Write a Python program, in a file called number_guessing.py, to play a number guessing game with two players. The game is played for a fixed number of “sets” as pre-determined by the players. For each set, each player is given 2 tries to guess the value of a randomly generated number (between 1 and 6, inclusive). A different random number is used for each player, for each set. If the player guesses the number on the first try, they get 5 points (and don’t have to guess again), if they guess the number on the second try, they get 3 points, otherwise they get no points. The player with the highest number of points, at the end of all sets, wins the game (or there can be a tie/draw). Your program should include the following functions: • function main to do the following: o obtain as input, the number of sets that the players wish to play o obtain the name of the two players o for each set of the game, and for each player, randomly generate the number to be guessed o compute and return the points for a player, at a given set, using the function computePoints (see description below) o print the results of each set (see sample output below) o print the winner of the game (after all sets are played) using the function printWinner (see description below) Note: Study the given sample input/output to see what and how the input should be obtained and how the output should be generated. • function computePoints which, given the player name and the random number for a player, asks the player for their guesses, and computes and returns the number of points obtained (as described above) • function printWinner which, given the total points and names of each player, prints the results (winner's name or "tie/draw" message). Sample input/output could be as follows (input in bold blue): Please enter the number of sets you wish to play: 3 Please enter first player's name: Bob Please enter second player's name: Lee Bob, enter your first guess: 6 Bob, enter your second guess: 3 Lee, enter your first guess: 6 Lee, enter your second guess: 2 For SET 1: Bob has earned 0 points. The random number was: 2 Lee has earned 0 points. The random number was: 4 Bob, enter your first guess: 8 Bob, enter your second guess: 2 Lee, enter your first guess: 5 Lee, enter your second guess: 1 For SET 2: Bob has earned 0 points. The random number was: 5 Lee has earned 0 points. The random number was: 3 Bob, enter your first guess: 4 Lee, enter your first guess: 3 Lee, enter your second guess: 6 For SET 3: Bob has earned 5 points. The random number was: 4 Lee has earned 0 points. The random number was: 1 Bob is the winner of the game.
Write a Python program, in a file called number_guessing.py, to play a number guessing game with two players. The game is played for a fixed number of “sets” as pre-determined by the players. For each set, each player is given 2 tries to guess the value of a randomly generated number (between 1 and 6, inclusive). A different random number is used for each player, for each set. If the player guesses the number on the first try, they get 5 points (and don’t have to guess again), if they guess the number on the second try, they get 3 points, otherwise they get no points. The player with the highest number of points, at the end of all sets, wins the game (or there can be a tie/draw). Your program should include the following functions: • function main to do the following: o obtain as input, the number of sets that the players wish to play o obtain the name of the two players o for each set of the game, and for each player, randomly generate the number to be guessed o compute and return the points for a player, at a given set, using the function computePoints (see description below) o print the results of each set (see sample output below) o print the winner of the game (after all sets are played) using the function printWinner (see description below) Note: Study the given sample input/output to see what and how the input should be obtained and how the output should be generated. • function computePoints which, given the player name and the random number for a player, asks the player for their guesses, and computes and returns the number of points obtained (as described above) • function printWinner which, given the total points and names of each player, prints the results (winner's name or "tie/draw" message). Sample input/output could be as follows (input in bold blue): Please enter the number of sets you wish to play: 3 Please enter first player's name: Bob Please enter second player's name: Lee Bob, enter your first guess: 6 Bob, enter your second guess: 3 Lee, enter your first guess: 6 Lee, enter your second guess: 2 For SET 1: Bob has earned 0 points. The random number was: 2 Lee has earned 0 points. The random number was: 4 Bob, enter your first guess: 8 Bob, enter your second guess: 2 Lee, enter your first guess: 5 Lee, enter your second guess: 1 For SET 2: Bob has earned 0 points. The random number was: 5 Lee has earned 0 points. The random number was: 3 Bob, enter your first guess: 4 Lee, enter your first guess: 3 Lee, enter your second guess: 6 For SET 3: Bob has earned 5 points. The random number was: 4 Lee has earned 0 points. The random number was: 1 Bob is the winner of the game.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter5: Control Structures Ii (repetition)
Section: Chapter Questions
Problem 14PE
Related questions
Question
Write a Python program, in a file called number_guessing.py, to play a number guessing game with two
players.
