should prompt the user to enter an encryption/decryption key. Once the user enters the key, pass this string in to the constructor of the Message class when creating your Message object.
Menu Options:
Option 1 – Encrypt file / Option 2- Decrypt file ( both same procedure)
When the user selects this option, they should be prompted to enter the name of the file to be encrypted: This file should already exist and contain a single paragraph of information. Make sure to include appropriate error checking to ensure that the file exists and can be opened. You may test with a file that contains something like this as its contents:
Our Father, which art in heaven, Hallowed be thy Name. Thy Kingdom come. Thy will be done in earth, As it is in heaven. Give us this day our daily bread. And forgive us our trespasses, As we forgive them that trespass against us. And lead us not into temptation, But deliver us from evil. For thine is the kingdom, The power, and the glory, For ever and ever. Amen.
Your program should open the file and read its contents into a character array using the getline function. As soon as the file’s contents have been read into the character array, main() should print the contents of the array to the screen.
Next, using the strtok_s function as described above, tokenize the entire message into separate words. As each word is tokenized, call the encryptWord function in the Message class which should receive the token as a char* data type and cast it into a string. It should then pass this string into the encrypt function in the Vigenere class using the Vigenere object that is a data member in the Message class. Once the word is encrypted, the encryptWord function in the Message class should push the encrypted word onto the vector of words in the Message class.
This process is repeated until the entire file’s contents have been tokenized and encrypted.
Once the entire file has been tokenized and its encrypted words stored, main() should call the function in the Message class to create a new file that contains the encrypted words. Therefore, the user must be prompted to enter the name of the file in which the encrypted data will be stored.
The program will then create a new file based on the name that the user entered containing the contents of the words vector in the Message class. main() should call the showWords function in the Message class to print the contents of the words vector and then call the makeFile function in the Message class to create the file and print the contents of the vector to the file.
![Vigenere Cypher
Main Menu
Enter an encryption/decryption key:
ThisStringCanBeAnything
1 - Encrypt File
2 - Decrypt File
3 - Quit
Menu Options:
Option 1- Encrypt file
When the user selects this option, he or she should immediately be prompted to enter the
name of the file to be encrypted: [Note that the file name can contain spaces.]
Selection:
Enter the name of the file to encrypt: The Lords Prayer.txt
unction. As soon as tne ile s contents nave been read into the cnaracter array, mman) should
eint the contents of the array to the screen.
A new file vill be created that contains the encrypted message
ur Father, which art in heaven, Hallowed be thy Name. Thy Kingdom come. Thy will b
done in earth, As it is in heaven. Give us this day our daily bread. And forgive
s our trespasses, As we forgive then that trespass against us. And lead us not int
temptation, But deliver us from evil. For thine is the kingdom, The power, and th
glory, For ever and ever. Amen.
Snip
Please enter the nane of the new file to create: The Lords Prayer (Encrypted).txt
The program will then create a new file besed on the name that the user entered, and that file will
contain the coatents of the wards vector in the Message class. Recall that this vector contains
the words that are sow encrypted. Therefore, main) should call the showWords function in the
Message chass to print the contents of the words vector to the screen and then call the makeFile
function in the Message class to create the filand print the contents of the vector to the file.
iext, using the strtok s function as described above, tokenize the entire message into separate
rde Ae each ned it mkenired eall the enewnelWard funetinn in the Meceson elace fneine O
e nen file containa the following encrypted ssage.
Z YHBZGR POQUO TYB BU ALINGN AHTOQARE UL HOG GHUM HOG DPVYFOZ WAM MOG PPTO UL
BU XHZL) T2 BA 82 BU ALINGN ZPOM NE NOOK WHG HBZ MHỌDA UYNSF TUL WZYKVR NZ HE
MKRAFTIS TZ PL WZYKVR VOME HOIL MRAT TNEAPSO NE TUL ELIV NZ OV BLBG ML
EN LBB MLTAXEE NZ YYME XCQO WZ MOOFG BZ HOH DPVYFOZ MOM IVENT TUL HON ZSWJA Wa
O TUL XOO TIVF
new file will be created that contains the decrypted message.
Please enter the name of the new file to create:
The Lords Prayer (Decrypted).txt
Enter the name of the file to decrypt: The Lords Prayer (Encrypted). txt
t The new file contains the following decrypted message
DUR FATHER WHICH ART IN HEAVEN HALLOWED BE THY NAME THY KINGDOM COME THY WILL BE DO
Vigurare Cypher
IN EARTH AS IT IS IN HEAVEN GIVE US THIS DAY OUR DAILY BREAD AND FORGIVE US OUR
Your program should open the file and read its contents into a character array using the gettine RESPASSES AS WE FORGIVE THEM THAT TRESPASS AGAINST US AND LEAD US NOT INTO TEMPTAT
function. As soon as the file's contents have been read into the character array, main() should ON BUT DELIVER US FROM EVIL FOR THINE IS THE KINGDOM THE POWER AND THE GLORY FORE
Main Menu
VER AND EVER AMEN
print the contents of the array to the screen.
1- Encrypt File
2- Decrypt File
3. Quit
vigenere Cypher
HBZ YHBZGR POQUI TYB BU ALINGN AHTDQURE UL MOG GHUN MOG DPVYFOZ WUN MOG PPTD UL W
W BU XHZLJ TZ BA BZ BU ALINGN ZPON NE HOOK WHG HBZ WHODA UYMSF TUL YWZYKVR NE HBZ
KRAFTIS TZ PL YVZYKVR MOME MOIL MYMKRAFT TNIAPSG NZ TUL ELIV NZ GVB BUBG MLUHVAG
JSN UBB MLTAXEE NZ YWE XCQO YVZ MOQFG BZ MOM DPVYFOZ MOM IVENT TUL MOM ZSKJA YWZ X
CO TUL XOO TTMF
Main Menu
1 - Encrypt File
2 - DeCrypt File
3 - Quit
Salaction:
Seles ts](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F917ba0ca-c311-4497-ab5e-23b52ffbd081%2Fff9f919a-9f3a-4b40-9187-53ca1fb607fd%2Fv33fot4_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

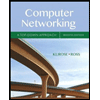
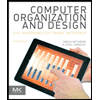
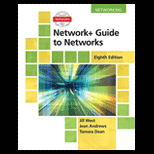
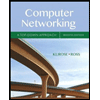
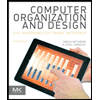
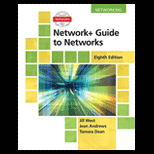
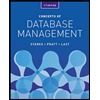
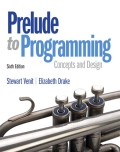
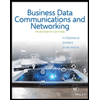