Write a program to manage a set of graphical objects based on the commands in a specified file. This is a graphical equivalent of the famous edlin tool for text manipulation. On the Vula page of the assignment, you will find VectorGraphics and Question2 classes that will handle the file I/O for you. When you run Question2, specify the file name like this: java Question2 myfile.in Each line of the file contains an instruction in one of the formats given below: a rectangle a hline a vline a ptline w d m x Explanation: ‘a’ adds an object of a particular type (rectangle/hline/vline/ptline) with the specified parameters. ‘w’ renders/draws the objects and prints the composite image to the screen. ‘d’ deletes an object based on its id. ‘m’ moves an object to a new position based on its id. ‘x’ exits the program. Note: • A 'ptline' differs from the other objects in that the values after the object type are not lengths, but instead a second point described by x1 and y1. This object represents a line from (x, y) to (x1, y1). • The answers the automatic marker expects were generated using an implementation of Bresenham's Line Drawing Algorithm. (See the appendix). • Assume that the drawing canvas is 20 blocks wide (in the x direction) and 20 blocks high in the y direction), starting with (0,0) in the top left. You are only required to create the Rectangle, HLine, VLine and PtLine subclasses of the given VectorObject base class. You should add comments to all classes you write. Sample Input File a 1 5 5 rectangle 10 10 a 2 3 3 hline 14 a 3 3 16 hline 14 a 4 3 3 vline 14 a 5 16 3 vline 14 w d 1 m 2 3 5 m 3 3 14 m 4 5 3 m 5 14 3 a 6 6 6 ptline 13 13 a 6 13 6 ptline 6 13 w x //programs given import java.util.*; import java.io.*; /** * Assignment 5 Q3 VectorGraphics 2011 version * * @version 1.1 */ class VectorGraphics { private static final String RENDER = "W"; private static final String EXIT = "X"; private static final String ADD = "A"; private static final String MOVE = "M"; private static final String RECTANGLE = "RECTANGLE"; private static final String HLINE = "HLINE"; private static final String VLINE = "VLINE"; private static final String PTLINE = "PTLINE"; private static final String DELETE = "D"; private int xSize, ySize; private VectorObject[] objects; private int numberOfObjects; VectorGraphics(int x, int y, int objSize) { this.xSize = x; this.ySize = y; this.objects = new VectorObject[objSize]; this.numberOfObjects = 0; } void run(String filename) { Scanner inputStream = null; try { inputStream = new Scanner(new FileInputStream(filename)); } catch (Exception e) { System.exit(0); } while (inputStream.hasNextLine()) { String action = inputStream.next().toUpperCase(); if (action.equals(EXIT)) { return; } else if (action.equals(RENDER)) { render(); } else if (action.equals(ADD)) { int id = inputStream.nextInt(); int x = inputStream.nextInt(); int y = inputStream.nextInt(); String obj = inputStream.next().toUpperCase(); if (obj.equals(RECTANGLE)) { int xLen = inputStream.nextInt(); int yLen = inputStream.nextInt(); add(new Rectangle(id, x, y, xLen, yLen)); } else if (obj.equals(HLINE)) { int len = inputStream.nextInt(); add(new HLine(id, x, y, len)); } else if (obj.equals(VLINE)) { int len = inputStream.nextInt(); add(new VLine(id, x, y, len)); } else if (obj.equals(PTLINE)) { int bx = inputStream.nextInt(); int by = inputStream.nextInt(); add(new PtLine(id, x, y, bx, by)); } } else if (action.equals(DELETE)) { int id = inputStream.nextInt(); delete(id); } else if (action.equals(MOVE)) { int id = inputStream.nextInt(); int x = inputStream.nextInt(); int y = inputStream.nextInt(); move(id, x, y); } } inputStream.close(); } private void add(VectorObject anObject) { objects[numberOfObjects++] = anObject; } private boolean delete(int aNumber) { for (int i = 0; i < numberOfObjects; i++) if (aNumber == objects[i].getId()) { for (int j = i; j < numberOfObjects - 1; j++) objects[j] = objects[j + 1]; numberOfObjects--; return true; } return false; } private void move(int aNumber, int x, int y) { for (int i = 0; i < numberOfObjects; i++) if (aNumber == objects[i].getId()) { objects[i].setNewCoords(x, y); return; } } private void render() { char[][] matrix = new char[ySize][xSize]; for (int y = 0; y < ySize; y++) { for (int x = 0; x < xSize; x++) { matrix[y][x] = ' '; } } for (int i = 0; i < numberOfObjects; i++) { objects[i].draw(matrix); } System.out.print('+'); for (int x = 0; x < xSize; x++) { System.out.print('-'); } System.out.println('+'); for (int y = 0; y < ySize; y++) { System.out.print('+'); for (int x = 0; x < xSize; x++) { System.out.print(matrix[y][x]); } System.out.println('+'); } System.out.print('+'); for (int x = 0; x < xSize; x++) { System.out.print('-'); } System.out.println('+'); } } abstract class VectorObject { protected int id, x, y; VectorObject ( int anId, int ax, int ay ) { id = anId; x = ax; y = ay; } int getId () { return id; } void setNewCoords ( int newx, int newy ) { x = newx; y = newy; } public abstract void draw ( char [][] matrix );
Write a program to manage a set of graphical objects based on the commands in a specified
file. This is a graphical equivalent of the famous edlin tool for text manipulation.
On the Vula page of the assignment, you will find VectorGraphics and Question2
classes that will handle the file I/O for you. When you run Question2, specify the file name
like this:
java Question2 myfile.in
Each line of the file contains an instruction in one of the formats given below:
a <id> <x> <y> rectangle <x_length> <y_length>
a <id> <x> <y> hline <x_length>
a <id> <x> <y> vline <y_length>
a <id> <x> <y> ptline <y1> <y2>
w
d <id>
m<id><x> <y>
x
Explanation:
‘a’ adds an object of a particular type (rectangle/hline/vline/ptline) with the specified
parameters.
‘w’ renders/draws the objects and prints the composite image to the screen.
‘d’ deletes an object based on its id.
‘m’ moves an object to a new position based on its id.
‘x’ exits the program.
Note:
• A 'ptline' differs from the other objects in that the values after the object type are not
lengths, but instead a second point described by x1 and y1. This object represents a
line from (x, y) to (x1, y1).
• The answers the automatic marker expects were generated using an implementation
of Bresenham's Line Drawing
• Assume that the drawing canvas is 20 blocks wide (in the x direction) and 20 blocks
high in the y direction), starting with (0,0) in the top left.
You are only required
to create the Rectangle, HLine, VLine and PtLine subclasses of the given VectorObject base
class. You should add comments to all classes you write.
Sample Input File
a 1 5 5 rectangle 10 10
a 2 3 3 hline 14
a 3 3 16 hline 14
a 4 3 3 vline 14
a 5 16 3 vline 14
w
d 1
m 2 3 5
m 3 3 14
m 4 5 3
m 5 14 3
a 6 6 6 ptline 13 13
a 6 13 6 ptline 6 13
w
x
//programs given
import java.util.*;
import java.io.*;
/**
* Assignment 5 Q3 VectorGraphics 2011 version
*
* @version 1.1
*/
class VectorGraphics {
private static final String RENDER = "W";
private static final String EXIT = "X";
private static final String ADD = "A";
private static final String MOVE = "M";
private static final String RECTANGLE = "RECTANGLE";
private static final String HLINE = "HLINE";
private static final String VLINE = "VLINE";
private static final String PTLINE = "PTLINE";
private static final String DELETE = "D";
private int xSize, ySize;
private VectorObject[] objects;
private int numberOfObjects;
VectorGraphics(int x, int y, int objSize) {
this.xSize = x;
this.ySize = y;
this.objects = new VectorObject[objSize];
this.numberOfObjects = 0;
}
void run(String filename) {
Scanner inputStream = null;
try {
inputStream = new Scanner(new FileInputStream(filename));
} catch (Exception e) {
System.exit(0);
}
while (inputStream.hasNextLine()) {
String action = inputStream.next().toUpperCase();
if (action.equals(EXIT)) {
return;
} else if (action.equals(RENDER)) {
render();
} else if (action.equals(ADD)) {
int id = inputStream.nextInt();
int x = inputStream.nextInt();
int y = inputStream.nextInt();
String obj = inputStream.next().toUpperCase();
if (obj.equals(RECTANGLE)) {
int xLen = inputStream.nextInt();
int yLen = inputStream.nextInt();
add(new Rectangle(id, x, y, xLen, yLen));
} else if (obj.equals(HLINE)) {
int len = inputStream.nextInt();
add(new HLine(id, x, y, len));
} else if (obj.equals(VLINE)) {
int len = inputStream.nextInt();
add(new VLine(id, x, y, len));
} else if (obj.equals(PTLINE)) {
int bx = inputStream.nextInt();
int by = inputStream.nextInt();
add(new PtLine(id, x, y, bx, by));
}
} else if (action.equals(DELETE)) {
int id = inputStream.nextInt();
delete(id);
} else if (action.equals(MOVE)) {
int id = inputStream.nextInt();
int x = inputStream.nextInt();
int y = inputStream.nextInt();
move(id, x, y);
}
}
inputStream.close();
}
private void add(VectorObject anObject) {
objects[numberOfObjects++] = anObject;
}
private boolean delete(int aNumber) {
for (int i = 0; i < numberOfObjects; i++)
if (aNumber == objects[i].getId()) {
for (int j = i; j < numberOfObjects - 1; j++)
objects[j] = objects[j + 1];
numberOfObjects--;
return true;
}
return false;
}
private void move(int aNumber, int x, int y) {
for (int i = 0; i < numberOfObjects; i++)
if (aNumber == objects[i].getId()) {
objects[i].setNewCoords(x, y);
return;
}
}
private void render() {
char[][] matrix = new char[ySize][xSize];
for (int y = 0; y < ySize; y++) {
for (int x = 0; x < xSize; x++) {
matrix[y][x] = ' ';
}
}
for (int i = 0; i < numberOfObjects; i++) {
objects[i].draw(matrix);
}
System.out.print('+');
for (int x = 0; x < xSize; x++) {
System.out.print('-');
}
System.out.println('+');
for (int y = 0; y < ySize; y++) {
System.out.print('+');
for (int x = 0; x < xSize; x++) {
System.out.print(matrix[y][x]);
}
System.out.println('+');
}
System.out.print('+');
for (int x = 0; x < xSize; x++) {
System.out.print('-');
}
System.out.println('+');
}
}
abstract class VectorObject
{
protected int id, x, y;
VectorObject ( int anId, int ax, int ay )
{
id = anId;
x = ax;
y = ay;
}
int getId ()
{
return id;
}
void setNewCoords ( int newx, int newy )
{
x = newx;
y = newy;
}
public abstract void draw ( char [][] matrix );
}
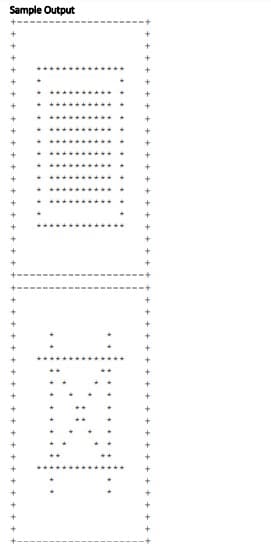
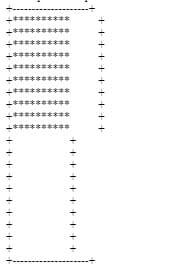

Step by step
Solved in 3 steps

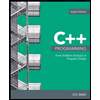
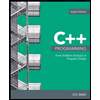