The game is played for a fixed number of “sets” as pre-determined by the players. For each set, each player is
given 2 tries to guess the value of a randomly generated number (between 1 and 6, inclusive). A different
random number is used for each player, for each set. If the player guesses the number on the first try, they get
5 points (and don’t have to guess again), if they guess the number on the second try, they get 3 points,
otherwise they get no points. The player with the highest number of points, at the end of all sets, wins the
game (or there can be a tie/draw).
Your program should include the following functions:
• function main to do the following:
o obtain as input, the number of sets that the players wish to play
o obtain the name of the two players
o for each set of the game, and for each player, randomly generate the number to be guessed
o compute and return the points for a player, at a given set, using the function computePoints (see
description below)
o print the results of each set (see sample output below)
o print the winner of the game (after all sets are played) using the function printWinner (see
description below)
Note: Study the given sample input/output to see what and how the input should be obtained and
how the output should be generated.
• function computePoints which, given the player name and the random number for a player, asks the
player for their guesses, and computes and returns the number of points obtained (as described above)
• function printWinner which, given the total points and names of each player, prints the results
(winner's name or "tie/draw" message).
Sample input/output could be as follows (input in bold blue):
Please enter the number of sets you wish to play: 3
Please enter first player's name: Bob
Please enter second player's name: Lee
Bob, enter your first guess: 6
Bob, enter your second guess: 3
Lee, enter your first guess: 6
Lee, enter your second guess: 2
For SET 1:
Bob has earned 0 points. The random number was: 2
Lee has earned 0 points. The random number was: 4
Bob, enter your first guess: 8
Bob, enter your second guess: 2
Lee, enter your first guess: 5
Lee, enter your second guess: 1
For SET 2:
Bob has earned 0 points. The random number was: 5
Lee has earned 0 points. The random number was: 3
players.
The game is played for a fixed number of “sets” as pre-determined by the players. For each set, each player is
given 2 tries to guess the value of a randomly generated number (between 1 and 6, inclusive). A different
random number is used for each player, for each set. If the player guesses the number on the first try, they get
5 points (and don’t have to guess again), if they guess the number on the second try, they get 3 points,
otherwise they get no points. The player with the highest number of points, at the end of all sets, wins the
game (or there can be a tie/draw).
Your program should include the following functions:
• function main to do the following:
o obtain as input, the number of sets that the players wish to play
o obtain the name of the two players
o for each set of the game, and for each player, randomly generate the number to be guessed
o compute and return the points for a player, at a given set, using the function computePoints (see
description below)
o print the results of each set (see sample output below)
o print the winner of the game (after all sets are played) using the function printWinner (see
description below)
Note: Study the given sample input/output to see what and how the input should be obtained and
how the output should be generated.
• function computePoints which, given the player name and the random number for a player, asks the
player for their guesses, and computes and returns the number of points obtained (as described above)
• function printWinner which, given the total points and names of each player, prints the results
(winner's name or "tie/draw" message).
Sample input/output could be as follows (input in bold blue):
Please enter the number of sets you wish to play: 3
Please enter first player's name: Bob
Please enter second player's name: Lee
Bob, enter your first guess: 6
Bob, enter your second guess: 3
Lee, enter your first guess: 6
Lee, enter your second guess: 2
For SET 1:
Bob has earned 0 points. The random number was: 2
Lee has earned 0 points. The random number was: 4
Bob, enter your first guess: 8
Bob, enter your second guess: 2
Lee, enter your first guess: 5
Lee, enter your second guess: 1
For SET 2:
Bob has earned 0 points. The random number was: 5
Lee has earned 0 points. The random number was: 3
Bob, enter your first guess: 4
Lee, enter your first guess: 3
Lee, enter your second guess: 6
For SET 3:
Bob has earned 5 points. The random number was: 4
Lee has earned 0 points. The random number was: 1
Bob is the winner of the game.
Lee, enter your first guess: 3
Lee, enter your second guess: 6
For SET 3:
Bob has earned 5 points. The random number was: 4
Lee has earned 0 points. The random number was: 1
Bob is the winner of the game.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
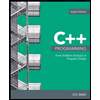
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
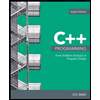
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning